Use Cross Product To Find Area Of Triangle
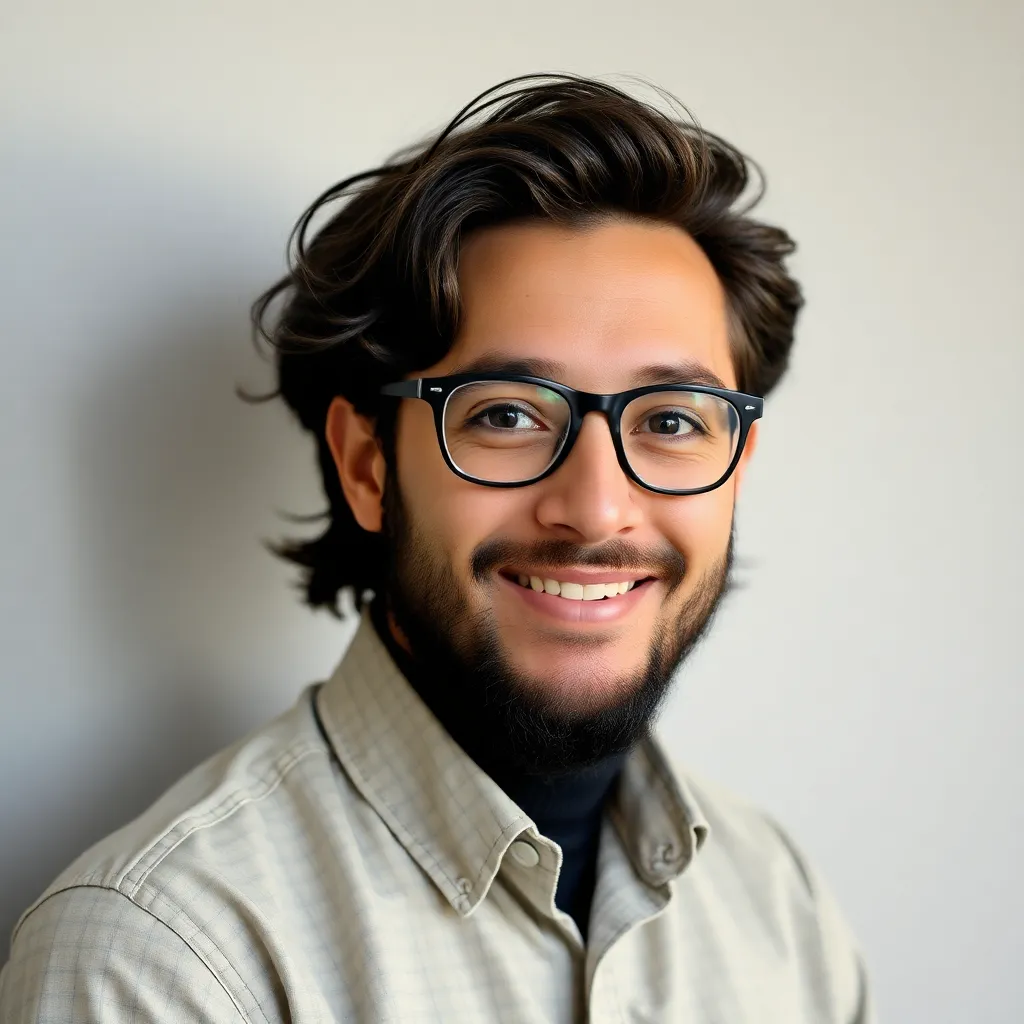
Juapaving
May 13, 2025 · 6 min read

Table of Contents
Using the Cross Product to Find the Area of a Triangle
Finding the area of a triangle is a fundamental concept in geometry, with applications spanning various fields, from basic surveying to advanced computer graphics. While the standard formula – half the base times the height – is well-known, it often requires additional calculations to determine the height, especially when dealing with triangles defined by their vertices in coordinate systems. This is where the power of the cross product shines. This article will delve into how to efficiently calculate the area of a triangle using the cross product, exploring its underlying principles and showcasing practical applications.
Understanding the Cross Product
Before diving into its application for triangle area calculation, let's review the cross product itself. The cross product is a binary operation on two vectors in three-dimensional space. The result is another vector that is perpendicular to both input vectors. This new vector’s magnitude is directly related to the area of the parallelogram formed by the two original vectors.
Let's consider two vectors, a and b, represented in Cartesian coordinates as:
a = (a<sub>x</sub>, a<sub>y</sub>, a<sub>z</sub>)
b = (b<sub>x</sub>, b<sub>y</sub>, b<sub>z</sub>)
The cross product c = a x b is calculated as follows:
c = (a<sub>y</sub>b<sub>z</sub> - a<sub>z</sub>b<sub>y</sub>, a<sub>z</sub>b<sub>x</sub> - a<sub>x</sub>b<sub>z</sub>, a<sub>x</sub>b<sub>y</sub> - a<sub>y</sub>b<sub>x</sub>)
The magnitude of the cross product, ||c||, is given by:
||c|| = √[(a<sub>y</sub>b<sub>z</sub> - a<sub>z</sub>b<sub>y</sub>)² + (a<sub>z</sub>b<sub>x</sub> - a<sub>x</sub>b<sub>z</sub>)² + (a<sub>x</sub>b<sub>y</sub> - a<sub>y</sub>b<sub>x</sub>)²]
This magnitude represents the area of the parallelogram formed by vectors a and b.
Connecting the Cross Product to Triangle Area
Since a triangle is half of a parallelogram, the area of a triangle formed by two vectors, a and b, is simply half the magnitude of their cross product:
Area of Triangle = (1/2) ||a x b||
This elegantly avoids the need to explicitly calculate the height of the triangle. This method is particularly useful when dealing with triangles defined by their vertices in a three-dimensional coordinate system.
Practical Applications and Examples
Let’s illustrate this with a few examples.
Example 1: 2D Triangle
Consider a triangle with vertices A(1, 2), B(4, 6), and C(7, 3). To use the cross product method, we need to treat these points as vectors. We can do this by defining vectors from one vertex to the others. Let's choose A as the origin:
- a = B - A = (4-1, 6-2) = (3, 4)
- b = C - A = (7-1, 3-2) = (6, 1)
Note: For 2D calculations, we can treat these as 3D vectors with a z-component of 0: a = (3, 4, 0) and b = (6, 1, 0).
The cross product is:
c = a x b = (0, 0, 3(1) - 4(6)) = (0, 0, -21)
The magnitude of c is ||c|| = √(0² + 0² + (-21)²) = 21
Therefore, the area of the triangle is:
Area = (1/2) * 21 = 10.5 square units.
Example 2: 3D Triangle
Let's consider a triangle in 3D space with vertices A(1, 2, 3), B(4, 1, 0), and C(2, 5, 2). Again, we define vectors:
- a = B - A = (3, -1, -3)
- b = C - A = (1, 3, -1)
The cross product is:
c = a x b = ((-1)(-1) - (-3)(3), (-3)(1) - (3)(-1), (3)(3) - (-1)(1)) = (10, 0, 10)
The magnitude of c is ||c|| = √(10² + 0² + 10²) = √200 = 10√2
Therefore, the area of the triangle is:
Area = (1/2) * 10√2 = 5√2 square units.
Advantages of Using the Cross Product
The cross product method offers several key advantages over other methods for calculating triangle areas:
- Efficiency: It directly calculates the area without needing to find the height, simplifying the calculation, especially in higher dimensions.
- Simplicity: The formula is concise and easy to implement in programming and mathematical calculations.
- Versatility: It works seamlessly in both 2D and 3D spaces.
- Geometric Insight: It provides a deeper understanding of the relationship between vectors and areas.
Handling Degenerate Cases
A degenerate triangle is one where the three vertices are collinear (lie on the same line). In such cases, the area of the triangle is zero. This is reflected in the cross product method because the cross product of two collinear vectors is the zero vector (magnitude 0).
Implementation in Different Programming Languages
The cross product calculation and subsequent area determination are straightforward to implement in various programming languages. The core mathematical operations are consistent, mainly involving vector subtraction, cross product computation, and magnitude calculation. Below are conceptual outlines – the specific syntax would depend on the language chosen.
Python:
import numpy as np
def triangle_area(A, B, C):
a = np.array(B) - np.array(A)
b = np.array(C) - np.array(A)
cross_product = np.cross(a, b)
area = 0.5 * np.linalg.norm(cross_product)
return area
# Example usage
A = [1, 2, 3]
B = [4, 1, 0]
C = [2, 5, 2]
area = triangle_area(A, B, C)
print(f"The area of the triangle is: {area}")
JavaScript:
function triangleArea(A, B, C) {
const a = {x: B.x - A.x, y: B.y - A.y, z: B.z - A.z};
const b = {x: C.x - A.x, y: C.y - A.y, z: C.z - A.z};
const crossProduct = {
x: a.y * b.z - a.z * b.y,
y: a.z * b.x - a.x * b.z,
z: a.x * b.y - a.y * b.x
};
const magnitude = Math.sqrt(crossProduct.x**2 + crossProduct.y**2 + crossProduct.z**2);
return 0.5 * magnitude;
}
// Example usage
const A = {x: 1, y: 2, z: 3};
const B = {x: 4, y: 1, z: 0};
const C = {x: 2, y: 5, z: 2};
const area = triangleArea(A, B, C);
console.log(`The area of the triangle is: ${area}`);
These are illustrative examples; error handling (e.g., for non-numeric inputs) should be added for robust production code.
Conclusion
The cross product provides a powerful and elegant method for calculating the area of a triangle, particularly when dealing with vertices defined in coordinate systems. Its efficiency, simplicity, and versatility make it a valuable tool in various applications, from mathematical problem-solving to computer graphics and engineering simulations. Understanding and utilizing this method can significantly simplify calculations and enhance the overall efficiency of your work. The method’s adaptability across different programming languages makes it a readily accessible technique for a broad range of users.
Latest Posts
Latest Posts
-
Turgor Pressure Is The Result Of
May 13, 2025
-
What Is Cube Root Of 27
May 13, 2025
-
Chemiosmosis Atp Synthesis In Chloroplasts Answer Key
May 13, 2025
-
3 Basic Components Of A Nucleotide
May 13, 2025
-
What Is Prime Factorization Of 144
May 13, 2025
Related Post
Thank you for visiting our website which covers about Use Cross Product To Find Area Of Triangle . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.