How To Check If Three Points Are Collinear
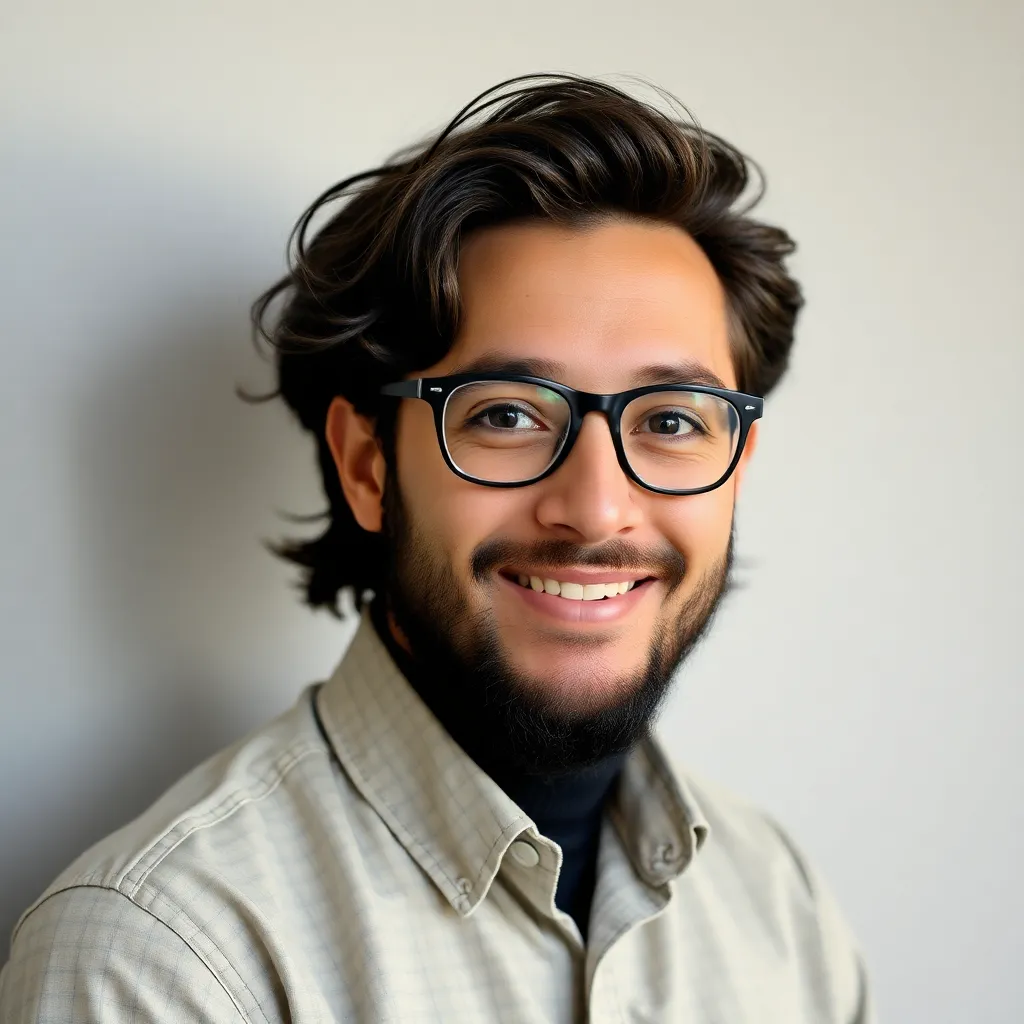
Juapaving
Mar 27, 2025 · 5 min read
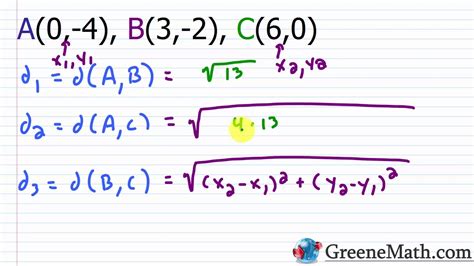
Table of Contents
How to Check if Three Points are Collinear: A Comprehensive Guide
Determining whether three points lie on the same straight line – a concept known as collinearity – is a fundamental problem in geometry with applications across various fields, including computer graphics, geographic information systems (GIS), and physics. This comprehensive guide explores multiple methods for checking collinearity, ranging from basic geometric principles to more advanced techniques suitable for programming. We'll delve into the underlying mathematics, provide practical examples, and offer insights into efficient computational strategies.
Understanding Collinearity
Before diving into the methods, let's clarify what collinearity means. Three points, let's call them A, B, and C, are collinear if and only if they lie on the same straight line. There's no deviation; they perfectly align. This seemingly simple concept has significant implications in various applications. For instance, in computer graphics, determining collinearity can help optimize algorithms for rendering lines and polygons. In GIS, it aids in identifying spatial relationships between geographical features.
Methods for Checking Collinearity
Several approaches exist for determining whether three points are collinear. Each method offers a unique perspective and computational efficiency, making the choice dependent on the specific context and available tools.
1. Using the Slope Formula
This is perhaps the most intuitive method, especially for those familiar with basic algebra. The slope of a line connecting two points (x₁, y₁) and (x₂, y₂) is given by:
m = (y₂ - y₁) / (x₂ - x₁)
If three points A(x₁, y₁), B(x₂, y₂), and C(x₃, y₃) are collinear, then the slope between any two pairs of points must be the same. Therefore, we can check collinearity by calculating the slopes between AB and BC (or AB and AC, or BC and AC – any two pairs will suffice):
- m₁ = (y₂ - y₁) / (x₂ - x₁)
- m₂ = (y₃ - y₂) / (x₃ - x₂)
If m₁ = m₂, then the points A, B, and C are collinear.
Caveat: This method fails when the line is vertical (x₂ - x₁ = 0 or x₃ - x₂ = 0). A vertical line has an undefined slope. We'll address this limitation in subsequent methods.
2. Using the Area of a Triangle
Another approach leverages the concept of the area of a triangle. The area of a triangle with vertices (x₁, y₁), (x₂, y₂), and (x₃, y₃) can be calculated using the determinant formula:
Area = 0.5 * |x₁(y₂ - y₃) + x₂(y₃ - y₁) + x₃(y₁ - y₂)|
If the three points are collinear, they form a degenerate triangle with zero area. Therefore, if the calculated area is 0, the points are collinear. This method elegantly handles both vertical and horizontal lines, overcoming the slope method's limitation.
3. Using the Cross Product (Vector Approach)
This method employs vector mathematics. We can represent the points as vectors:
- Vector AB = (x₂ - x₁, y₂ - y₁)
- Vector AC = (x₃ - x₁, y₃ - y₁)
The cross product of two vectors in 2D space is a scalar value given by:
Cross Product = (x₂ - x₁)(y₃ - y₁) - (y₂ - y₁)(x₃ - x₁)
If the cross product is 0, the vectors are parallel, indicating that the points are collinear. This method is robust and handles all cases, including vertical and horizontal lines.
4. Using the Distance Formula
This less-efficient but conceptually simple method checks if the sum of the distances between pairs of points equals the distance between the other pair. If points A, B, and C are collinear, then:
AB + BC = AC or AB + AC = BC or AC + BC = AB (depending on the order of points)
This method uses the distance formula:
Distance = √((x₂ - x₁)² + (y₂ - y₁)²)
While straightforward, this method is computationally expensive compared to others, particularly when dealing with many points.
Computational Considerations and Algorithm Efficiency
When implementing collinearity checks in programming, efficiency is crucial, especially when dealing with a large number of points. The area of a triangle method and the cross-product method are generally preferred for their efficiency and robustness.
Here's a Python code example demonstrating the area of a triangle method:
def are_collinear(x1, y1, x2, y2, x3, y3):
"""Checks if three points are collinear using the area of a triangle method."""
area = 0.5 * abs(x1 * (y2 - y3) + x2 * (y3 - y1) + x3 * (y1 - y2))
return area == 0
# Example usage:
x1, y1 = 1, 1
x2, y2 = 2, 2
x3, y3 = 3, 3
if are_collinear(x1, y1, x2, y2, x3, y3):
print("Points are collinear")
else:
print("Points are not collinear")
x1, y1 = 1, 1
x2, y2 = 2, 3
x3, y3 = 3, 2
if are_collinear(x1, y1, x2, y2, x3, y3):
print("Points are collinear")
else:
print("Points are not collinear")
This code snippet provides a concise and efficient way to check for collinearity. Remember to adapt the code to your preferred programming language and incorporate appropriate error handling for real-world applications.
Applications of Collinearity
The ability to efficiently determine collinearity is vital in various fields:
-
Computer Graphics: Collinearity checks are used in algorithms for line clipping, polygon filling, and hidden surface removal.
-
Geographic Information Systems (GIS): Identifying collinear points helps in simplifying geographical data, detecting errors in spatial data, and performing spatial analysis.
-
Computer Vision: Collinearity is crucial in tasks such as object recognition, feature extraction, and image alignment.
-
Robotics: Path planning and obstacle avoidance algorithms often rely on collinearity checks to optimize robot movement.
-
Physics and Engineering: Collinearity is relevant in mechanics, structural analysis, and other areas involving the interaction of forces and objects along a single line.
Conclusion
Checking whether three points are collinear is a fundamental geometric problem with widespread practical applications. While the slope method offers an intuitive approach, the area of a triangle method and the cross-product method provide more robust and computationally efficient solutions, particularly when dealing with large datasets or potential vertical lines. Understanding these methods and their associated computational considerations allows for informed decision-making in selecting the most appropriate technique for a given application. Remember to consider factors like computational cost and robustness when choosing a method for your specific use case. Efficient implementation in programming, as shown in the Python example, is key to leveraging these methods effectively in real-world scenarios.
Latest Posts
Latest Posts
-
Lowest Common Factor Of 3 And 8
Mar 30, 2025
-
Five Letter Words Starting With Ra
Mar 30, 2025
-
What Is The Chemical Formula For Phosphorus Pentachloride
Mar 30, 2025
-
Least Common Multiple Of 7 And 16
Mar 30, 2025
-
How Is A Sound Wave Different From A Light Wave
Mar 30, 2025
Related Post
Thank you for visiting our website which covers about How To Check If Three Points Are Collinear . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.