C In Terms Of Mu And Epsilon
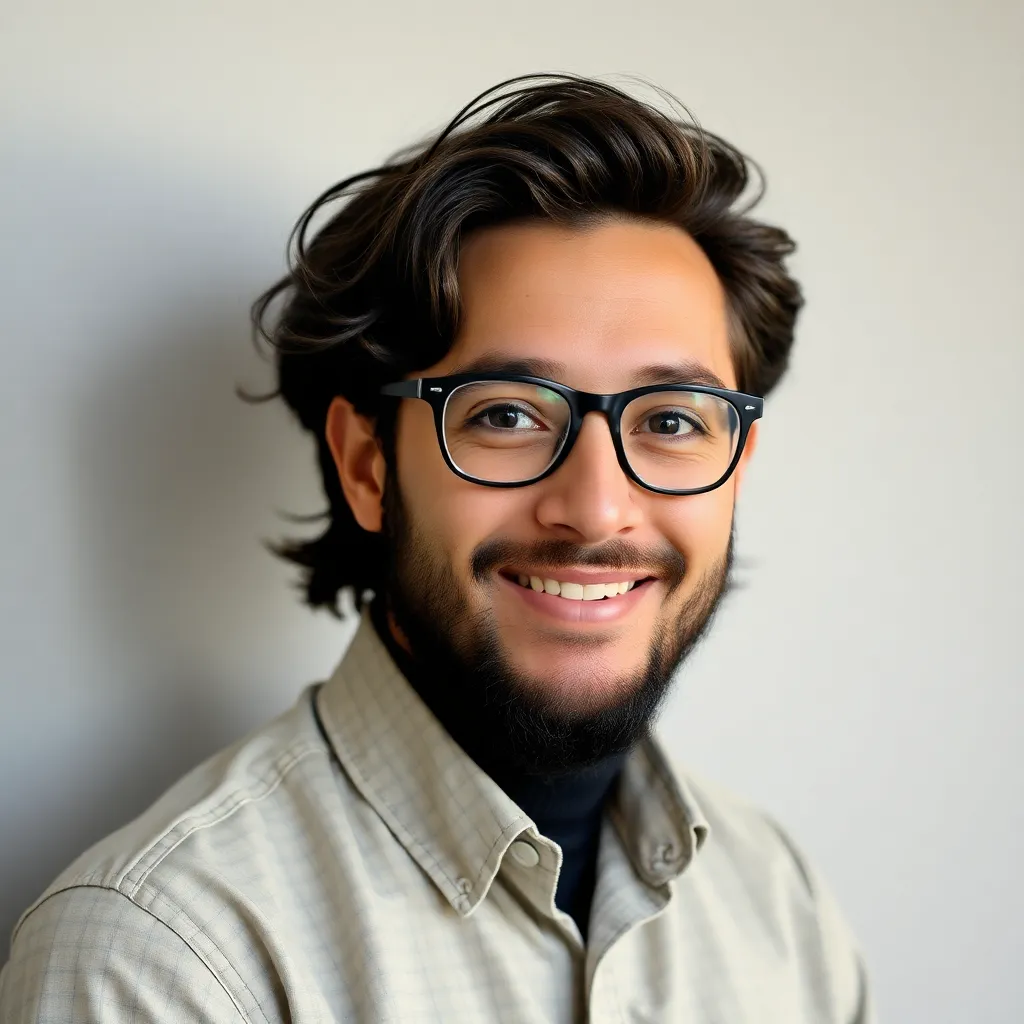
Juapaving
Apr 02, 2025 · 6 min read
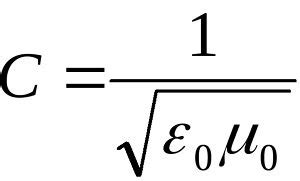
Table of Contents
C in Terms of Mu and Epsilon: A Deep Dive into Numerical Precision and Error Handling
The seemingly simple C programming language, while powerful and versatile, presents challenges when dealing with floating-point arithmetic. Understanding the nuances of floating-point representation, particularly the concepts of mu (µ) and epsilon (ε), is crucial for writing robust and accurate C programs, especially those involved in scientific computing, financial modeling, or any application requiring high precision. This article will delve deep into the implications of mu and epsilon within the context of C, exploring their significance, practical applications, and strategies for mitigating the associated errors.
Understanding Floating-Point Representation in C
Before diving into mu and epsilon, let's briefly review how floating-point numbers are represented in C. C uses the IEEE 754 standard for floating-point arithmetic, which represents numbers in a format similar to scientific notation:
(-1)^sign * mantissa * 2^exponent
Where:
- sign: Indicates whether the number is positive or negative (0 for positive, 1 for negative).
- mantissa (or significand): The fractional part of the number. It's usually normalized to be between 1 (inclusive) and 2 (exclusive).
- exponent: Scales the mantissa by a power of 2.
The precision of a floating-point number depends on the number of bits allocated to the mantissa. Common floating-point types in C include float
(single-precision, usually 32 bits), double
(double-precision, usually 64 bits), and long double
(extended precision, size varies depending on the architecture). The limited number of bits allocated to represent the mantissa means that not all real numbers can be represented exactly. This inherent limitation is the root cause of many floating-point errors.
Mu (µ): The Unit of Least Precision
Mu (µ), often referred to as the unit in the last place (ULP), represents the smallest amount by which a floating-point number can change. It's essentially the distance between two adjacent floating-point numbers. The value of mu depends on the magnitude of the floating-point number itself. For example, mu will be smaller for numbers close to zero and larger for numbers with larger magnitudes.
Calculating mu precisely requires understanding the specific floating-point format used (e.g., single-precision or double-precision) and the number's exponent. However, understanding its conceptual meaning is more important than calculating its exact value in most situations.
Practical Implications of Mu:
- Rounding Errors: Understanding mu helps us comprehend the inherent limitations of floating-point representation. Many operations result in rounding errors where the exact result cannot be represented, and the closest representable number is used instead. The magnitude of this rounding error is directly related to mu.
- Comparison of Floating-Point Numbers: Direct equality comparisons (
==
) between floating-point numbers are often unreliable due to rounding errors. Instead, it's safer to check if two numbers are within a certain tolerance (a multiple of mu) of each other. - Algorithm Stability: In numerical algorithms, the accumulation of rounding errors over many iterations can significantly affect the accuracy of the final result. A deep understanding of mu helps in designing numerically stable algorithms that minimize error propagation.
Epsilon (ε): Machine Epsilon
Epsilon (ε), also known as machine epsilon, is a related but distinct concept. It represents the smallest positive number that, when added to 1.0, produces a result that is greater than 1.0. In other words, it's the smallest number that can cause a noticeable change when added to 1. Epsilon is a constant for a given floating-point format (e.g., float
or double
). It reflects the relative precision of the floating-point representation.
Practical Implications of Epsilon:
- Tolerance in Comparisons: Epsilon often serves as a useful threshold in floating-point comparisons. Instead of checking for strict equality, one can check if the difference between two numbers is less than a multiple of epsilon. This approach handles rounding errors more effectively.
- Error Analysis: Epsilon helps in analyzing the magnitude of relative errors in calculations. By comparing the error to epsilon, you can assess whether the error is significant or simply due to the inherent limitations of floating-point arithmetic.
- Algorithm Design: Awareness of epsilon is crucial in designing algorithms that are robust to floating-point errors. For instance, algorithms that involve iterative refinement may need to incorporate epsilon-based stopping criteria to prevent unnecessary iterations.
Illustrative C Code: Exploring Mu and Epsilon
While directly calculating mu for an arbitrary floating-point number can be complex, we can get a reasonable approximation using the following C code snippet:
#include
#include
int main() {
double x = 1.0;
double mu_approx;
// Approximate mu for a specific number x
do {
mu_approx = x;
x /= 2.0;
} while (1.0 + x > 1.0);
printf("Approximation of mu (for x=1.0): %e\n", mu_approx);
// Obtaining machine epsilon (DBL_EPSILON for double)
printf("Machine Epsilon (DBL_EPSILON): %e\n", DBL_EPSILON);
return 0;
}
This code provides an approximation of mu for x = 1.0
and directly uses DBL_EPSILON
from <float.h>
to get the machine epsilon for double-precision floating-point numbers. Remember that the mu approximation is specific to the value of x
. For different values of x
, the mu approximation will change.
Strategies for Handling Floating-Point Errors in C
Several techniques help mitigate the impact of floating-point errors in C programs:
1. Using Higher Precision:
If precision is critical, consider using double
instead of float
or even long double
if your system supports it. Higher precision means smaller values of mu and epsilon, reducing the impact of rounding errors.
2. Careful Comparisons:
Avoid direct equality comparisons (==
) of floating-point numbers. Instead, use a tolerance-based comparison:
#include
// ... other code ...
if (fabs(a - b) < 1e-10) { // Use a suitable tolerance
// Consider a and b as equal
}
The tolerance should be chosen based on the expected magnitude of the numbers and the desired level of accuracy. Epsilon can guide the choice of tolerance.
3. Accumulating Sums Carefully:
When summing a large number of floating-point numbers, the order of summation can affect the accuracy of the result. Consider using techniques like compensated summation or Kahan summation to minimize the accumulation of rounding errors.
4. Using Decimal Data Types (for specific scenarios):
While C doesn't have built-in decimal floating-point types, libraries exist (e.g., some arbitrary-precision arithmetic libraries) that offer higher precision using decimal representations. These are valuable when exact decimal representation is crucial (e.g., financial applications).
5. Implementing Robust Numerical Algorithms:
Choose numerically stable algorithms for your calculations. Some algorithms are inherently less susceptible to error propagation than others. Research and choose algorithms known for their robustness in floating-point arithmetic.
Conclusion
Mu and epsilon are fundamental concepts in understanding floating-point arithmetic within the context of C programming. By acknowledging the inherent limitations of floating-point representation and employing the strategies outlined above, developers can create more robust and accurate C programs, especially those working with numerical computations demanding high precision. A thorough grasp of mu and epsilon is essential for developing reliable and trustworthy software in numerous applications where numerical accuracy is paramount. Remember that understanding the limitations of floating-point numbers is the first step towards writing code that handles them effectively. The choice of data types, algorithms, and comparison methods should always reflect the desired precision and accuracy requirements of your application.
Latest Posts
Latest Posts
-
How Are Prokaryotes And Eukaryotes Alike
Apr 03, 2025
-
Which Of The Following Statements Is Always True
Apr 03, 2025
-
Balanced Chemical Equation For Magnesium Oxide
Apr 03, 2025
-
What Is The Group Of Birds Called
Apr 03, 2025
-
Common Factors Of 28 And 42
Apr 03, 2025
Related Post
Thank you for visiting our website which covers about C In Terms Of Mu And Epsilon . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.