The Unit Of Work Is The
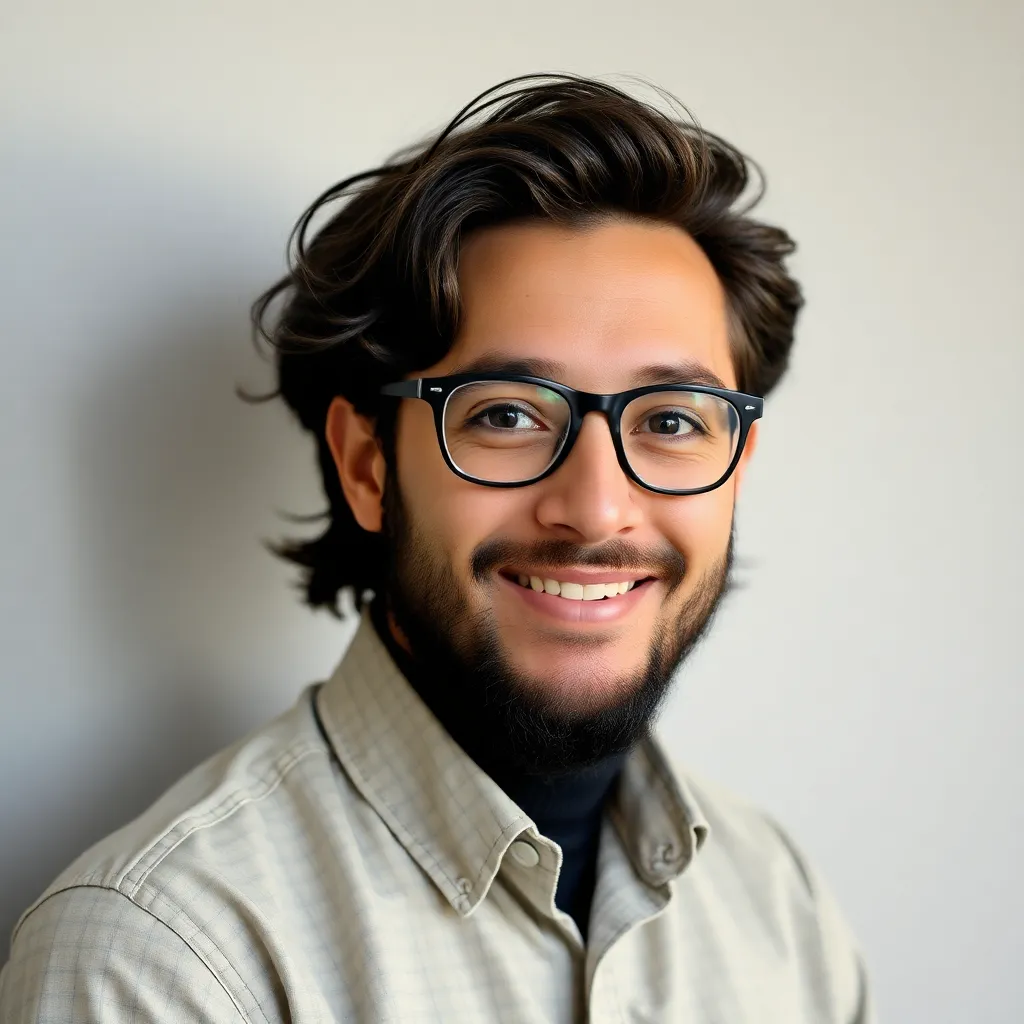
Juapaving
Apr 21, 2025 · 6 min read

Table of Contents
The Unit of Work Is the Key to Maintainable and Scalable Applications
The "Unit of Work" pattern is a cornerstone of robust and maintainable software design. While seemingly simple at first glance, its implications ripple throughout the architecture of an application, influencing aspects ranging from database interactions to error handling and concurrency. Understanding and effectively implementing the Unit of Work pattern is crucial for building scalable, testable, and ultimately successful applications. This comprehensive guide dives deep into the nuances of the Unit of Work, exploring its benefits, practical implementation, and common pitfalls.
What is the Unit of Work Pattern?
The Unit of Work pattern encapsulates a series of operations within a transaction boundary. Imagine a banking application where you need to transfer money between two accounts. This involves several steps: retrieving account balances, deducting from one account, and adding to the other. These operations are inherently related; if one fails, the entire transaction should roll back to maintain data integrity. The Unit of Work acts as the orchestrator, ensuring all these steps are treated as a single atomic unit.
In essence, the Unit of Work acts as a transaction manager for your application's data access layer. It ensures data consistency across multiple database operations, simplifies error handling, and improves performance by reducing round trips to the database.
Key Characteristics of a Unit of Work:
- Transaction Management: The core functionality is managing database transactions. It ensures either all operations within a unit succeed or none do, maintaining data integrity.
- Abstraction: It hides the complexities of database interaction from the application logic. The application interacts with the Unit of Work, not directly with the database.
- Atomic Operations: The unit treats multiple operations as a single atomic unit, guaranteeing consistency.
- Error Handling: It simplifies error handling by providing a single point of failure detection and rollback.
Benefits of Using the Unit of Work Pattern
Implementing the Unit of Work pattern offers a myriad of advantages, contributing significantly to the overall quality and maintainability of your application:
- Data Consistency: The most significant benefit is maintaining data integrity. By grouping related operations within a transaction, the Unit of Work prevents partial updates and ensures consistency even in the face of errors.
- Simplified Error Handling: Instead of managing transaction rollback across multiple database calls, the Unit of Work handles it centrally, leading to cleaner and more maintainable code.
- Improved Performance: By batching database operations, the Unit of Work can reduce the number of round trips to the database, significantly improving performance, especially with high-volume transactions.
- Testability: The abstraction provided by the Unit of Work facilitates easier unit testing. You can mock the Unit of Work and simulate database interactions without needing an actual database connection.
- Code Reusability: Once implemented, the Unit of Work can be reused across different parts of your application, promoting consistency and reducing code duplication.
- Enhanced Maintainability: The clear separation of concerns offered by the Unit of Work leads to more modular and maintainable code. Changes in the data access layer are less likely to affect other parts of the application.
Implementing the Unit of Work Pattern
The implementation of the Unit of Work can vary depending on the technology stack. However, the underlying principles remain consistent. Here's a conceptual example illustrating the key components:
public interface IUnitOfWork : IDisposable
{
IRepository Repository() where T : class;
int Complete(); // Saves changes and commits the transaction
}
public class UnitOfWork : IUnitOfWork
{
private readonly DbContext _context;
public UnitOfWork(DbContext context)
{
_context = context;
}
public IRepository Repository() where T : class
{
return new Repository(_context);
}
public int Complete()
{
return _context.SaveChanges(); // Commits the transaction
}
public void Dispose()
{
_context.Dispose();
}
}
public interface IRepository where T : class
{
void Add(T entity);
void Remove(T entity);
void Update(T entity);
// ... other repository methods ...
}
public class Repository : IRepository where T : class
{
private readonly DbContext _context;
private DbSet _dbSet;
public Repository(DbContext context)
{
_context = context;
_dbSet = context.Set();
}
// Implementation of Add, Remove, Update methods using _dbSet and _context
}
This example showcases a basic structure using a repository pattern. The UnitOfWork
class manages the DbContext
(or equivalent in your framework) and provides access to repositories. The Complete()
method saves changes and commits the transaction. The Dispose()
method ensures proper resource cleanup.
Note: The specific implementation details will vary depending on your chosen ORM (Object-Relational Mapper) and database technology. For instance, you might use different transaction management mechanisms based on your database system. You'll also need to consider handling exceptions appropriately within the Complete()
method to ensure proper rollback on failure.
Advanced Considerations
While the basic implementation is straightforward, several advanced considerations can enhance the robustness and scalability of your Unit of Work:
- Nested Unit of Work: In complex scenarios, you might need nested Unit of Work instances. Careful management is crucial to avoid conflicts and maintain data consistency.
- Distributed Transactions: For applications spanning multiple databases or services, you'll need mechanisms for distributed transactions to guarantee atomicity across different systems.
- Concurrency Control: Implementing appropriate concurrency control mechanisms (e.g., optimistic or pessimistic locking) is critical to handle concurrent access to data.
- Exception Handling: Robust exception handling is paramount. The Unit of Work should gracefully handle exceptions, ensuring proper rollback and informative error messages.
- Performance Optimization: Techniques like batching updates or using connection pooling can optimize database interactions and improve performance.
Common Pitfalls to Avoid
Several common pitfalls can undermine the effectiveness of the Unit of Work pattern:
- Ignoring Transaction Boundaries: Failing to define clear transaction boundaries can lead to data inconsistency and errors.
- Inappropriate Use of Nested Units of Work: Improperly managing nested Unit of Work instances can result in unexpected behavior and deadlocks.
- Insufficient Error Handling: Lack of robust exception handling can leave your application vulnerable to data corruption and failures.
- Ignoring Concurrency: Not considering concurrency issues can lead to data inconsistencies and race conditions.
- Overuse or Underuse: The Unit of Work pattern is not a one-size-fits-all solution. Using it inappropriately (e.g., for single database operations) can add unnecessary complexity. Conversely, not using it for operations requiring atomicity can lead to data inconsistencies.
Conclusion
The Unit of Work pattern is a powerful tool for building maintainable, scalable, and robust applications. By encapsulating database operations within transaction boundaries, it ensures data consistency, simplifies error handling, and improves overall performance. While the implementation might involve some initial setup, the long-term benefits far outweigh the effort. Understanding the nuances of the pattern, including advanced considerations and potential pitfalls, is crucial for successful implementation and leveraging its full potential. Remember to tailor the implementation to your specific needs and technology stack, always prioritizing data integrity and application stability. By mastering the Unit of Work pattern, you take a significant step toward building high-quality, dependable applications.
Latest Posts
Latest Posts
-
1 2 3 1 2 3
Apr 21, 2025
-
Zinc Nitrate Crystals Are Strongly Heated
Apr 21, 2025
-
Standard Form Expanded Form And Word Form
Apr 21, 2025
-
1 5 Rounded To The Nearest Tenth
Apr 21, 2025
-
Angle Of Incidence Equals Angle Of Reflection
Apr 21, 2025
Related Post
Thank you for visiting our website which covers about The Unit Of Work Is The . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.