Input And Output In C Programming
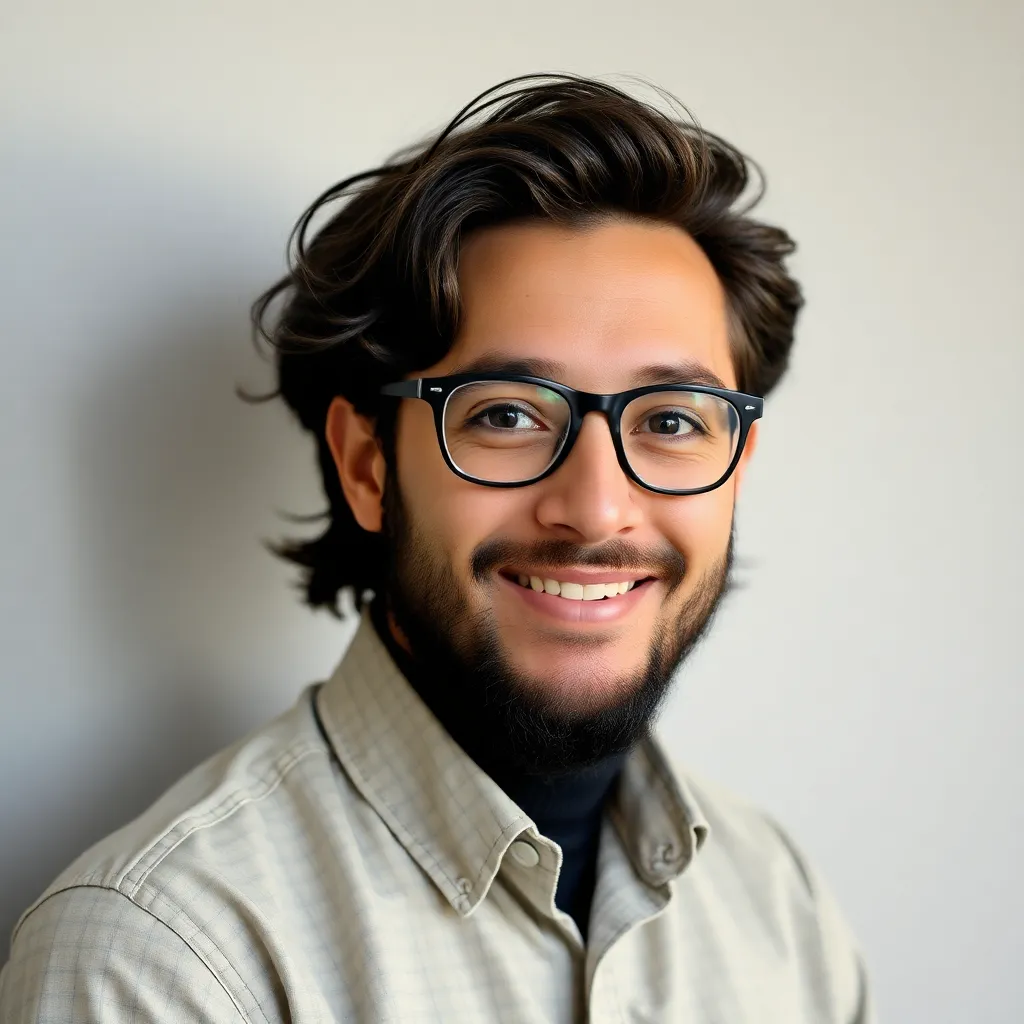
Juapaving
Apr 15, 2025 · 6 min read

Table of Contents
Input and Output in C Programming: A Comprehensive Guide
C programming, despite its age, remains a cornerstone of software development. Understanding its input and output (I/O) mechanisms is crucial for any aspiring C programmer. This comprehensive guide will delve into the intricacies of C I/O, covering both standard I/O using stdio.h
and more advanced techniques. We'll explore various functions, their functionalities, and best practices for effective and efficient I/O operations.
Understanding Standard Input and Output
The standard I/O library, declared using #include <stdio.h>
, provides a set of functions for handling input and output operations. These functions interact with standard streams:
stdin
(standard input): Typically connected to the keyboard. Data is read fromstdin
.stdout
(standard output): Typically connected to the console screen. Data is written tostdout
.stderr
(standard error): Also connected to the console screen, but used for error messages. This allows for separating program output from error reports.
These streams enable a simple and consistent way to interact with the user and display program results.
Functions for Standard Output
The most common function for standard output is printf()
. It's a versatile function capable of formatting output in various ways.
printf()
Function:
The printf()
function takes a format string as its first argument and then a variable number of additional arguments. The format string specifies how the subsequent arguments should be formatted and displayed.
#include
int main() {
int age = 30;
float height = 1.75;
char name[] = "John Doe";
printf("My name is %s, I am %d years old and %f meters tall.\n", name, age, height);
return 0;
}
Format Specifiers:
printf()
uses format specifiers (e.g., %d
, %f
, %s
, %c
) to indicate the type of data to be printed. Key format specifiers include:
%d
or%i
: Integer%u
: Unsigned integer%f
: Floating-point number%e
or%E
: Scientific notation%g
or%G
: Chooses between%f
and%e
based on magnitude%c
: Character%s
: String%%
: Prints a literal%
sign
puts()
Function:
The puts()
function is simpler than printf()
. It writes a string to stdout
, followed by a newline character (\n
).
#include
int main() {
puts("Hello, world!");
return 0;
}
putchar()
Function:
putchar()
writes a single character to stdout
.
#include
int main() {
putchar('A');
putchar('\n'); // Add a newline for better readability.
return 0;
}
Functions for Standard Input
The primary function for standard input is scanf()
. It's used to read formatted input from stdin
.
scanf()
Function:
Similar to printf()
, scanf()
uses a format string to specify the expected data types. It then reads the input accordingly and stores it in the provided variables.
#include
int main() {
int age;
float salary;
char name[50];
printf("Enter your name: ");
scanf("%s", name); // Note: No & is needed for strings (char arrays)
printf("Enter your age: ");
scanf("%d", &age); // & is crucial for integer variables
printf("Enter your salary: ");
scanf("%f", &salary);
printf("Name: %s, Age: %d, Salary: %.2f\n", name, age, salary);
return 0;
}
Important Note on scanf()
: scanf()
can be tricky to use correctly, especially when dealing with spaces in input. It's crucial to understand how it parses the input string based on the format specifiers. For more robust input handling, consider using fgets()
(explained later).
getchar()
Function:
getchar()
reads a single character from stdin
. It's often used for character-by-character input.
#include
int main() {
char ch;
printf("Enter a character: ");
ch = getchar();
printf("You entered: %c\n", ch);
return 0;
}
File Input and Output
Beyond standard I/O, C allows for file I/O, enabling programs to read and write data to files. This is essential for persistent data storage.
File Handling Functions
The key functions for file I/O are:
-
fopen()
: Opens a file. Takes the filename and mode as arguments. Modes include:"r"
: Read mode (file must exist)"w"
: Write mode (creates a new file or truncates an existing one)"a"
: Append mode (adds data to the end of an existing file)"r+"
: Read and write mode (file must exist)"w+"
: Read and write mode (creates a new file or truncates an existing one)"a+"
: Read and append mode (adds data to the end of an existing file)
-
fclose()
: Closes a file. Important to release resources and ensure data is written to disk. -
fprintf()
: Writes formatted data to a file. Similar toprintf()
, but takes a file pointer as the first argument. -
fscanf()
: Reads formatted data from a file. Similar toscanf()
, but takes a file pointer as the first argument. -
fgetc()
: Reads a single character from a file. -
fputc()
: Writes a single character to a file. -
fgets()
: Reads a line of text from a file, including the newline character. Generally safer and more robust thanfscanf()
for string input. -
fputs()
: Writes a string to a file.
Example: Reading from a File
#include
int main() {
FILE *fp;
char line[255];
fp = fopen("my_file.txt", "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
while (fgets(line, sizeof(line), fp) != NULL) {
printf("%s", line);
}
fclose(fp);
return 0;
}
Example: Writing to a File
#include
int main() {
FILE *fp;
fp = fopen("my_output.txt", "w");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
fprintf(fp, "This is some text to write to the file.\n");
fprintf(fp, "Another line of text.\n");
fclose(fp);
return 0;
}
Error Handling in I/O
Proper error handling is crucial for robust C programs. Always check the return values of I/O functions. For example, fopen()
returns NULL
if the file cannot be opened. fscanf()
and scanf()
return the number of items successfully read. Checking these return values allows you to detect and handle errors gracefully, preventing unexpected program behavior.
The perror()
function is useful for displaying system error messages. It takes a string as an argument that describes the error.
Advanced I/O Techniques
Beyond standard and file I/O, C offers more advanced techniques:
-
Binary I/O: Allows for reading and writing data in its binary format, often more efficient than text-based I/O. Functions like
fread()
andfwrite()
are used for binary I/O. -
Low-level I/O: This involves direct interaction with operating system functionalities, offering more control but increased complexity. Functions like
read()
andwrite()
are examples of low-level I/O. -
Memory Mapping: Allows a portion of a file to be mapped directly into memory, enabling efficient random access.
-
Networking I/O: C provides libraries (like
sockets
) for network programming, enabling communication between different systems.
Best Practices for C I/O
- Always check for errors: Verify the return values of I/O functions.
- Close files when finished: Use
fclose()
to release file resources. - Use appropriate functions: Choose the right function for the task (e.g.,
fgets()
overscanf()
for string input from files). - Handle memory carefully: Allocate and deallocate memory appropriately, especially when dealing with large files or dynamic data structures.
- Consider buffering: For large amounts of data, buffering can improve performance.
Conclusion
Mastering input and output in C programming is foundational to building effective and robust applications. By understanding the standard I/O functions, file handling techniques, and error-handling strategies, you can create C programs that interact seamlessly with users and manage data efficiently. This guide provides a comprehensive overview, equipping you with the knowledge to handle various I/O scenarios and build sophisticated C applications. Remember to practice and experiment to solidify your understanding. The more you work with C I/O, the more proficient you will become.
Latest Posts
Latest Posts
-
What Organelles Are Only Found In Plant Cells
Apr 15, 2025
-
Why Do Conductors Have A High Heat Capacity
Apr 15, 2025
-
Which Of The Following Diseases Are Caused By A Virus
Apr 15, 2025
-
Which Of The Following Is Not A Function Of Lysosomes
Apr 15, 2025
-
Pressure Is Measured In What Units
Apr 15, 2025
Related Post
Thank you for visiting our website which covers about Input And Output In C Programming . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.