Which Of The Following Is An Example Of A Function
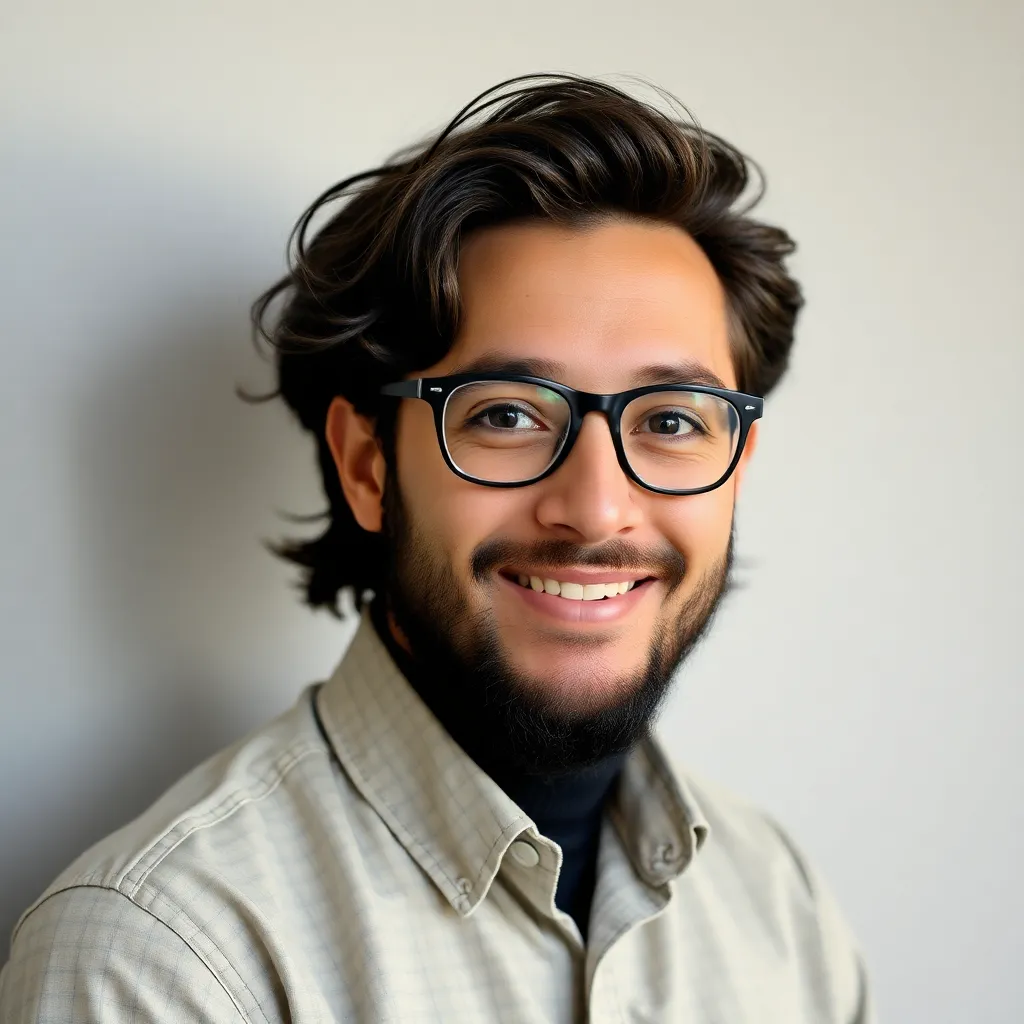
Juapaving
May 11, 2025 · 6 min read
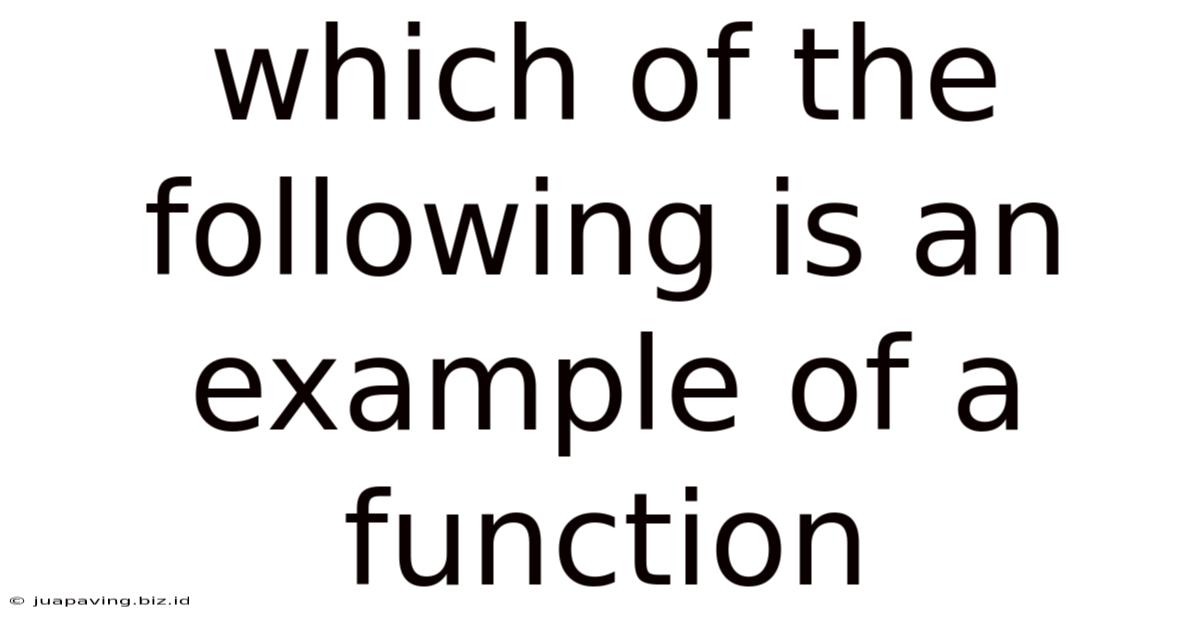
Table of Contents
Which of the Following is an Example of a Function? A Deep Dive into Functional Programming
Understanding functions is crucial in programming. This comprehensive guide will explore what constitutes a function, differentiating it from other programming constructs. We'll dissect various examples, clarifying the key characteristics that define a function and highlighting the practical implications of using them effectively. By the end, you’ll be able to confidently identify functions and appreciate their role in building robust and efficient programs.
What is a Function?
A function in programming is a self-contained block of code that performs a specific task. It’s a fundamental building block of structured programming, promoting modularity, reusability, and readability. Think of a function as a mini-program within a larger program. It receives input (arguments or parameters), processes that input, and returns an output (a result). This input-process-output model is the essence of a function's operation.
Key Characteristics of a Function:
- Input (Arguments/Parameters): A function can accept zero or more inputs, allowing it to operate on different data. These inputs are specified when the function is called.
- Process: The core logic of the function resides in its body. This is where the input data is manipulated or processed according to the function's purpose.
- Output (Return Value): A function typically produces a result, which is then returned to the part of the program that called it. While some functions might not explicitly return a value (often called
void
functions), they still perform an action. - Name: Each function is given a descriptive name that reflects its purpose. This makes the code easier to understand and maintain.
- Reusability: Once defined, a function can be called multiple times from different parts of the program, avoiding code duplication and improving efficiency.
- Modularity: Functions break down complex tasks into smaller, manageable units, making the overall program easier to design, debug, and modify.
Examples of Functions (and what's NOT a function)
Let's analyze various code snippets to illustrate what constitutes a function and what doesn't. We'll use Python for its clarity and widespread use, but the concepts apply broadly across programming languages.
Example 1: A Simple Function
def add_numbers(x, y):
"""This function adds two numbers and returns the sum."""
sum = x + y
return sum
result = add_numbers(5, 3) # Calling the function
print(result) # Output: 8
This is a clear example of a function. It takes two arguments (x
and y
), adds them, and returns the sum. The def
keyword defines the function, followed by its name, parameters, and the function body. The return
statement specifies the output.
Example 2: A Function with No Return Value
def greet(name):
"""This function greets the user by name."""
print(f"Hello, {name}!")
greet("Alice") # Output: Hello, Alice!
This function doesn't explicitly return a value (it's a void
function). Its purpose is to perform an action—printing a greeting—rather than calculating and returning a result. However, it still fits the definition of a function because it takes input and performs a specific task.
Example 3: A Function with a Conditional Statement
def is_even(number):
"""This function checks if a number is even."""
if number % 2 == 0:
return True
else:
return False
print(is_even(4)) # Output: True
print(is_even(7)) # Output: False
This function demonstrates the use of conditional logic within a function. It takes a number as input and returns True
if it's even and False
otherwise. The conditional statement (if-else
) is part of the function's internal processing.
Example 4: What is NOT a Function
x = 10
y = 5
sum = x + y
print(sum) # Output: 15
This code snippet performs an addition but doesn't define a function. It's a sequence of instructions executed linearly. There's no reusable block of code that can be called repeatedly with different inputs. It lacks the defining characteristics of a function: encapsulation, reusability, and modularity.
Example 5: A Function with Multiple Return Statements
def check_number(number):
"""Checks if a number is positive, negative, or zero."""
if number > 0:
return "Positive"
elif number < 0:
return "Negative"
else:
return "Zero"
print(check_number(5)) # Output: Positive
print(check_number(-3)) # Output: Negative
print(check_number(0)) # Output: Zero
This showcases a function utilizing multiple return
statements based on different conditions. This is perfectly acceptable and often enhances the readability and efficiency of functions by allowing early exits from the function once the result is determined.
Example 6: A Function with Default Argument Values
def greet_with_message(name, message="Hello"):
"""Greets the user with a customizable message."""
print(f"{message}, {name}!")
greet_with_message("Bob") # Output: Hello, Bob!
greet_with_message("Alice", "Good morning") # Output: Good morning, Alice!
This demonstrates the use of default argument values. If the message
argument isn't provided when calling the function, it defaults to "Hello". This enhances flexibility and reduces the number of function overloads needed for slightly varied functionality.
Advanced Function Concepts
1. Lambda Functions (Anonymous Functions):
Many languages support lambda functions, which are small, anonymous functions often used for short, simple operations. They are particularly useful with functional programming paradigms.
add = lambda x, y: x + y
print(add(2, 3)) # Output: 5
2. Higher-Order Functions:
Higher-order functions are functions that take other functions as arguments or return functions as results. They are powerful tools for abstracting and manipulating functions.
def apply_function(func, x):
return func(x)
def square(x):
return x * x
result = apply_function(square, 5)
print(result) # Output: 25
3. Recursion:
A function can call itself, a technique known as recursion. This is useful for solving problems that can be broken down into smaller, self-similar subproblems. However, it's crucial to have a base case to prevent infinite recursion.
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(5)) # Output: 120
4. Scope and Lifetime of Variables:
Variables declared within a function have local scope, meaning they are only accessible within that function. This promotes encapsulation and prevents naming conflicts. Variables declared outside functions have global scope. Understanding scope is critical for writing correct and maintainable code.
The Importance of Functions in Programming
Functions are more than just convenient code blocks. They are essential for:
- Improved Code Readability and Maintainability: Functions break down complex tasks into smaller, more understandable units. This makes the code easier to read, debug, and modify.
- Enhanced Reusability: Functions can be called multiple times from different parts of the program, eliminating code duplication and improving efficiency.
- Increased Modularity: Functions promote modular programming, where the program is organized as a collection of independent modules (functions). This improves code organization and allows for easier collaboration on larger projects.
- Better Code Organization: Functions contribute to a structured and organized codebase, improving overall software quality.
- Support for Advanced Programming Paradigms: Functions are fundamental to functional programming, a programming paradigm that emphasizes the use of functions as the primary building blocks of programs.
Conclusion
Identifying functions hinges on recognizing their core characteristics: input, process, and output (or action). Understanding these characteristics, as illustrated through the diverse examples above, empowers you to write cleaner, more efficient, and maintainable code. Mastering functions is a crucial step in your journey to becoming a proficient programmer. Remember to choose descriptive names, keep functions concise and focused, and leverage the power of higher-order functions and other advanced techniques to build truly robust and scalable software.
Latest Posts
Latest Posts
-
What Are Two Types Of Interference
May 12, 2025
-
Is A Rhombus A Regular Polygon
May 12, 2025
-
200 Mg Equals How Many Grams
May 12, 2025
-
What Is The Hybridization Of Co2
May 12, 2025
-
In Which Layer Of The Atmosphere Does Weather Take Place
May 12, 2025
Related Post
Thank you for visiting our website which covers about Which Of The Following Is An Example Of A Function . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.