3-3 Assignment Introduction To Pseudocode And Flowcharts
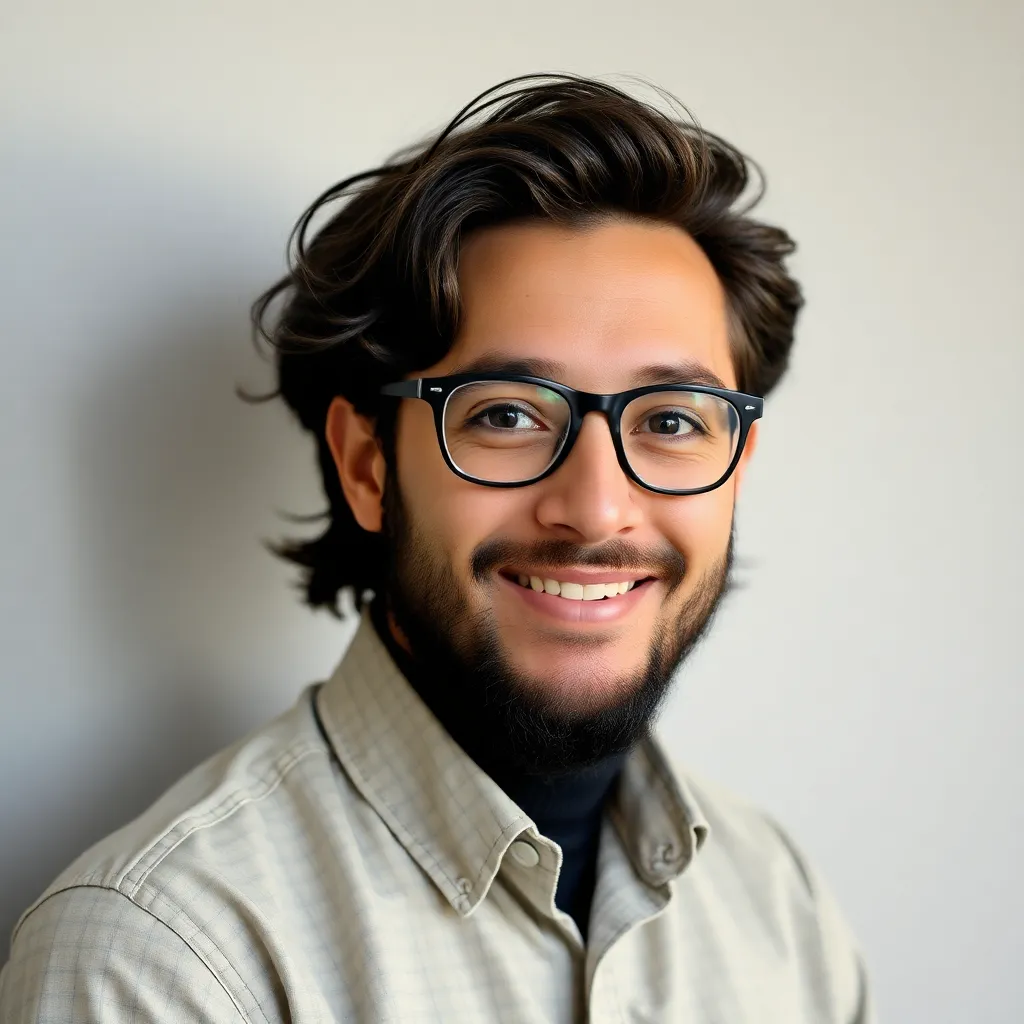
Juapaving
May 24, 2025 · 5 min read
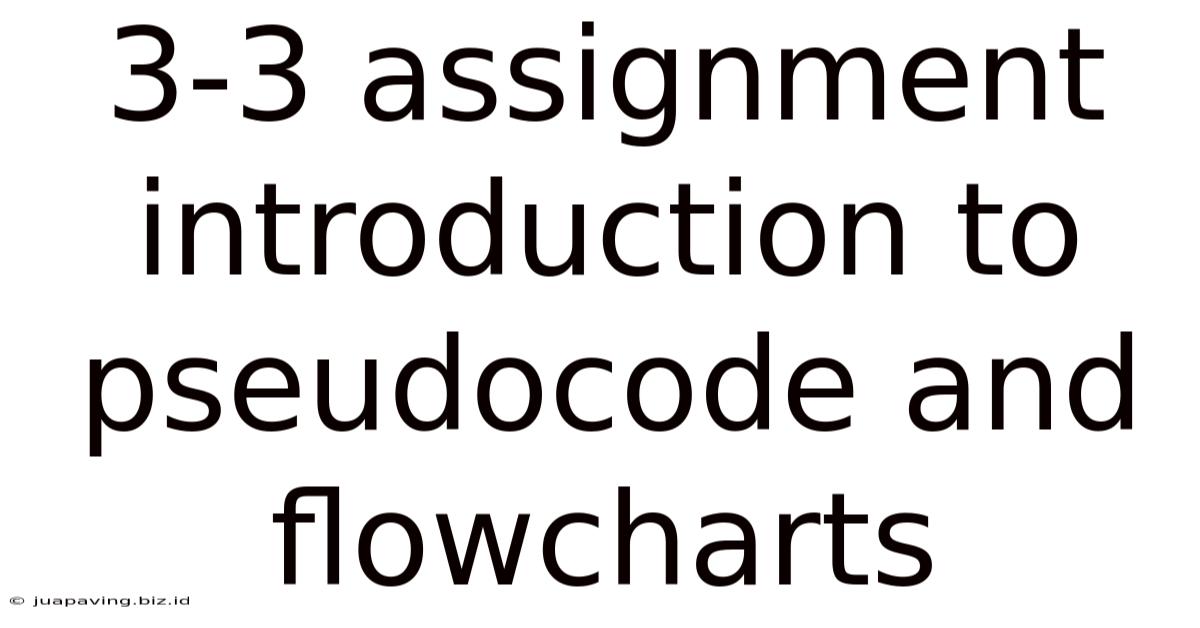
Table of Contents
3-3 Assignment: Introduction to Pseudocode and Flowcharts
This comprehensive guide delves into the world of pseudocode and flowcharts, essential tools for programmers and problem-solvers alike. We'll explore their individual strengths, demonstrate how they work together, and provide practical examples to solidify your understanding. This guide is designed to be a complete resource for your 3-3 assignment, offering more than enough information to excel.
Understanding Pseudocode
Pseudocode is a simplified, informal way of describing an algorithm. It's not a programming language; instead, it bridges the gap between human language and actual code. Think of it as a detailed plan for your program, written in a way that's easily understandable by both humans and, with some practice, computers.
Key Features of Effective Pseudocode:
- Readability: The primary goal is clarity. Use plain English (or your preferred language) and avoid overly technical jargon.
- Structure: Employ indentation to highlight blocks of code and show the logical flow. Keywords like
IF
,THEN
,ELSE
,WHILE
,FOR
, etc., help structure the algorithm. - Abstraction: Focus on the what and how of the algorithm, not the specific syntax of a particular programming language.
- Modularity: Break down complex tasks into smaller, manageable subroutines or functions. This improves readability and maintainability.
Example: Let's create pseudocode for a simple program that calculates the average of three numbers.
INPUT num1, num2, num3
sum = num1 + num2 + num3
average = sum / 3
OUTPUT average
This is a basic example. More complex algorithms will require more detailed pseudocode, incorporating conditional statements, loops, and function calls.
Advanced Pseudocode Concepts
As your algorithms become more complex, you'll need to incorporate more advanced structures:
- Conditional Statements (IF-THEN-ELSE): These control the flow of execution based on conditions.
IF age >= 18 THEN
OUTPUT "You are an adult."
ELSE
OUTPUT "You are a minor."
ENDIF
- Loops (WHILE and FOR): Loops repeat a block of code multiple times.
// WHILE loop
WHILE count < 10 DO
OUTPUT count
count = count + 1
ENDWHILE
// FOR loop (iterating through an array)
FOR each item IN array DO
PROCESS item
ENDFOR
- Functions/Procedures: These encapsulate reusable blocks of code.
FUNCTION calculateArea(length, width)
area = length * width
RETURN area
ENDFUNCTION
main program:
area = calculateArea(5, 10)
OUTPUT area
- Data Structures: Pseudocode can represent various data structures, such as arrays, linked lists, stacks, and queues, to manage data efficiently. This allows you to outline how your algorithm will handle and manipulate data.
Understanding Flowcharts
Flowcharts are visual representations of algorithms. They use standard symbols to depict different steps and the flow of control between them. This visual approach makes it easier to understand the logic of an algorithm, especially for complex problems.
Common Flowchart Symbols:
- Oval: Represents the start and end points of the flowchart.
- Rectangle: Represents a processing step (e.g., an assignment, calculation).
- Parallelogram: Represents input or output operations.
- Diamond: Represents a decision point (e.g., an IF-THEN-ELSE statement).
- Arrow: Shows the direction of flow between steps.
Example: Let's create a flowchart for the same average calculation program as before:
[Start] --> [Input num1, num2, num3] --> [sum = num1 + num2 + num3] --> [average = sum / 3] --> [Output average] --> [End]
This is a simplified flowchart. More complex algorithms will involve multiple decision points and loops.
Advanced Flowcharting Techniques
For more sophisticated algorithms, you'll need to use more advanced flowcharting techniques:
-
Subroutines/Functions: Representing functions as separate flowcharts or using a predefined symbol for function calls. This improves the readability and organization of the overall flowchart.
-
Multiple Branches (Decision Points): Handling multiple conditions within a single decision point, leading to multiple possible paths of execution.
-
Loops (Iteration): Representing loops using looping constructs, showing clearly the conditions for loop entry and exit.
-
Data Structures: While not explicitly represented by a specific symbol, the flowchart should clearly show how data is manipulated and passed between different parts of the algorithm.
Combining Pseudocode and Flowcharts
Pseudocode and flowcharts are powerful tools when used together. Pseudocode provides a detailed textual description, while the flowchart offers a visual representation. This combination offers several advantages:
- Improved Clarity: The textual detail of pseudocode combined with the visual overview of the flowchart enhances understanding.
- Easier Debugging: Errors in logic are often easier to spot when examining both representations.
- Better Communication: Sharing both pseudocode and a flowchart with others facilitates easier collaboration and communication of the algorithm's design.
- Enhanced Documentation: They serve as excellent documentation for the program, making it easier to understand and maintain the code in the future.
Workflow: A typical workflow involves first creating a high-level flowchart to visualize the overall flow, then developing detailed pseudocode for individual components. The pseudocode then guides the detailed implementation of the program in a specific programming language.
Practical Application and Examples
Let's look at a more complex example: a program that determines whether a number is prime. We'll develop both pseudocode and a flowchart.
Pseudocode:
FUNCTION isPrime(number)
IF number <= 1 THEN
RETURN false
ENDIF
FOR i = 2 TO sqrt(number) DO
IF number % i == 0 THEN
RETURN false
ENDIF
ENDFOR
RETURN true
ENDFUNCTION
main program:
INPUT number
IF isPrime(number) THEN
OUTPUT number + " is a prime number."
ELSE
OUTPUT number + " is not a prime number."
ENDIF
Flowchart: (A textual representation since graphical flowcharts are difficult to render in Markdown)
[Start] --> [Input number] --> [number <= 1?] --> [Yes] --> [Output "Not prime"] --> [End]
|
No
|
-->[i = 2] --> [i <= sqrt(number)?] --> [Yes] --> [number % i == 0?] --> [Yes] --> [Output "Not prime"] --> [End]
|
No
|
-->[i = i + 1] --> [Loop back to i <= sqrt(number)?]
|
No
-->[Output "Prime"] --> [End]
This example demonstrates a more complex algorithm involving a function, a loop, and multiple decision points. The combination of pseudocode and the described flowchart provides a clearer and more complete understanding of the algorithm than either alone.
Conclusion
Pseudocode and flowcharts are invaluable tools for programmers and problem-solvers at all levels. They enhance the design, development, and documentation of algorithms, leading to cleaner, more efficient, and easier-to-maintain code. Mastering these techniques is crucial for success in any programming endeavor. This guide has provided a comprehensive overview, equipping you with the knowledge to tackle your 3-3 assignment and beyond. Remember, practice is key; the more you use pseudocode and flowcharts, the more proficient and confident you will become. Apply these techniques to diverse problems, and you'll quickly discover their immense value in your programming journey. Good luck with your assignment!
Latest Posts
Latest Posts
-
Using The Count Data And Observational Data
May 24, 2025
-
What Type Of Questions Are On The Ged Test
May 24, 2025
-
Spark Notes A Tale Of Two Cities
May 24, 2025
-
Match The Relationships To The Corresponding Concepts
May 24, 2025
-
Perfect Competition Is Characterized By All Of The Following Except
May 24, 2025
Related Post
Thank you for visiting our website which covers about 3-3 Assignment Introduction To Pseudocode And Flowcharts . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.