Which Option Rotates The Square 90 Degrees
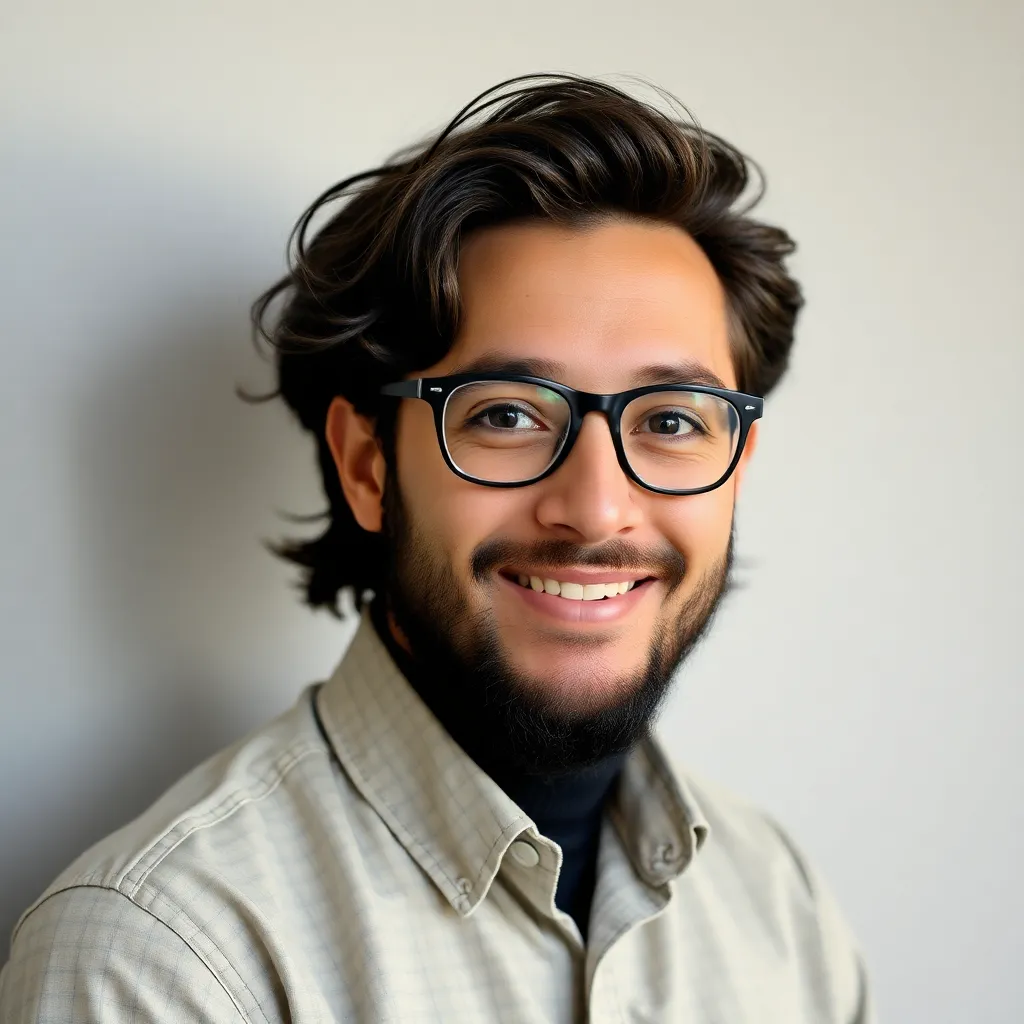
Juapaving
May 13, 2025 · 5 min read
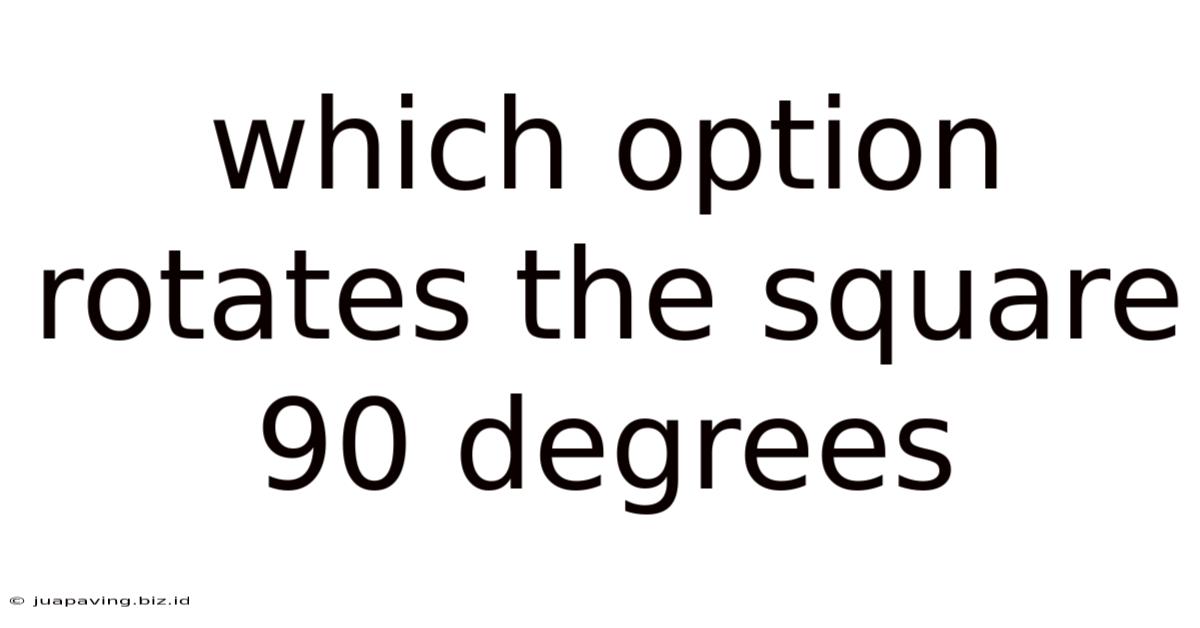
Table of Contents
Which Option Rotates the Square 90 Degrees? A Deep Dive into Rotation Transformations
Rotating a square 90 degrees might seem simple, but understanding the underlying mathematics and various methods reveals a fascinating world of transformations. This comprehensive guide explores different approaches to achieve a 90-degree rotation, focusing on the theoretical underpinnings and practical applications in computer graphics, game development, and other fields.
Understanding Rotation: The Basics
Before diving into specific methods, let's establish a fundamental understanding of rotation. Rotation is a geometric transformation that moves points around a fixed point called the center of rotation. The amount of rotation is specified by an angle, usually measured in degrees or radians. A 90-degree rotation means each point in the square moves 90 degrees around the center of rotation.
The center of rotation can be anywhere, but common choices include the center of the square itself or one of its vertices. The direction of rotation is also crucial; it's typically described as clockwise or counter-clockwise. In many systems, counter-clockwise is considered positive rotation.
Matrix Transformations: The Power of Linear Algebra
One of the most efficient and elegant ways to perform a 90-degree rotation is using rotation matrices. This method leverages the power of linear algebra to represent and manipulate geometric transformations. A 2D rotation matrix for a counter-clockwise rotation by an angle θ is:
[ cos(θ) -sin(θ) ]
[ sin(θ) cos(θ) ]
For a 90-degree rotation (θ = 90°), the matrix simplifies to:
[ 0 -1 ]
[ 1 0 ]
To rotate a point (x, y), we represent it as a column vector:
[ x ]
[ y ]
Multiplying the rotation matrix by this vector gives the rotated coordinates (x', y'):
[ x' ] [ 0 -1 ] [ x ] [ -y ]
[ y' ] = [ 1 0 ] [ y ] = [ x ]
Therefore, to rotate a square 90 degrees counter-clockwise, we apply this transformation to each of its vertices. Let's assume the square's vertices are (0,0), (1,0), (1,1), and (0,1). After the rotation:
- (0,0) remains (0,0)
- (1,0) becomes (0,1)
- (1,1) becomes (-1,1)
- (0,1) becomes (-1,0)
Implementing Rotation in Programming
Different programming languages and libraries provide various ways to implement these matrix transformations. Here's a conceptual illustration using Python:
import numpy as np
def rotate_90(point):
"""Rotates a point 90 degrees counter-clockwise."""
rotation_matrix = np.array([[0, -1], [1, 0]])
return np.dot(rotation_matrix, point)
# Example usage:
square_vertices = np.array([[0, 0], [1, 0], [1, 1], [0, 1]])
rotated_vertices = np.apply_along_axis(rotate_90, 1, square_vertices)
print(rotated_vertices)
This code snippet uses NumPy, a powerful library for numerical computation, to perform the matrix multiplication efficiently. Similar approaches can be adapted for other languages like C++, Java, or JavaScript using their respective linear algebra libraries.
Quaternion Rotations: Handling 3D and Beyond
While matrix transformations work well in 2D, for 3D rotations and more complex scenarios, quaternions offer a more efficient and robust solution. Quaternions are a mathematical extension of complex numbers, providing a compact and elegant way to represent rotations in any dimension. They avoid the problems of gimbal lock, which can occur with Euler angles (another common rotation representation). The use of quaternions is crucial in applications like 3D game development and animation. While understanding quaternions requires a deeper mathematical background, libraries readily handle quaternion-based rotations.
Other Rotation Methods: Exploring Alternatives
Besides matrix transformations and quaternions, other methods exist for rotating a square 90 degrees, albeit often less efficient or elegant:
-
Individual Point Rotation: This involves rotating each point of the square individually using trigonometric functions (sine and cosine) to calculate the new coordinates. This method is straightforward but can be less computationally efficient than matrix transformations for larger shapes.
-
Transformation Libraries: Many graphics and game development libraries (e.g., OpenGL, DirectX, Unity) provide built-in functions for rotations. These libraries often handle the underlying mathematical complexities, simplifying the process for developers. Using these libraries leverages optimizations and ensures compatibility across various platforms.
-
Graphical User Interfaces (GUIs): GUIs often provide drag-and-drop functionalities or simple controls to rotate objects. Internally, these interfaces may use one of the previously discussed methods, abstracting the complexity away from the user.
Applications of 90-degree Rotations
The seemingly simple 90-degree rotation has numerous applications across diverse fields:
-
Image Processing: Rotating images by 90 degrees is a common operation in image editing and processing.
-
Computer Graphics: In computer graphics, rotations are fundamental for creating and manipulating 3D models, animations, and scenes.
-
Game Development: 90-degree rotations are essential for controlling character movement, camera angles, and object manipulation in games.
-
Robotics: Robots often require precise rotational movements, and 90-degree rotations are fundamental in many robotic applications.
-
Signal Processing: Signal processing uses rotations in various contexts, often within the framework of Fourier transforms and related techniques.
Choosing the Right Rotation Method: A Practical Guide
The optimal method for rotating a square 90 degrees depends on various factors:
-
Dimensionality: For 2D rotations, matrix transformations are generally efficient and straightforward. For 3D and higher dimensions, quaternions are preferred to avoid gimbal lock.
-
Performance Requirements: In performance-critical applications like real-time games, using optimized libraries or specialized hardware acceleration is essential.
-
Development Resources: If using a game engine or graphics library with built-in rotation functions, leveraging these functions is often simpler and more robust.
-
Mathematical Background: If you have a strong mathematical background, implementing matrix transformations or quaternion rotations yourself can offer more control and understanding.
Conclusion: Mastering 90-Degree Rotations
Rotating a square 90 degrees might appear trivial at first glance, but exploring the different methods reveals a rich tapestry of mathematical concepts and practical applications. Understanding matrix transformations, quaternions, and the various libraries and techniques available empowers you to tackle complex geometric transformations efficiently and effectively. Choosing the right method depends on your specific context, balancing performance, simplicity, and the tools at your disposal. Whether you're working with images, 3D models, or robotic systems, mastering 90-degree rotations is a cornerstone skill in numerous fields. The information presented here provides a solid foundation to understand and implement this fundamental transformation.
Latest Posts
Latest Posts
-
2 Gallons Is How Many Liters
May 13, 2025
-
What Temp Does Water Boil Kelvin
May 13, 2025
-
An Object At Rest May Have
May 13, 2025
-
1 Us Dollar Is Equal To How Many Cents
May 13, 2025
-
Advantages Of Alternating Current Over Direct Current
May 13, 2025
Related Post
Thank you for visiting our website which covers about Which Option Rotates The Square 90 Degrees . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.