Array Of Pointers In C Language
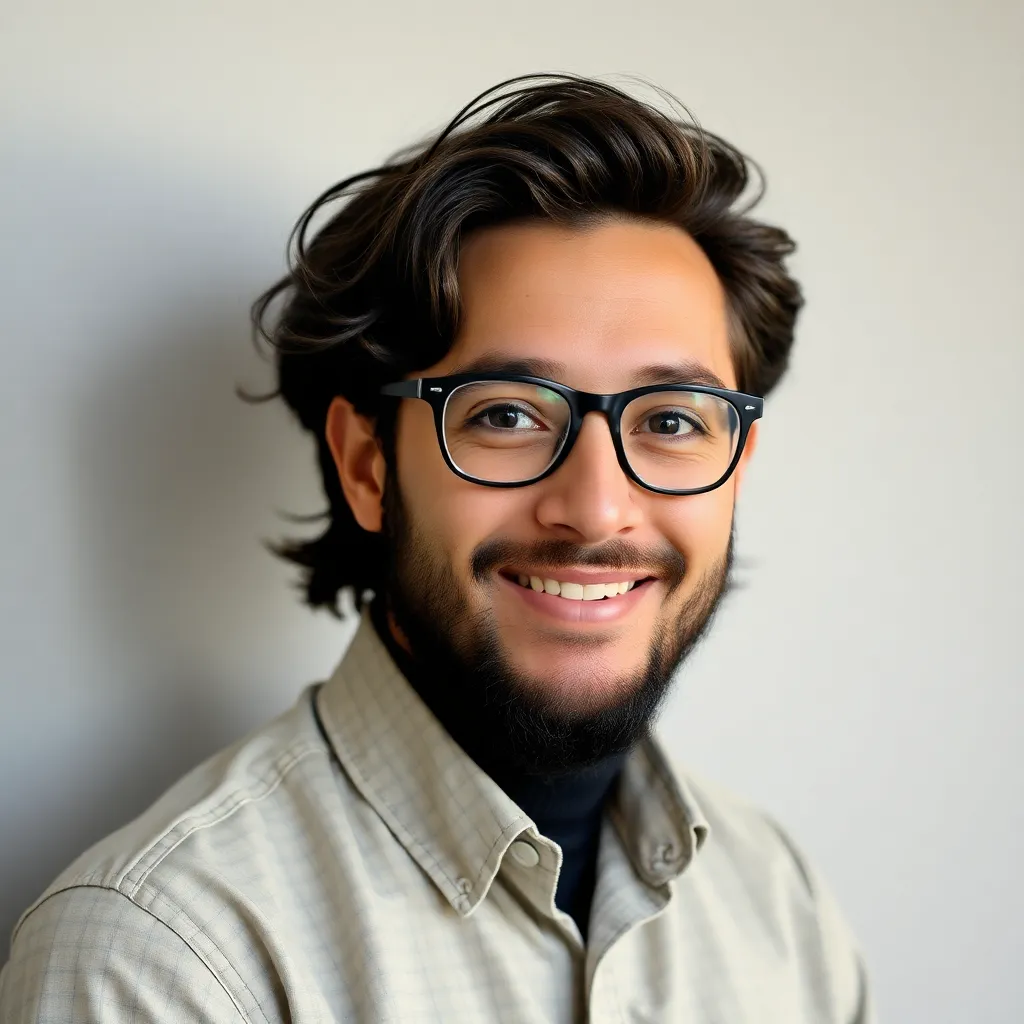
Juapaving
Apr 01, 2025 · 7 min read
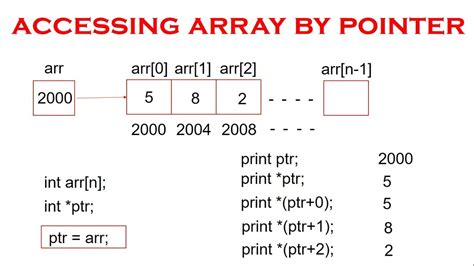
Table of Contents
Arrays of Pointers in C: A Deep Dive
Arrays of pointers in C are a powerful yet often misunderstood feature. They allow for dynamic memory allocation, manipulation of multiple data structures, and efficient handling of large datasets. Mastering arrays of pointers is crucial for any serious C programmer. This comprehensive guide will delve into the intricacies of arrays of pointers, exploring their functionalities, benefits, and practical applications with illustrative examples.
Understanding Pointers in C
Before diving into arrays of pointers, let's solidify our understanding of pointers themselves. A pointer is a variable that holds the memory address of another variable. We declare a pointer using the asterisk (*) symbol before the variable name.
int x = 10;
int *ptr; // Declares a pointer named 'ptr' that can hold the address of an integer variable.
ptr = &x; // Assigns the address of x to ptr.
&x
is the address-of operator, yielding the memory location of x
. *ptr
(the dereference operator) accesses the value at the memory address stored in ptr
.
Declaring and Initializing Arrays of Pointers
An array of pointers is simply an array where each element is a pointer. The type of the pointer determines the data type the pointers can address.
int *ptrArray[5]; // Array of 5 integer pointers
char *charPtrArray[10]; // Array of 10 character pointers
float *floatPtrArray[20]; //Array of 20 float pointers
These declarations only create an array of pointers; they don't allocate memory for the data they will point to. Each element of ptrArray
is initially uninitialized, meaning it holds a garbage value (a random memory address). Attempting to dereference an uninitialized pointer leads to undefined behavior and potential program crashes.
Initializing Arrays of Pointers
There are several ways to initialize arrays of pointers:
1. Assigning addresses of existing variables:
int a = 10, b = 20, c = 30;
int *ptrArray[3] = {&a, &b, &c};
This directly assigns the addresses of a
, b
, and c
to the elements of ptrArray
.
2. Dynamic Memory Allocation:
This approach uses functions like malloc
or calloc
to allocate memory dynamically.
int *ptrArray[5];
for (int i = 0; i < 5; i++) {
ptrArray[i] = (int *)malloc(sizeof(int));
if (ptrArray[i] == NULL) {
// Handle memory allocation failure
printf("Memory allocation failed!\n");
//Consider appropriate error handling, such as exiting the program or freeing already allocated memory.
exit(1);
}
*ptrArray[i] = i * 10; // Assign values to the dynamically allocated memory
}
This code allocates memory for five integers and assigns each a different value. Crucially, it checks if malloc
was successful, handling potential errors. Always check the return value of malloc
! Failure to do so can lead to segmentation faults.
3. Initializing with NULL
:
Initializing pointers to NULL
indicates that they don't currently point to any valid memory location. This is a good practice for avoiding undefined behavior.
int *ptrArray[5] = {NULL, NULL, NULL, NULL, NULL};
Using Arrays of Pointers: Practical Applications
Arrays of pointers unlock a range of possibilities:
1. Arrays of Strings:
One of the most common uses is representing arrays of strings. Strings in C are null-terminated character arrays.
char *stringArray[3] = {"Hello", "World", "C Programming"};
for (int i = 0; i < 3; i++) {
printf("%s\n", stringArray[i]);
}
Here, stringArray
holds the addresses of three string literals.
2. Arrays of Functions:
This is a less common but powerful technique. You can create an array of function pointers, allowing you to call different functions based on some criteria.
// Function prototypes
int add(int a, int b);
int subtract(int a, int b);
int (*funcPtrArray[2])(int, int) = {add, subtract}; // Array of function pointers
int main() {
int result = funcPtrArray; // Calls add(5,3)
printf("Addition: %d\n", result);
result = funcPtrArray; // Calls subtract(5,3)
printf("Subtraction: %d\n", result);
return 0;
}
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
This showcases an array holding pointers to two functions (add
and subtract
).
3. Dynamically Resizable Arrays:
Arrays of pointers are often used to create dynamically resizable arrays. This is far more efficient than using a standard array which has a fixed size at compile time. We can allocate more space as needed, avoiding the limitations of fixed-size arrays.
#include
#include
int main() {
int **dynamicArray = NULL; // Double pointer for dynamic array of integers
int size = 5;
int capacity = 5; // Initial capacity
int i;
// Allocate initial memory block
dynamicArray = (int **)malloc(capacity * sizeof(int*));
if (dynamicArray == NULL){
fprintf(stderr,"Memory allocation failed!\n");
return 1;
}
for (i = 0; i < size; i++) {
dynamicArray[i] = (int *)malloc(sizeof(int));
if(dynamicArray[i] == NULL){
fprintf(stderr,"Memory allocation failed!\n");
//Free already allocated memory here.
for(int j = 0; j < i; j++) free(dynamicArray[j]);
free(dynamicArray);
return 1;
}
*dynamicArray[i] = i + 1;
}
// Print values
printf("Initial array:\n");
for (i = 0; i < size; i++) {
printf("%d ", *dynamicArray[i]);
}
printf("\n");
// Resize the array (example)
size += 2;
if(size > capacity){
capacity *=2; //Double the capacity
dynamicArray = (int **)realloc(dynamicArray, capacity * sizeof(int*));
if(dynamicArray == NULL){
fprintf(stderr,"Memory reallocation failed!\n");
//Free already allocated memory here.
for(int j = 0; j < i; j++) free(dynamicArray[j]);
free(dynamicArray);
return 1;
}
for(i = 5; i < size; i++){
dynamicArray[i] = (int *)malloc(sizeof(int));
if(dynamicArray[i] == NULL){
fprintf(stderr,"Memory allocation failed!\n");
//Free already allocated memory here.
for(int j = 0; j <= i; j++) free(dynamicArray[j]);
free(dynamicArray);
return 1;
}
*dynamicArray[i] = i + 1;
}
}
// Print values after resizing
printf("Resized array:\n");
for (i = 0; i < size; i++) {
printf("%d ", *dynamicArray[i]);
}
printf("\n");
//Free allocated memory
for(int i = 0; i < size; i++) free(dynamicArray[i]);
free(dynamicArray);
return 0;
}
This example demonstrates a dynamically resizable array. realloc
is used to extend the array's capacity when needed. Remember to always free the dynamically allocated memory to prevent memory leaks. Error handling is crucial in this scenario.
4. Representing 2D Arrays:
While C doesn't directly support true 2D arrays in the same way some languages do, an array of pointers can effectively mimic this functionality.
int *matrix[3]; // Array of 3 integer pointers
for (int i = 0; i < 3; i++) {
matrix[i] = (int *)malloc(4 * sizeof(int)); // Each row has 4 elements. Error handling omitted for brevity.
for (int j = 0; j < 4; j++) {
matrix[i][j] = i * 4 + j;
}
}
// Access elements: matrix[i][j]
// Free the memory. Crucial to avoid memory leaks.
for (int i = 0; i < 3; i++) {
free(matrix[i]);
}
This simulates a 3x4 matrix. Each element of matrix
points to a dynamically allocated row. Error handling is critical (omitted here for brevity). Always free the allocated memory to prevent leaks.
Important Considerations and Best Practices
- Error Handling: Always check the return values of
malloc
andrealloc
. Handle memory allocation failures gracefully to prevent crashes. - Memory Leaks: Always free dynamically allocated memory when it's no longer needed using
free()
. Failing to do this leads to memory leaks, which can severely impact your program's performance and stability. - Pointer Arithmetic: Be cautious when using pointer arithmetic with arrays of pointers. Ensure your calculations are correct to avoid accessing invalid memory locations.
- NULL Checks: Before dereferencing a pointer, always check if it's
NULL
. Dereferencing aNULL
pointer results in a segmentation fault. - Code Readability: Use meaningful variable names and add comments to explain complex logic. This improves maintainability and understanding.
Conclusion
Arrays of pointers are a fundamental concept in C programming, enabling powerful features like dynamic memory management, manipulation of strings and functions, and the creation of flexible data structures. Understanding their nuances, alongside careful memory management and robust error handling, is crucial for writing efficient and reliable C programs. By mastering arrays of pointers, you significantly enhance your ability to create sophisticated and versatile applications in C. Remember to consistently apply best practices, such as thorough error checking and diligent memory management, to ensure the robustness and stability of your code. This detailed exploration serves as a robust foundation for building more complex and efficient programs in C.
Latest Posts
Latest Posts
-
Unit Of Heat That Measures In The Energy In Food
Apr 02, 2025
-
How Are Sedimentary Rocks Formed Short Answer
Apr 02, 2025
-
Difference Between Atom And An Ion
Apr 02, 2025
-
2 Variances F Hypothesis Test Calculator
Apr 02, 2025
-
What Monument In India Is Made Of Metamorphic Rock
Apr 02, 2025
Related Post
Thank you for visiting our website which covers about Array Of Pointers In C Language . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.