What Is A Static Variable In C
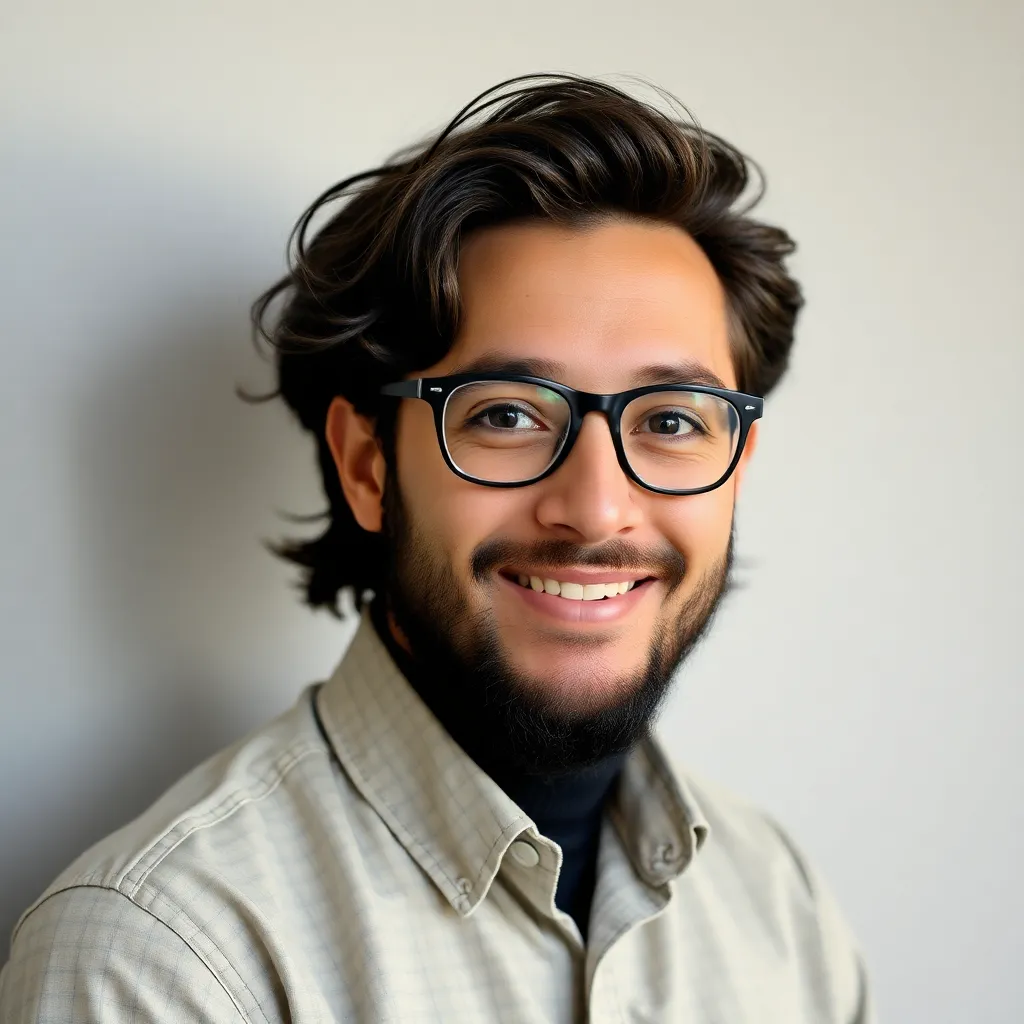
Juapaving
Mar 22, 2025 · 6 min read
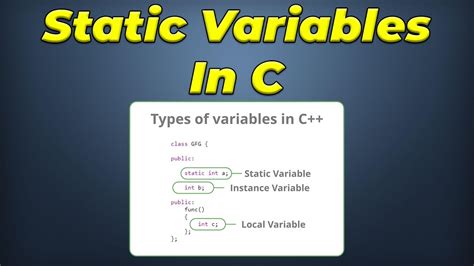
Table of Contents
What is a Static Variable in C? A Deep Dive
Understanding static variables in C is crucial for writing efficient and robust programs. They offer a powerful mechanism for managing data persistence and scope, influencing how your code behaves and interacts with memory. This comprehensive guide will delve into the intricacies of static variables, exploring their characteristics, usage, and implications for your C programming projects. We'll cover everything from the basics to advanced scenarios, equipping you with the knowledge to harness the power of static variables effectively.
Understanding Variable Scope and Lifetime
Before diving into static variables, let's refresh our understanding of variable scope and lifetime. These two concepts are fundamentally intertwined with how static variables function.
Scope: Where a Variable is Accessible
The scope of a variable defines the region of your code where that variable can be accessed and used. There are primarily two types of scope in C:
-
Local Scope: Variables declared within a function or block of code have local scope. They are only accessible within that specific function or block. Once the function or block completes execution, the local variables are destroyed and their memory is released.
-
Global Scope: Variables declared outside any function have global scope. They are accessible from any part of your program, after their declaration.
Lifetime: How Long a Variable Exists
The lifetime of a variable refers to the period during which the variable exists in memory. This is directly related to its scope and when it's created and destroyed. Generally, variables have a lifetime tied to their scope:
-
Automatic Storage Duration: Local variables typically have automatic storage duration. They are created when the function or block is entered and destroyed when it exits.
-
Static Storage Duration: Global variables and variables declared as
static
have static storage duration. They are created when the program begins execution and remain in memory until the program terminates.
The Power of the static
Keyword
The static
keyword in C significantly alters the behavior of variables. When applied to a variable, it changes both its scope and lifetime, as follows:
Static Local Variables: Persistence Across Function Calls
A static
local variable within a function maintains its value between function calls. Unlike regular local variables, which are re-initialized each time the function is invoked, a static local variable retains its value from the previous call.
#include
void myFunction() {
static int count = 0; // Static local variable
count++;
printf("Count: %d\n", count);
}
int main() {
myFunction(); // Output: Count: 1
myFunction(); // Output: Count: 2
myFunction(); // Output: Count: 3
return 0;
}
In this example, count
is initialized to 0 only once. Each subsequent call to myFunction()
increments count
from its previously stored value. This is a key advantage of using static local variables for maintaining state within a function.
Static Global Variables: Limiting Scope
A static
global variable, declared outside any function but within a source file, has file scope. This means it's only accessible within the same source file where it's defined, unlike regular global variables, which are accessible from any part of the program.
// file1.c
static int globalVar = 10; // Static global variable, file scope
void printGlobalVar() {
printf("Global Var: %d\n", globalVar);
}
// file2.c
// Cannot access globalVar from here!
This limitation of scope prevents naming conflicts between variables in different parts of your program. It enhances code modularity and makes it easier to manage larger projects.
Advanced Uses of Static Variables
Static variables extend beyond basic scope and lifetime manipulation. They play a crucial role in several advanced programming techniques:
Implementing Singleton Patterns
The singleton pattern, a design pattern ensuring only one instance of a class (or structure, in C) exists, can be elegantly implemented using static variables.
#include
#include
typedef struct {
int data;
} MySingleton;
static MySingleton* singleton = NULL;
MySingleton* getSingleton() {
if (singleton == NULL) {
singleton = (MySingleton*)malloc(sizeof(MySingleton));
singleton->data = 100;
}
return singleton;
}
int main() {
MySingleton* instance1 = getSingleton();
MySingleton* instance2 = getSingleton();
printf("Instance 1 data: %d\n", instance1->data); // Output: 100
printf("Instance 2 data: %d\n", instance2->data); // Output: 100
//Both point to same memory location, ensuring only one instance.
free(singleton); //Important: Free the memory after use.
return 0;
}
Static Functions: Encapsulation and Code Organization
A static
function, declared within a source file, is only accessible from within that same file. This enhances encapsulation, limiting external access to internal helper functions, and improves code organization.
// file1.c
static void helperFunction() {
// Internal helper function
}
void myFunction() {
helperFunction(); // Allowed
}
// file2.c
// Cannot call helperFunction() from here!
This restricted visibility prevents unintended modifications or misuse of these internal functions.
Static Arrays: Data Persistence Across Function Calls
Similar to static local variables, static arrays maintain their values between function calls. This enables functions to accumulate or store data over multiple invocations.
#include
void addToStaticArray(int value){
static int arr[5] = {0};
static int index = 0;
if(index < 5){
arr[index++] = value;
} else {
printf("Array is full\n");
}
}
int main() {
addToStaticArray(10);
addToStaticArray(20);
addToStaticArray(30);
//Access the array elements (for demonstration):
for (int i = 0; i < 3; i++){
printf("Element %d: %d\n", i+1, arr[i]);
}
return 0;
}
This differs from regular arrays which lose their contents after each function call.
Potential Pitfalls and Best Practices
While static variables offer significant advantages, it's essential to be aware of potential drawbacks and adopt best practices for their use.
Initialization Order: A Source of Subtle Bugs
The initialization order of static variables across multiple translation units (.c
files) is undefined. This means you cannot rely on a specific sequence when using multiple static variables across different files. Always strive for initialization within functions rather than relying on global initialization order.
Global State and Debugging: Increased Complexity
Excessive use of static global variables can introduce hidden dependencies and make debugging considerably more challenging. Over-reliance on global state can make your code harder to understand, test, and maintain.
Memory Management: Potential for Leaks (with dynamic memory allocation)
When using dynamic memory allocation with static variables (as in the singleton example), ensure proper deallocation (free()
) to prevent memory leaks. Failure to release allocated memory will lead to a gradual increase in memory consumption, potentially causing program crashes.
Conclusion: Mastering Static Variables in C
Static variables in C provide a powerful mechanism for controlling variable scope and lifetime, offering numerous advantages for efficient code development. However, they introduce complexities that demand careful consideration and adherence to best practices. By understanding their capabilities, limitations, and potential pitfalls, you can leverage static variables effectively to improve code organization, enhance modularity, and implement sophisticated programming patterns. Remember to prioritize code clarity, maintainability, and the avoidance of undefined behavior when working with static variables in your C projects. Always aim for a balance between the advantages of static variables and the potential challenges they present, choosing them judiciously to enhance the quality and robustness of your programs.
Latest Posts
Latest Posts
-
Does A Cube Have Equal Sides
Mar 23, 2025
-
Melting Of Ice Is A Physical Change
Mar 23, 2025
-
Why Is Ethanol Soluble In Water
Mar 23, 2025
-
8 Vertices 12 Edges 6 Faces
Mar 23, 2025
-
Can Photosynthesis Occur In The Dark
Mar 23, 2025
Related Post
Thank you for visiting our website which covers about What Is A Static Variable In C . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.