How To Find The Gcd Of Three Numbers
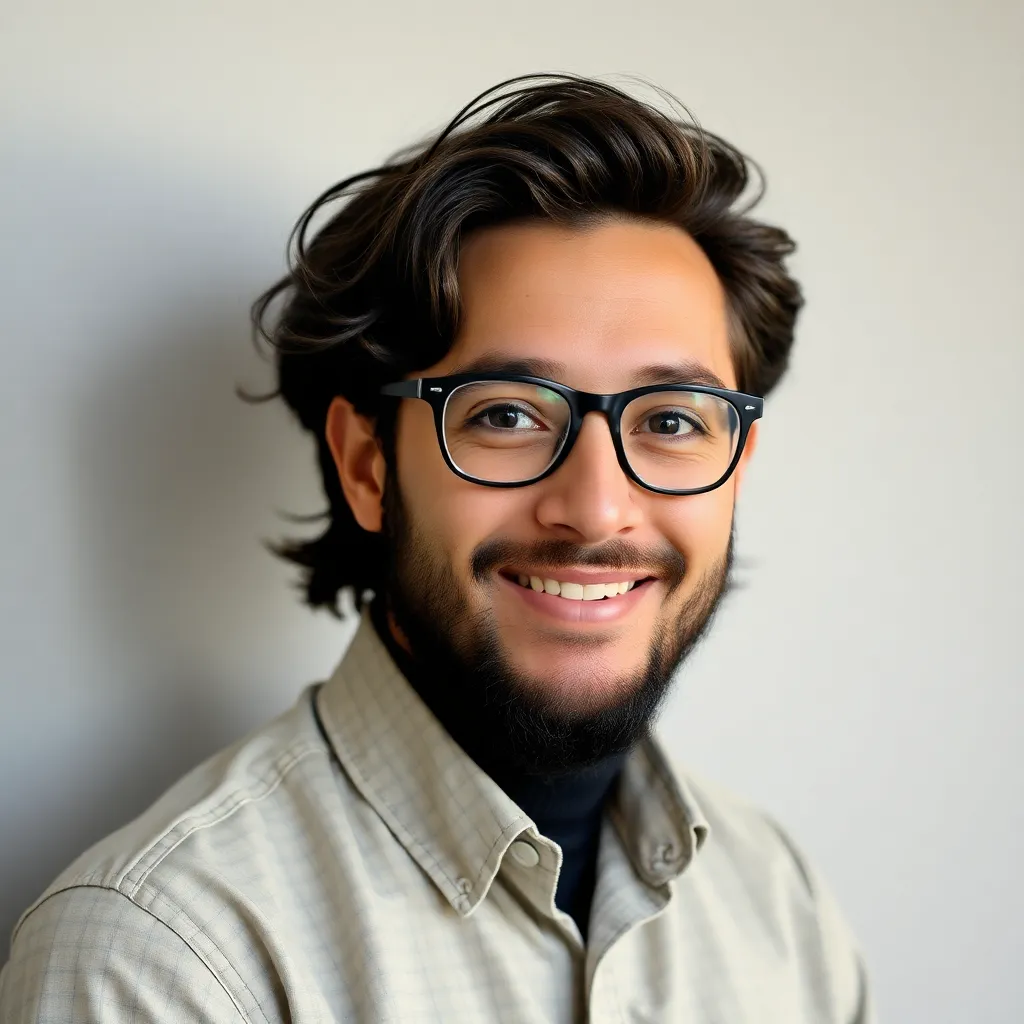
Juapaving
Mar 18, 2025 · 6 min read
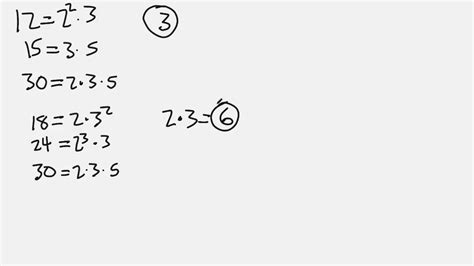
Table of Contents
How to Find the Greatest Common Divisor (GCD) of Three Numbers
Finding the greatest common divisor (GCD) of three or more numbers is a fundamental concept in number theory with applications in various fields, from cryptography to computer science. While finding the GCD of two numbers is relatively straightforward using the Euclidean algorithm, extending this to three or more numbers requires a systematic approach. This comprehensive guide will explore various methods to efficiently determine the GCD of three numbers, catering to different levels of mathematical understanding.
Understanding the Greatest Common Divisor (GCD)
Before diving into the methods, let's solidify our understanding of the GCD. The GCD of a set of numbers is the largest positive integer that divides all the numbers in the set without leaving a remainder. For example, the GCD of 12, 18, and 24 is 6 because 6 is the largest number that divides 12, 18, and 24 evenly.
Method 1: Repeated Application of the Euclidean Algorithm
This is perhaps the most intuitive method. Since the Euclidean algorithm efficiently finds the GCD of two numbers, we can apply it iteratively to find the GCD of three or more.
Steps:
-
Find the GCD of the first two numbers: Use the Euclidean algorithm (explained below) to find the GCD of the first two numbers in your set.
-
Find the GCD of the result and the third number: Take the GCD obtained in step 1 and find its GCD with the third number using the Euclidean algorithm again.
-
Repeat for more numbers: If you have more than three numbers, continue this process, using the GCD obtained in the previous step and the next number in the set.
The Euclidean Algorithm:
The Euclidean algorithm is a highly efficient method for finding the GCD of two numbers. It's based on the principle that the GCD of two numbers doesn't change if the larger number is replaced by its difference with the smaller number. This process is repeated until the two numbers are equal, which is the GCD.
Let's illustrate with an example: Find the GCD of 48 and 18.
- 48 = 2 * 18 + 12
- 18 = 1 * 12 + 6
- 12 = 2 * 6 + 0
The remainder is 0, so the GCD is the last non-zero remainder, which is 6.
Example with Three Numbers:
Let's find the GCD of 24, 36, and 60.
-
GCD(24, 36):
- 36 = 1 * 24 + 12
- 24 = 2 * 12 + 0
- GCD(24, 36) = 12
-
GCD(12, 60):
- 60 = 5 * 12 + 0
- GCD(12, 60) = 12
Therefore, the GCD(24, 36, 60) = 12.
Method 2: Prime Factorization
This method leverages the fundamental theorem of arithmetic, which states that every integer greater than 1 can be uniquely represented as a product of prime numbers.
Steps:
-
Find the prime factorization of each number: Decompose each number into its prime factors.
-
Identify common prime factors: Look for the prime factors that are common to all three numbers.
-
Calculate the GCD: Multiply the common prime factors raised to the lowest power they appear in any of the factorizations.
Example:
Find the GCD of 12, 18, and 30.
-
Prime Factorization:
- 12 = 2² * 3
- 18 = 2 * 3²
- 30 = 2 * 3 * 5
-
Common Prime Factors: The common prime factors are 2 and 3.
-
Calculate GCD: The lowest power of 2 is 2¹ and the lowest power of 3 is 3¹. Therefore, GCD(12, 18, 30) = 2 * 3 = 6.
Method 3: Using the gcd()
function in programming languages
Most programming languages, including Python, have built-in functions to calculate the GCD of two numbers. While these functions typically only handle two numbers at a time, we can still use them iteratively, just like in Method 1.
Python Example:
import math
def gcd_three_numbers(a, b, c):
"""Calculates the GCD of three numbers using the math.gcd() function."""
return math.gcd(math.gcd(a, b), c)
# Example usage
result = gcd_three_numbers(24, 36, 60)
print(f"The GCD of 24, 36, and 60 is: {result}")
This Python code efficiently calculates the GCD by repeatedly applying the math.gcd()
function. This approach is concise and leverages the optimized implementation within the language itself. Similar functions exist in other languages like Java (java.math.BigInteger.gcd()
), C++ (std::gcd()
), and JavaScript (math.gcd()
).
Choosing the Right Method
The best method for finding the GCD of three numbers depends on several factors:
-
Computational efficiency: For very large numbers, the Euclidean algorithm (Method 1) is generally the most efficient. Prime factorization (Method 2) can become computationally expensive for large numbers.
-
Ease of implementation: Using built-in functions (Method 3) is the easiest to implement if you're working within a programming environment.
-
Educational purpose: If the goal is to understand the underlying mathematical concepts, the prime factorization method can be more insightful.
Advanced Considerations and Applications
While the methods described above cover the basics, let's explore some advanced aspects and applications of finding the GCD of three or more numbers:
-
More than three numbers: The iterative application of the Euclidean algorithm (Method 1) extends seamlessly to any number of integers. Similarly, the prime factorization method can handle more numbers by finding the common prime factors among all of them.
-
Least Common Multiple (LCM): The GCD and LCM are closely related. The product of the GCD and LCM of two numbers is equal to the product of the two numbers. This relationship can be extended to more than two numbers, although the calculation becomes more involved.
-
Linear Diophantine Equations: The GCD plays a crucial role in solving linear Diophantine equations, which are equations of the form ax + by = c, where a, b, and c are integers, and x and y are integer variables. The equation has integer solutions if and only if c is divisible by the GCD(a, b).
-
Cryptography: The GCD is fundamental in various cryptographic algorithms, especially those based on modular arithmetic. The Euclidean algorithm is used extensively in these algorithms for tasks like key generation and encryption/decryption.
-
Computer Graphics: GCD calculations are utilized in computer graphics for tasks such as finding the greatest common divisor of pixel coordinates for texture mapping and image processing.
-
Music Theory: In music theory, GCDs are used to determine the greatest common divisor of note intervals, helping in understanding the relationships between different notes in a musical composition.
Conclusion
Finding the GCD of three numbers is a valuable skill with widespread applications. While the Euclidean algorithm provides a generally efficient and widely applicable solution, the prime factorization method offers a deeper understanding of the underlying mathematical principles. The choice of method depends on the context, the size of the numbers involved, and the computational resources available. Understanding these methods equips you with a powerful tool for various mathematical and computational tasks. Remember to choose the approach that best suits your needs and level of understanding, always prioritizing efficiency and clarity.
Latest Posts
Latest Posts
-
Round A Decimal To The Nearest Thousandth
Mar 18, 2025
-
Fraction As A Product Of A Whole Number
Mar 18, 2025
-
Moment Of Inertia For Right Triangle
Mar 18, 2025
-
How Many Electrons Can 3d Hold
Mar 18, 2025
-
Group 18 Elements Were Called The Noble Gases Originally Because
Mar 18, 2025
Related Post
Thank you for visiting our website which covers about How To Find The Gcd Of Three Numbers . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.