Difference Between Procedural And Object Oriented Programming
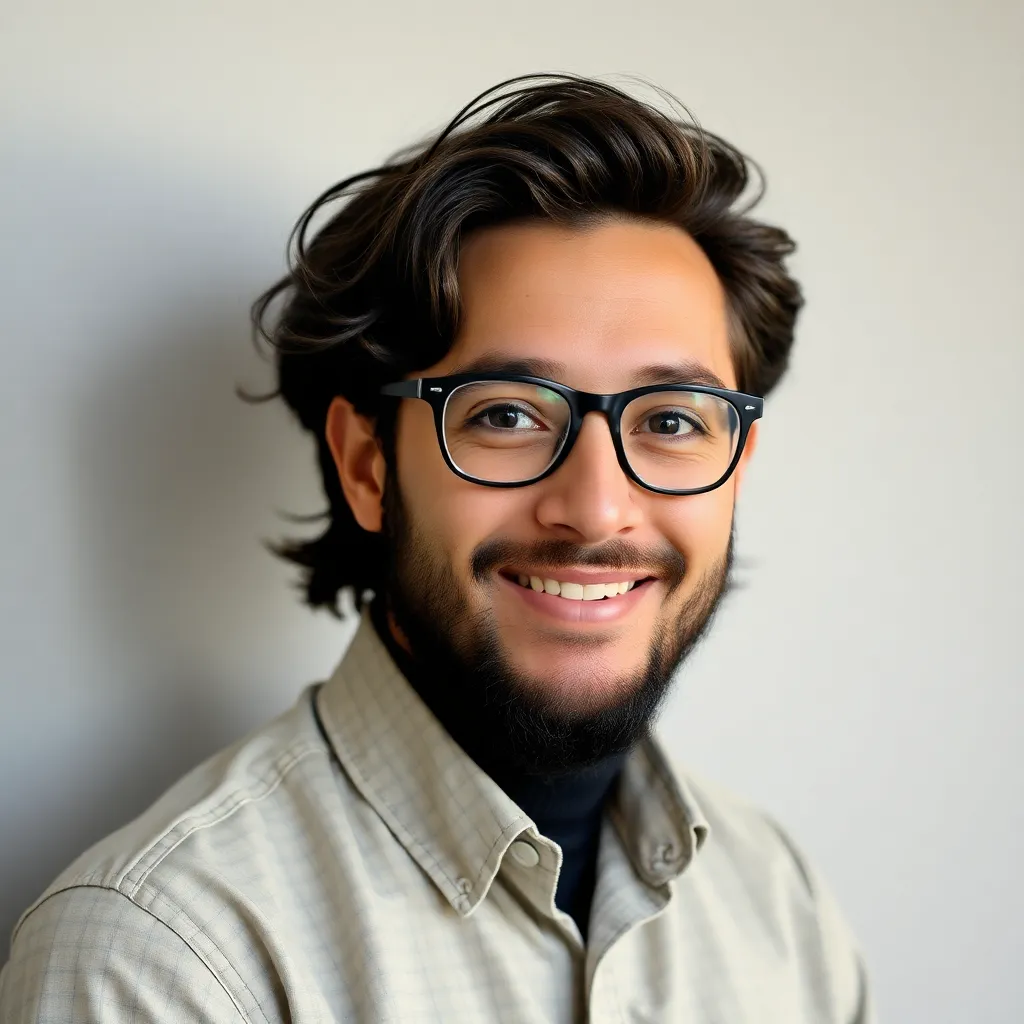
Juapaving
May 12, 2025 · 6 min read
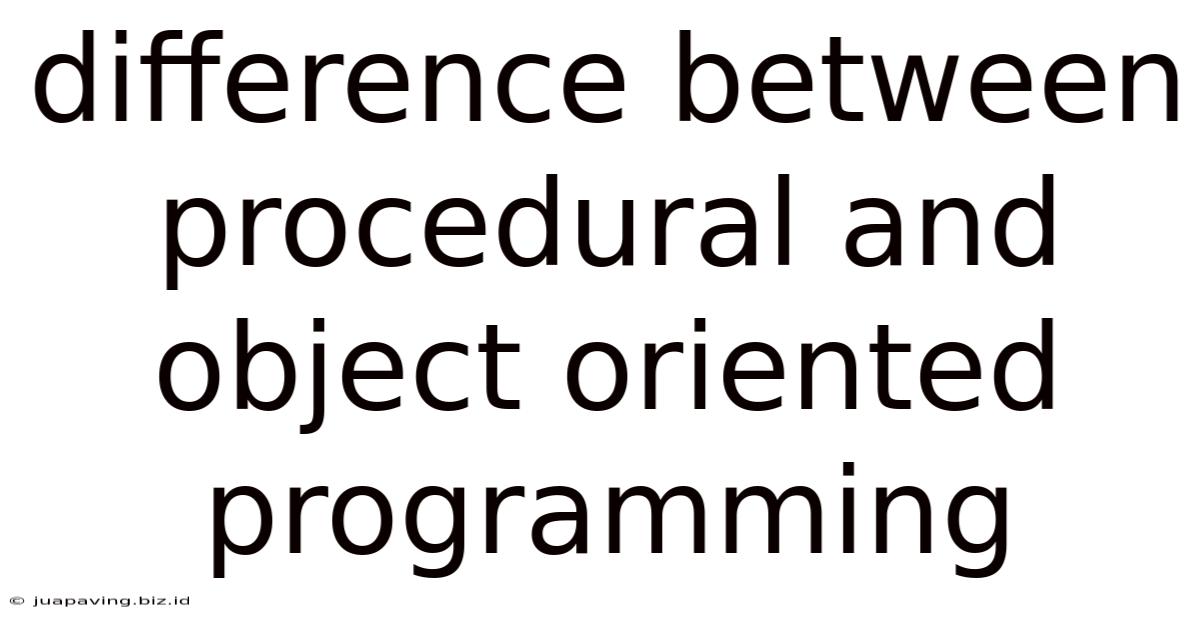
Table of Contents
Delving Deep into the Differences: Procedural vs. Object-Oriented Programming
The programming world is vast and varied, but at its core lie fundamental paradigms that shape how software is designed and built. Two of the most dominant paradigms are procedural programming and object-oriented programming (OOP). While both aim to solve problems through code, they approach the task with drastically different philosophies. Understanding the core differences between these paradigms is crucial for any aspiring programmer, enabling informed choices about which approach is best suited for a given project. This comprehensive guide will dissect the intricacies of procedural and object-oriented programming, highlighting their strengths, weaknesses, and ideal applications.
Procedural Programming: A Sequential Approach
Procedural programming, a cornerstone of early programming languages like C and Pascal, organizes code into a series of procedures or functions. These functions operate on data, often stored in global variables, to perform specific tasks. The program's execution follows a linear path, progressing from one procedure to the next in a predefined sequence.
Core Characteristics of Procedural Programming:
- Emphasis on Procedures: The core building blocks are procedures or functions, which perform specific operations.
- Global Data: Data is often stored in global variables, accessible from anywhere in the program. This can lead to issues with data integrity and maintainability.
- Sequential Execution: Program execution flows sequentially from one procedure to the next.
- Limited Data Abstraction: Data and functions are usually treated separately, leading to less encapsulation and potential issues with data security.
- Simple Structure: Relatively easier to learn and understand, especially for beginners.
Advantages of Procedural Programming:
- Simplicity: The straightforward, sequential nature makes it easier to understand and debug, particularly for smaller programs.
- Efficiency: For simple tasks, procedural programs can be very efficient as they avoid the overhead of complex object structures.
- Ease of Learning: The simpler structure makes it a good starting point for beginners in programming.
Disadvantages of Procedural Programming:
- Data Integrity Issues: The use of global variables can lead to unintended modification of data, making debugging difficult and hindering maintainability.
- Difficult to Maintain and Scale: As programs grow larger, maintaining and modifying the code becomes challenging due to the interconnected nature of procedures and global data.
- Lack of Reusability: Code reusability is limited; functions often need significant modification to be used in different contexts.
- Poor Data Security: Global data access allows any function to modify the data, compromising data security.
- Limited Modularity: Procedures are independent units, making it difficult to create highly modular and reusable code.
Example (Illustrative):
Let's consider a simple example of calculating the area of a rectangle using procedural programming:
#include
// Global variables storing rectangle dimensions
float length, width;
// Function to calculate the area
float calculateArea() {
return length * width;
}
int main() {
printf("Enter length: ");
scanf("%f", &length);
printf("Enter width: ");
scanf("%f", &width);
float area = calculateArea();
printf("Area: %.2f\n", area);
return 0;
}
This example demonstrates the use of global variables (length
, width
) and a function (calculateArea
) to perform a specific task.
Object-Oriented Programming: A Paradigm Shift
Object-oriented programming (OOP) represents a significant shift in programming philosophy. Instead of focusing on procedures, OOP centers around objects, which encapsulate both data (attributes) and the functions (methods) that operate on that data. This encapsulation fosters modularity, reusability, and improved data security.
Core Principles of Object-Oriented Programming:
- Abstraction: Hiding complex implementation details and exposing only essential information to the user.
- Encapsulation: Bundling data and methods that operate on that data within a single unit (the object), protecting the data from unauthorized access.
- Inheritance: Creating new classes (objects) based on existing ones, inheriting their attributes and methods. This promotes code reusability and reduces redundancy.
- Polymorphism: The ability of objects of different classes to respond to the same method call in their own specific way.
Advantages of Object-Oriented Programming:
- Modularity: Code is organized into reusable modules (objects), making it easier to maintain, update, and extend.
- Reusability: Inheritance allows for the creation of new objects based on existing ones, promoting code reuse.
- Data Security: Encapsulation protects data from unauthorized access, enhancing data integrity and security.
- Maintainability: The modular nature makes it easier to debug and maintain large, complex programs.
- Scalability: OOP designs are better suited for large-scale projects due to their modularity and reusability.
- Real-World Modeling: OOP's object-based approach allows for a more natural representation of real-world entities and their interactions.
Disadvantages of Object-Oriented Programming:
- Steeper Learning Curve: OOP concepts can be more challenging for beginners to grasp compared to procedural programming.
- Increased Complexity: OOP programs can be more complex than procedural programs, especially for simple tasks.
- Performance Overhead: The added overhead of object management can result in slightly slower execution speeds compared to procedural programs, although this difference is often negligible in modern systems.
- Over-Engineering: OOP can sometimes lead to over-engineering of simple problems, making the solution more complex than necessary.
Example (Illustrative):
Let's revisit the rectangle area calculation using OOP principles (using Python for clarity):
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def calculate_area(self):
return self.length * self.width
# Create a rectangle object
rectangle = Rectangle(5, 10)
# Calculate the area using the object's method
area = rectangle.calculate_area()
print(f"Area: {area}")
This example demonstrates encapsulation (data length
and width
are bundled with the method calculate_area
), and the creation of an object (rectangle
) that encapsulates both data and functionality.
Comparing Procedural and Object-Oriented Programming: A Head-to-Head
Feature | Procedural Programming | Object-Oriented Programming |
---|---|---|
Core Unit | Procedures/Functions | Objects |
Data Handling | Global variables, potential data integrity issues | Encapsulated within objects, better data security |
Modularity | Limited | High, through objects and classes |
Reusability | Low | High, through inheritance and polymorphism |
Maintainability | Difficult for large programs | Easier for large programs |
Scalability | Difficult to scale | Easier to scale |
Complexity | Simpler for small programs | More complex, especially for beginners |
Learning Curve | Easier to learn initially | Steeper learning curve |
Real-World Modeling | Less natural representation of real-world entities | More natural representation of real-world entities |
Example Languages | C, Pascal, Fortran | Java, Python, C++, C#, Ruby, Swift |
Choosing the Right Paradigm: Context Matters
The choice between procedural and object-oriented programming depends heavily on the project's nature, size, and complexity.
-
Procedural programming is often preferred for smaller programs, simple tasks, or situations where performance is paramount and the overhead of object management is undesirable. Its simpler structure makes it easier to learn and debug for beginners.
-
Object-oriented programming is generally the preferred choice for larger, more complex projects that require high modularity, reusability, maintainability, and scalability. Its ability to model real-world entities naturally makes it suitable for a wide range of applications.
While the lines between these paradigms are becoming increasingly blurred with the advent of hybrid approaches, understanding the core differences and selecting the appropriate paradigm based on the project’s requirements remains a crucial skill for any software developer. The benefits of choosing the right approach extend far beyond the initial development phase; maintainability, scalability, and long-term success heavily rely on these fundamental programming concepts.
Latest Posts
Latest Posts
-
Five Letter Words Beginning With Ra
May 12, 2025
-
What Is A Group Of Kangaroos
May 12, 2025
-
Least Common Multiple Of 5 And 13
May 12, 2025
-
4 1 8 In Decimal Form
May 12, 2025
-
The Hardest Substance In The Body Is
May 12, 2025
Related Post
Thank you for visiting our website which covers about Difference Between Procedural And Object Oriented Programming . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.