Which Of The Following Is An Example Of A Parameter
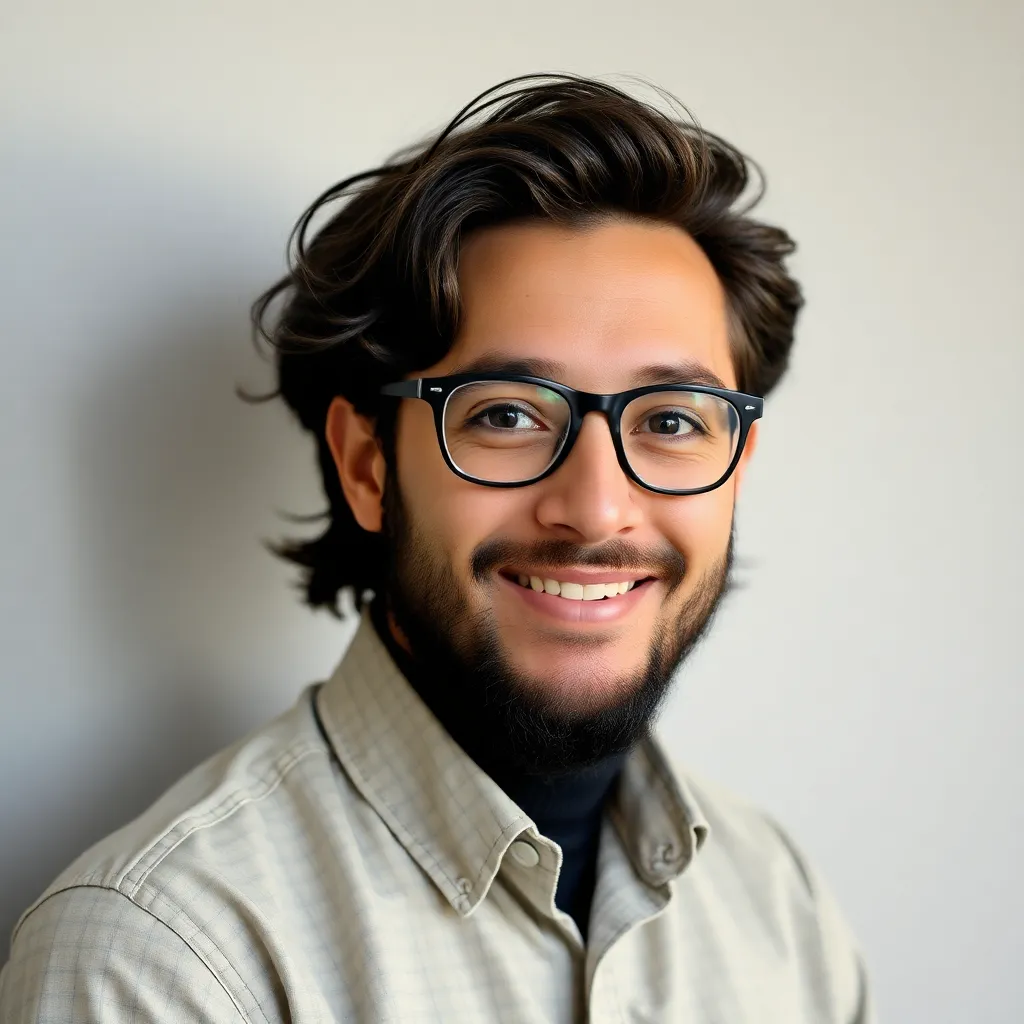
Juapaving
May 23, 2025 · 5 min read
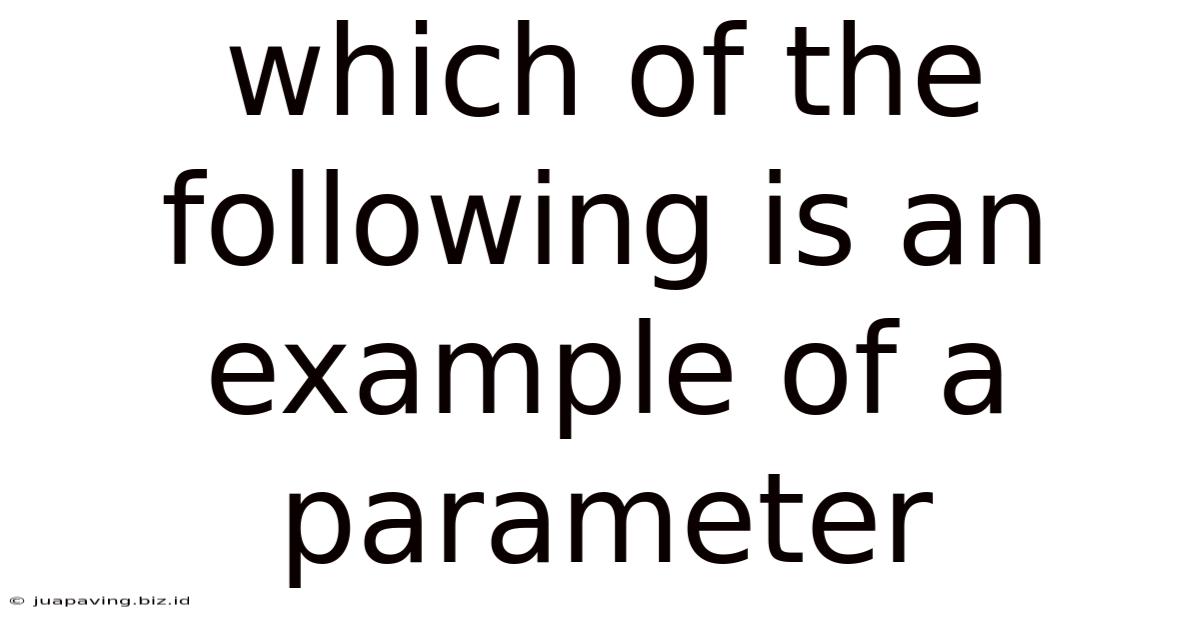
Table of Contents
Which of the Following is an Example of a Parameter? Understanding Parameters in Programming
The question, "Which of the following is an example of a parameter?" hinges on a fundamental concept in programming: understanding the difference between parameters and arguments. While often used interchangeably in casual conversation, they represent distinct parts of a function or method's structure. This article will delve deep into the definition of parameters, provide clear examples, differentiate them from arguments, explore their significance in various programming paradigms, and discuss common scenarios where understanding parameters is crucial.
Defining Parameters: The Blueprint of a Function
In the world of programming, a parameter is a variable listed inside the parentheses of a function or method's definition. It acts as a placeholder, a symbolic representation of the data the function expects to receive when it's called. Think of it as the blueprint or the template for the input the function will process. Parameters essentially define the interface of a function—what kind of data the function is designed to work with.
Example (Python):
def greet(name, greeting): # name and greeting are parameters
print(f"{greeting}, {name}!")
greet("Alice", "Hello") # "Alice" and "Hello" are arguments
In this Python example, name
and greeting
are parameters. They specify that the greet
function anticipates receiving two inputs: a string representing a name and a string representing a greeting.
Example (JavaScript):
function calculateArea(length, width) { // length and width are parameters
return length * width;
}
let area = calculateArea(5, 10); // 5 and 10 are arguments
Here, length
and width
serve as parameters for the calculateArea
function, indicating that it expects two numerical inputs representing the length and width of a rectangle.
Differentiating Parameters and Arguments: The Blueprint vs. The House
The key distinction lies in the when and how they are used. Parameters are defined in the function's declaration, whereas arguments are provided when the function is called. You define the blueprint (parameters) and then build the house (arguments) according to that blueprint.
-
Parameters: Part of the function's definition. They are placeholders for the values the function will receive. They are declared within the parentheses of a function's header.
-
Arguments: The actual values passed to the function when it's invoked. They are supplied during the function's call.
Therefore, in the examples above, name
and greeting
are parameters, while "Alice"
and "Hello"
(in the Python example) and 5
and 10
(in the JavaScript example) are the arguments.
Types of Parameters: Adding Specificity
Programming languages offer various mechanisms to define parameters with increased precision, enhancing code readability and robustness.
-
Required Parameters: These parameters must be provided when the function is called. Omitting a required parameter will result in a runtime error. All examples shown above demonstrate required parameters.
-
Optional Parameters (or Default Parameters): These parameters have default values assigned in the function definition. If the caller doesn't provide a value for an optional parameter, the default value is used.
Example (Python):
def greet(name, greeting="Hello"): # greeting is an optional parameter
print(f"{greeting}, {name}!")
greet("Bob") # Uses the default greeting "Hello"
greet("Charlie", "Good morning") # Overrides the default greeting
- Keyword Arguments: In many languages, arguments can be passed using keywords, making the code more readable and less prone to errors, especially when dealing with many parameters.
Example (Python):
def describe_pet(animal_type, pet_name, age=None):
print(f"\nI have a {animal_type}.")
print(f"My {animal_type}'s name is {pet_name.title()}.")
if age:
print(f"My {animal_type} is {age} years old.")
describe_pet(animal_type='hamster', pet_name='harry')
describe_pet(pet_name='willie', animal_type='dog', age=5)
- **Variable-Length Arguments (*args and kwargs): Python allows for functions to accept a variable number of arguments using
*args
(for positional arguments) and**kwargs
(for keyword arguments). This is particularly useful when the number of inputs is unknown beforehand.
Example (Python):
def sum_all_numbers(*args):
total = 0
for number in args:
total += number
return total
print(sum_all_numbers(1, 2, 3, 4, 5)) # Output: 15
Parameters in Different Programming Paradigms
The role and implementation of parameters vary slightly across different programming paradigms.
-
Procedural Programming: Parameters are fundamental to passing data to procedures (functions or subroutines). They're used to control the behavior and output of these procedures.
-
Object-Oriented Programming (OOP): Parameters are crucial in methods (functions within classes). They allow objects to interact and exchange data. Constructor methods (like
__init__
in Python) often use parameters to initialize an object's attributes. -
Functional Programming: Functions are treated as first-class citizens, and parameters are used extensively to pass data between functions, often as immutable values to maintain referential transparency.
The Significance of Parameters: Why They Matter
A strong grasp of parameters is vital for several reasons:
-
Code Reusability: Functions with well-defined parameters are highly reusable. They can be called with different arguments, leading to efficient and concise code.
-
Modularity: Parameters contribute to modular design, breaking down complex problems into smaller, manageable functions.
-
Abstraction: Parameters hide implementation details, allowing users to interact with a function without needing to understand its internal workings.
-
Maintainability: Well-defined parameters improve code readability and make it easier to maintain and debug.
Common Scenarios Where Parameter Understanding is Crucial
Several programming tasks heavily rely on the correct use of parameters.
-
Data Validation: Parameters allow functions to check the validity of input data before processing it, preventing errors and unexpected behavior.
-
Error Handling: Parameters help in identifying and handling potential errors associated with input data.
-
Algorithm Implementation: Algorithms often require various inputs, and parameters provide a structured way to pass these inputs.
-
Event Handling: Event handlers (in GUI programming or other event-driven architectures) use parameters to convey information about the event that has occurred.
-
Database Interactions: Database queries and procedures typically use parameters to specify data to be retrieved or modified.
Conclusion: Mastering the Art of Parameters
Parameters are the unsung heroes of programming, quietly shaping the functionality and efficiency of our code. By understanding their definition, types, and interactions with arguments, programmers significantly enhance their ability to write clean, reusable, and robust code. Mastering parameters is a cornerstone of efficient and effective software development, paving the way for more complex and sophisticated projects. The examples provided throughout this article illustrate not only the basic concept of parameters but also highlight the crucial role they play in various programming contexts, making this knowledge indispensable for any programmer, regardless of experience level. From simple functions to complex algorithms, the careful consideration and implementation of parameters are vital for producing high-quality, maintainable software.
Latest Posts
Latest Posts
-
Ap World History Unit 1 Notes Pdf
May 23, 2025
-
Give Me Liberty Chapter 3 Summary
May 23, 2025
-
Characters In Red Badge Of Courage
May 23, 2025
-
The Communication Related Activity Organizations Role Is To
May 23, 2025
-
Who Is The Narrator In The Things They Carried
May 23, 2025
Related Post
Thank you for visiting our website which covers about Which Of The Following Is An Example Of A Parameter . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.