Order Values From Least To Greatest
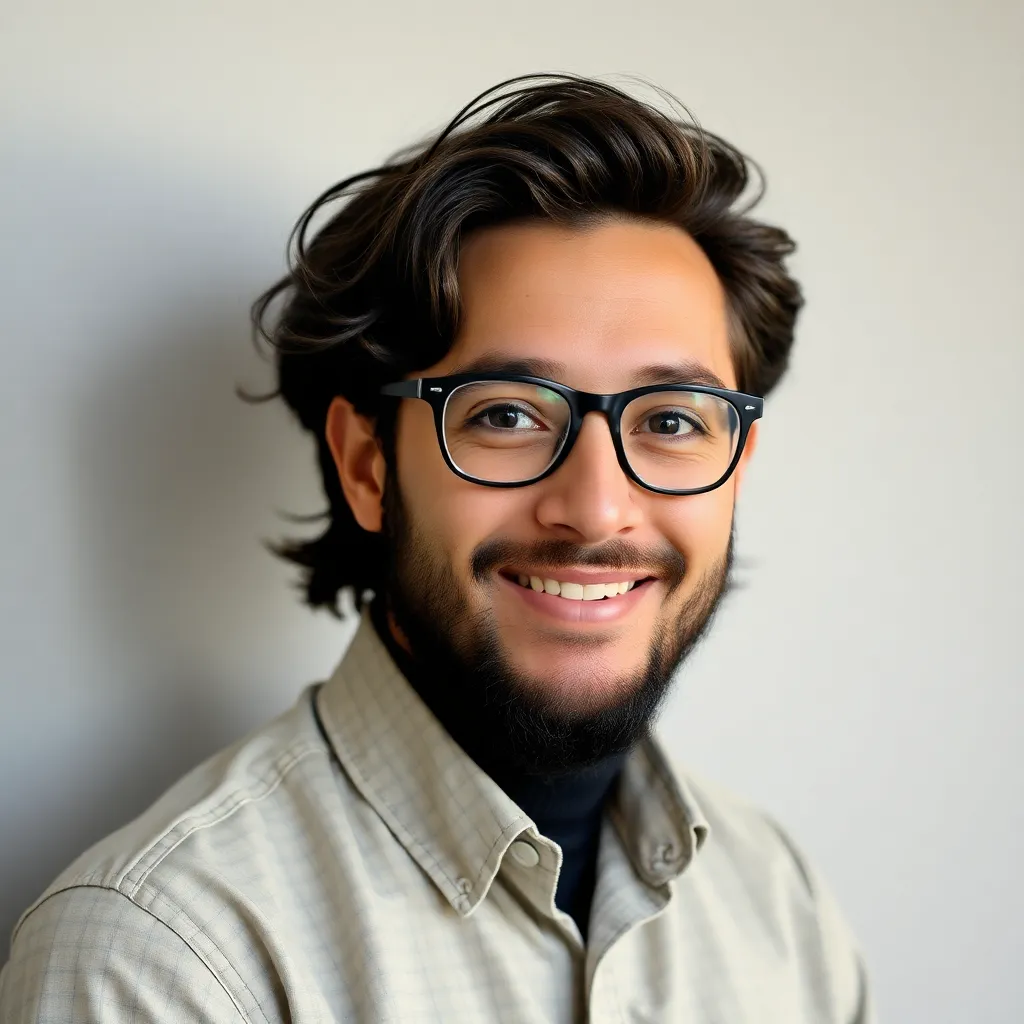
Juapaving
Apr 09, 2025 · 7 min read

Table of Contents
Ordering Values from Least to Greatest: A Comprehensive Guide
Ordering values, whether they are numbers, dates, or even alphabetical strings, is a fundamental skill with applications spanning numerous fields. From simple everyday tasks to complex data analysis, the ability to effectively arrange data from least to greatest (or ascending order) is crucial. This comprehensive guide delves into various methods and scenarios for ordering values, focusing on efficiency and understanding the underlying principles.
Understanding Ordering Principles
Before diving into specific techniques, let's establish a clear understanding of the core principles behind ordering values. The objective is to arrange a set of values in a sequence where each subsequent value is greater than or equal to its predecessor. This seemingly simple concept holds significant implications across diverse domains.
Numerical Ordering: The Foundation
The most basic form of ordering involves numerical values. This is intuitive: we naturally compare numbers based on their magnitude. For instance, arranging the set {5, 2, 9, 1, 7} from least to greatest results in {1, 2, 5, 7, 9}. This principle extends to integers, decimals, fractions, and even scientific notation.
Alphabetical Ordering: String Comparison
Alphabetical ordering is another common form of ordering, particularly useful for textual data. This relies on the lexicographical order of characters within a given alphabet. For example, arranging the set {"banana", "apple", "cherry", "date"} alphabetically yields {"apple", "banana", "cherry", "date"}. The comparison happens character by character, and the first difference determines the order.
Date and Time Ordering: Chronological Sequence
Ordering dates and times follows a chronological sequence, from earliest to latest. This requires careful consideration of the date and time format. For instance, "2023-10-26" precedes "2024-01-15". The year, month, and day are compared sequentially to determine the correct order. Similarly, time values, including hours, minutes, and seconds, are ordered chronologically.
Custom Ordering: Defining Your Own Rules
In more complex scenarios, you might need to define custom ordering rules. This could involve prioritizing specific attributes or applying specialized comparison logic. For example, ordering a list of products based on a combination of price and popularity would require a custom ordering function that takes both factors into account.
Methods for Ordering Values
Several methods can be employed for ordering values, ranging from manual sorting for small datasets to sophisticated algorithms for larger datasets.
Manual Sorting: Suitable for Small Sets
For small sets of values (a few items), manual sorting is a straightforward and efficient method. Simply visually inspect the values and arrange them in the desired order. This method is intuitive and requires no specialized tools or knowledge. However, it becomes impractical for larger datasets due to its time-consuming nature and increased risk of errors.
Bubble Sort: A Simple but Inefficient Algorithm
Bubble sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order. The pass through the list is repeated until no swaps are needed, which indicates that the list is sorted. While easy to understand, bubble sort is inefficient for large datasets, having a time complexity of O(n²).
Example (Python):
def bubble_sort(list_):
n = len(list_)
for i in range(n-1):
for j in range(n-i-1):
if list_[j] > list_[j+1]:
list_[j], list_[j+1] = list_[j+1], list_[j]
return list_
my_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(my_list)
print("Sorted array:", sorted_list)
Insertion Sort: Efficient for Small to Moderately Sized Lists
Insertion sort builds the final sorted array one item at a time. It is much more efficient than bubble sort for small to moderately sized lists. It works by taking elements from the unsorted portion and inserting them into their correct position within the sorted portion. The time complexity of insertion sort is O(n²) in the worst case, but it performs better in practice for nearly sorted lists.
Example (Python):
def insertion_sort(list_):
for i in range(1, len(list_)):
key = list_[i]
j = i - 1
while j >= 0 and key < list_[j]:
list_[j + 1] = list_[j]
j -= 1
list_[j + 1] = key
return list_
my_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = insertion_sort(my_list)
print("Sorted array:", sorted_list)
Merge Sort: A Highly Efficient Algorithm
Merge sort is a divide-and-conquer algorithm that recursively divides the list into smaller sublists until each sublist contains only one element. Then, it repeatedly merges the sublists to produce new sorted sublists until there is only one sorted list remaining. Merge sort has a time complexity of O(n log n), making it highly efficient for large datasets.
Quick Sort: Another Efficient Algorithm
Quick sort is another divide-and-conquer algorithm that works by selecting a 'pivot' element and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted. Quick sort also has an average time complexity of O(n log n), but its worst-case time complexity can be O(n²).
Ordering Values in Different Contexts
The methods for ordering values adapt to the specific context and data type.
Ordering Numbers in Spreadsheets
Spreadsheets like Microsoft Excel or Google Sheets provide built-in sorting functionality. Simply select the column containing the numbers, and use the sorting option to arrange them from least to greatest. This is highly convenient for tabular data.
Ordering Data in Programming Languages
Most programming languages offer built-in sorting functions or methods. These functions often use optimized algorithms like merge sort or quicksort to efficiently order large datasets. For example, Python's list.sort()
method and the sorted()
function are widely used for sorting lists.
Ordering Strings in Databases
Databases also have efficient mechanisms for ordering data, often using specialized indexing structures to speed up the process. SQL queries frequently employ the ORDER BY
clause to specify the sorting criteria.
Ordering Complex Data Structures
Ordering more complex data structures, such as objects or custom data types, requires defining a comparison function or operator that specifies how the objects should be compared. This function dictates the ordering based on the relevant attributes of the objects.
Advanced Ordering Techniques
For very large datasets or scenarios with specific requirements, more advanced techniques may be necessary.
Radix Sort: Efficient for Integers and Strings
Radix sort is a non-comparison-based sorting algorithm that works by sorting numbers or strings digit by digit or character by character, starting from the least significant digit or character. It's particularly efficient for integers and strings with a fixed length.
Counting Sort: Efficient for Integers with a Limited Range
Counting sort is another non-comparison-based algorithm that operates by counting the occurrences of each unique element in the input array. It then uses this information to construct the output array in sorted order. This algorithm is very efficient for integers within a known range, but it is not suitable for floating-point numbers or large ranges.
External Sorting: Handling Datasets Larger Than Memory
For datasets too large to fit into computer memory, external sorting techniques are employed. These algorithms involve reading portions of the dataset from secondary storage (like a hard drive), sorting them in memory, and then merging the sorted portions.
Conclusion
Ordering values is a fundamental operation with applications throughout various fields. Choosing the appropriate method depends on factors such as the dataset size, data type, and desired efficiency. Understanding the underlying principles and algorithms empowers you to efficiently manage and analyze data, extracting meaningful insights and making informed decisions. Whether manually sorting a small list or implementing a sophisticated algorithm for large-scale data processing, mastering the art of ordering values is essential for effective data handling. By leveraging the right techniques and tools, you can transform raw data into organized, easily interpretable information.
Latest Posts
Latest Posts
-
Image Of A Plant Cell With Labels
Apr 17, 2025
-
Prevents Backflow Into The Left Atrium
Apr 17, 2025
-
What Is The Least Common Multiple For 12 And 18
Apr 17, 2025
-
Animals That Come Up For Air
Apr 17, 2025
-
Lab Mitosis In Onion Root Tip
Apr 17, 2025
Related Post
Thank you for visiting our website which covers about Order Values From Least To Greatest . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.