Uses Numbers Variables And Operation Symbol
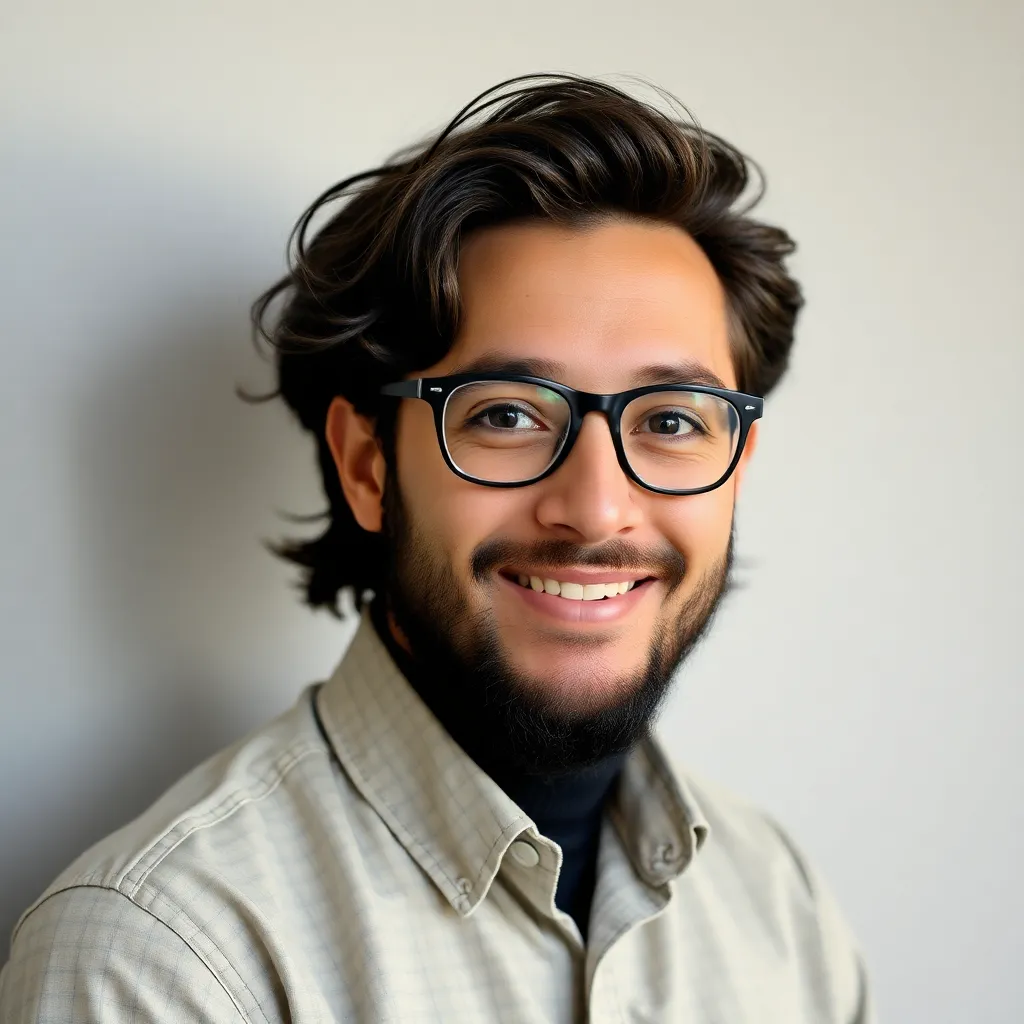
Juapaving
Mar 14, 2025 · 6 min read
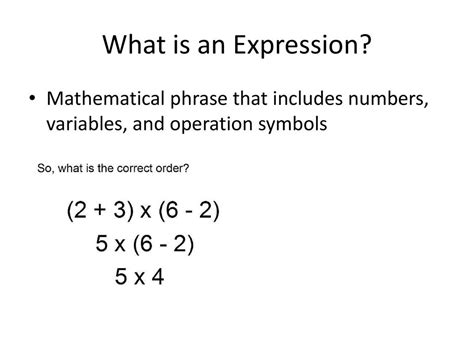
Table of Contents
Mastering the Building Blocks of Programming: Numbers, Variables, and Operators
Understanding numbers, variables, and operators is fundamental to any programming language. These core concepts form the bedrock upon which all other programming constructs are built. This comprehensive guide will explore each element in detail, providing practical examples and explaining their importance in building robust and efficient code. We'll delve into the nuances of different number types, the crucial role of variables in storing and manipulating data, and the power of operators to perform calculations and comparisons.
Numbers: The Foundation of Numerical Computation
Numbers are the most basic data type in programming, representing numerical values. Different programming languages handle numbers in various ways, offering different types to accommodate various ranges and precision levels. Let's examine some common number types:
Integers (Int)
Integers represent whole numbers without any fractional part. They can be positive, negative, or zero. For example: -3
, 0
, 10
, 1000
. The size of an integer (the range of values it can hold) depends on the specific programming language and the system architecture (32-bit or 64-bit).
Example (Python):
integer_variable = 10
print(integer_variable) # Output: 10
print(type(integer_variable)) # Output:
Floating-Point Numbers (Float)
Floating-point numbers represent numbers with fractional parts. They are used to represent real numbers, including decimal values. They are often represented using scientific notation internally (e.g., 1.23e+2 represents 123). The precision of floating-point numbers is limited; they can't represent all real numbers exactly due to the way they are stored in memory.
Example (JavaScript):
float_variable = 3.14159;
console.log(float_variable); // Output: 3.14159
console.log(typeof float_variable); // Output: number
Other Number Types
Some programming languages offer additional number types such as:
- Doubles: Similar to floats, but usually provide higher precision (more decimal places).
- Longs: Integers that can store larger values than standard integers.
- Shorts: Integers that use less memory than standard integers, suitable for smaller numbers.
- Decimals/BigDecimals: Used for precise decimal arithmetic, particularly crucial in financial applications where rounding errors are unacceptable.
The choice of number type depends heavily on the specific application. If you only need to represent whole numbers within a certain range, int
is efficient. For numbers with fractional parts, float
or double
are necessary. When extremely large numbers or high precision is required, specialized types like long
or decimal
are essential.
Variables: Dynamic Data Holders
Variables are symbolic names that refer to memory locations where data is stored. They are crucial for storing and manipulating data during program execution. Variables have a type (e.g., integer, floating-point) that determines the kind of data they can hold. The process of assigning a value to a variable is called initialization.
Key aspects of variables:
- Naming: Variable names should be descriptive and follow the naming conventions of the programming language. Many languages use camelCase (e.g.,
myVariable
) or snake_case (e.g.,my_variable
). - Declaration: In some languages (like C++, Java), you must declare the type of a variable before using it. In others (like Python), type inference automatically determines the type based on the assigned value.
- Scope: The scope of a variable refers to the part of the program where it is accessible. Variables can have global scope (accessible from anywhere) or local scope (accessible only within a specific function or block of code).
Example (C++):
int age = 30; // Declare an integer variable named 'age' and initialize it to 30.
double price = 99.99; // Declare a double variable and initialize it.
std::cout << "Age: " << age << ", Price: " << price << std::endl;
Example (Python):
age = 30
price = 99.99
print(f"Age: {age}, Price: {price}")
Operators: The Tools for Manipulation
Operators are symbols that perform operations on variables and values. They are essential for performing calculations, comparisons, and logical operations. Let's explore various operator categories:
Arithmetic Operators
These operators perform mathematical calculations:
+
(Addition)-
(Subtraction)*
(Multiplication)/
(Division)%
(Modulo – returns the remainder after division)**
(Exponentiation – raises a number to a power; available in some languages like Python)//
(Floor division – returns the integer part of the division; available in some languages like Python)
Example (Python):
x = 10
y = 3
print(f"x + y = {x + y}") # Output: 13
print(f"x - y = {x - y}") # Output: 7
print(f"x * y = {x * y}") # Output: 30
print(f"x / y = {x / y}") # Output: 3.3333333333333335
print(f"x % y = {x % y}") # Output: 1
print(f"x ** y = {x ** y}") # Output: 1000
print(f"x // y = {x // y}") # Output: 3
Comparison Operators
These operators compare two values and return a Boolean result (true or false):
==
(Equal to)!=
(Not equal to)>
(Greater than)<
(Less than)>=
(Greater than or equal to)<=
(Less than or equal to)
Example (JavaScript):
let a = 10;
let b = 5;
console.log(a == b); // Output: false
console.log(a > b); // Output: true
console.log(a <= b); // Output: false
Logical Operators
These operators combine or modify Boolean expressions:
&&
(Logical AND – true only if both operands are true)||
(Logical OR – true if at least one operand is true)!
(Logical NOT – inverts the Boolean value)
Example (C#):
bool isAdult = true;
bool hasLicense = false;
if (isAdult && hasLicense) {
Console.WriteLine("Can drive.");
} else {
Console.WriteLine("Cannot drive."); // This will be executed.
}
Assignment Operators
These operators assign values to variables:
=
(Simple assignment)+=
(Add and assign)-=
(Subtract and assign)*=
(Multiply and assign)/=
(Divide and assign)%=
(Modulo and assign)
Example (Java):
int count = 5;
count += 2; // count is now 7
count *= 3; // count is now 21
System.out.println(count); // Output: 21
Bitwise Operators
These operators perform operations on individual bits of integers:
&
(Bitwise AND)|
(Bitwise OR)^
(Bitwise XOR)~
(Bitwise NOT)<<
(Left shift)>>
(Right shift)
Bitwise operators are less frequently used than other operators but are powerful for low-level programming and bit manipulation tasks.
Combining Concepts: Building Practical Programs
The true power of numbers, variables, and operators becomes apparent when they are combined to solve problems. Let’s illustrate with a few examples:
Example 1: Calculating the area of a rectangle:
length = float(input("Enter the length of the rectangle: "))
width = float(input("Enter the width of the rectangle: "))
area = length * width
print(f"The area of the rectangle is: {area}")
This program uses variables to store the length and width, uses the multiplication operator to calculate the area, and then prints the result.
Example 2: Determining if a number is even or odd:
import java.util.Scanner;
public class EvenOdd {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter a number: ");
int number = input.nextInt();
if (number % 2 == 0) {
System.out.println(number + " is even.");
} else {
System.out.println(number + " is odd.");
}
}
}
This program utilizes the modulo operator to check if the remainder after dividing by 2 is 0. If it is, the number is even; otherwise, it's odd. This demonstrates the use of comparison and arithmetic operators within a conditional statement.
Example 3: Calculating the average of three numbers:
let num1 = parseFloat(prompt("Enter the first number:"));
let num2 = parseFloat(prompt("Enter the second number:"));
let num3 = parseFloat(prompt("Enter the third number:"));
let average = (num1 + num2 + num3) / 3;
console.log("The average is: " + average);
This uses variables to store the three numbers, performs addition and division to calculate the average, and displays the result. It showcases the practical application of arithmetic operators and variable manipulation.
These examples are just a glimpse of the possibilities. By mastering numbers, variables, and operators, you lay the groundwork for creating increasingly complex and sophisticated programs that can tackle a wide range of computational tasks. Understanding data types and their limitations is also crucial to writing robust and error-free code. Remember to always choose the most appropriate data type for the task at hand to optimize efficiency and prevent potential issues stemming from data overflow or precision limitations.
Latest Posts
Latest Posts
-
Adding And Subtracting Rational Expressions Solver
Mar 14, 2025
-
Which Of The Following Is A Semilunar Valve
Mar 14, 2025
-
The Lowest Rank Of Taxa Is The
Mar 14, 2025
-
Moment Of Inertia For A T Beam
Mar 14, 2025
-
What Is Explained By The Sliding Filament Theory
Mar 14, 2025
Related Post
Thank you for visiting our website which covers about Uses Numbers Variables And Operation Symbol . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.