Difference Between Checked Exception And Unchecked Exception
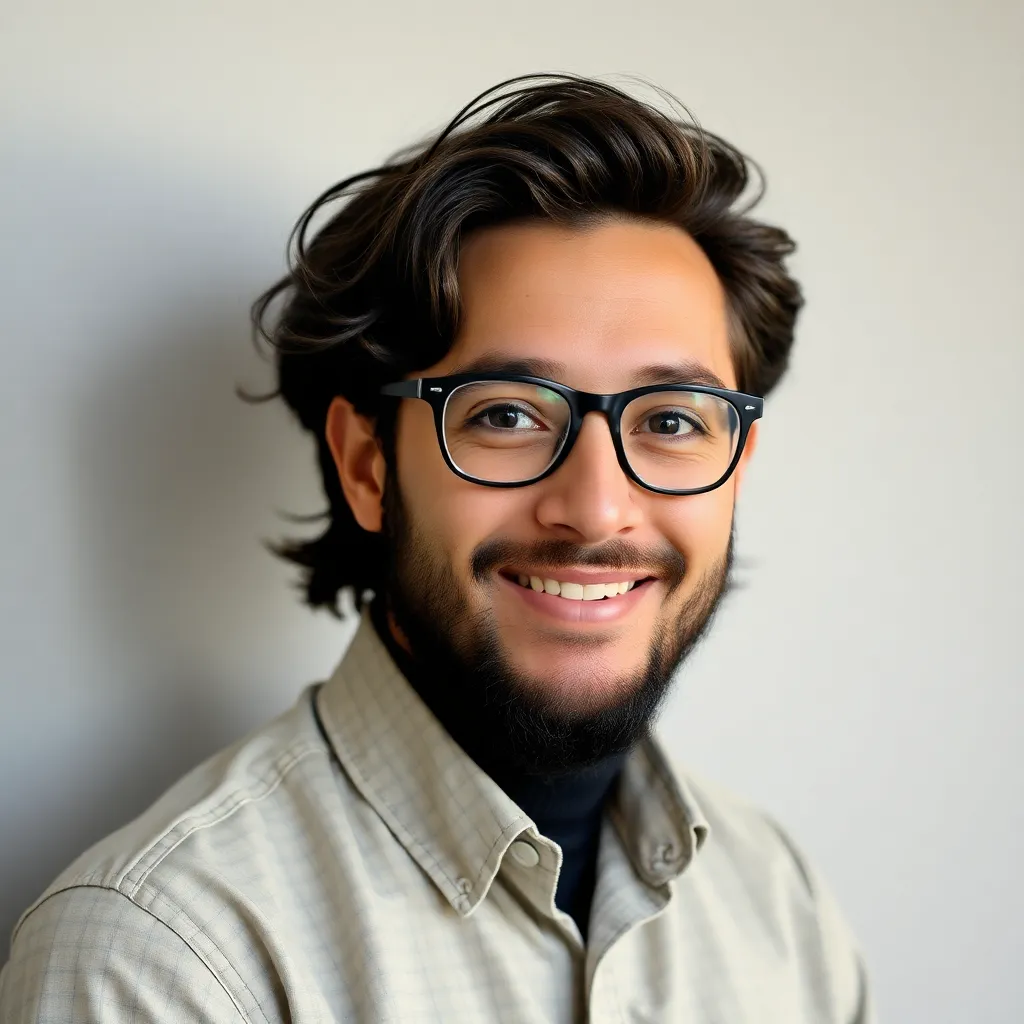
Juapaving
May 14, 2025 · 5 min read
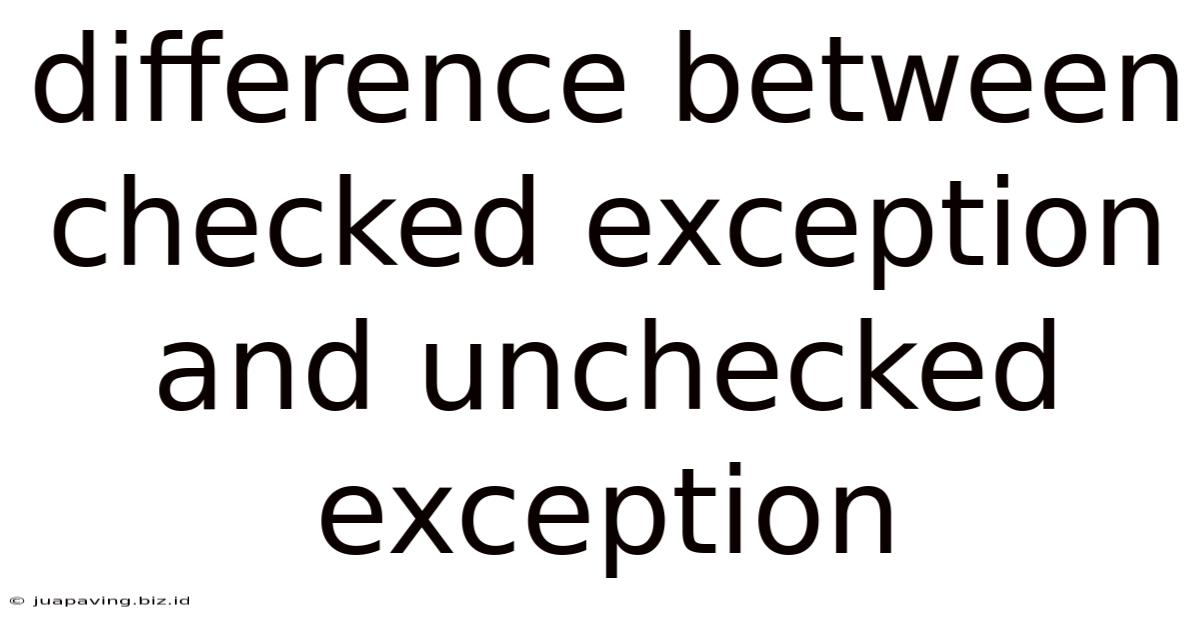
Table of Contents
Checked vs. Unchecked Exceptions in Java: A Deep Dive
Exception handling is a cornerstone of robust Java programming. It's the mechanism that allows your code to gracefully handle unexpected situations, preventing crashes and ensuring a smoother user experience. Central to this mechanism are two key types of exceptions: checked and unchecked exceptions. Understanding the difference between them is crucial for writing reliable and maintainable Java applications. This comprehensive guide will delve into the nuances of checked and unchecked exceptions, exploring their definitions, characteristics, and best practices for handling them.
What are Exceptions?
Before diving into the specifics of checked and unchecked exceptions, let's establish a foundational understanding of exceptions themselves. In Java, an exception is an event that disrupts the normal flow of program execution. This disruption can stem from various sources, including:
- Programming errors: These are mistakes in your code, such as trying to access an array element beyond its bounds (ArrayIndexOutOfBoundsException) or attempting to divide by zero (ArithmeticException).
- Resource issues: Problems accessing external resources like files, network connections, or databases (IOException, SQLException).
- Logical errors: Flaws in your program's logic that lead to unexpected results (NullPointerException, IllegalArgumentException).
When an exception occurs, Java throws an exception object. If not handled, this can lead to program termination. However, the power of exception handling lies in the ability to catch these exceptions and take appropriate action to recover or gracefully handle the error.
Checked Exceptions: The Compile-Time Guardians
Checked exceptions are a specific class of exceptions that the Java compiler forces you to handle explicitly. This means that if your code calls a method that might throw a checked exception, you must either:
- Catch the exception: Use a
try-catch
block to handle the exception. This involves specifying the type of exception you expect and writing code to deal with it appropriately. - Declare the exception: Use a
throws
clause in your method signature to indicate that the method might throw the checked exception. This shifts the responsibility of handling the exception to the calling method.
The key characteristic of checked exceptions is that they represent situations that are often recoverable or at least predictable. They typically arise from external factors or resource limitations that the programmer can anticipate.
Examples of Checked Exceptions:
IOException
: Occurs during input/output operations, such as reading from a file or writing to a network socket.SQLException
: Thrown when database operations fail.ClassNotFoundException
: Indicates that a class required by the program cannot be found.
Why Use Checked Exceptions?
The primary advantage of checked exceptions is improved code reliability and maintainability. The compiler's enforcement ensures that potential problems are addressed during development, preventing runtime surprises. By forcing explicit handling, checked exceptions contribute to more robust and predictable applications.
Example:
import java.io.FileReader;
import java.io.IOException;
public class CheckedExceptionExample {
public static void readFile(String filename) throws IOException {
FileReader reader = new FileReader(filename);
// ... process the file ...
reader.close();
}
public static void main(String[] args) {
try {
readFile("myFile.txt");
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
}
In this example, readFile
declares that it might throw an IOException
. The main
method handles this potential exception using a try-catch
block.
Unchecked Exceptions: The Runtime Rebels
Unchecked exceptions, also known as runtime exceptions, are a different breed. The Java compiler does not force you to handle them explicitly. They typically represent programming errors or unexpected conditions that are less likely to be recoverable or easily predicted.
Examples of Unchecked Exceptions:
NullPointerException
: Thrown when you try to access a member of an object that isnull
.IllegalArgumentException
: Indicates that an invalid argument was passed to a method.ArrayIndexOutOfBoundsException
: Thrown when you try to access an array element using an invalid index.ArithmeticException
: Occurs when an arithmetic operation fails, such as division by zero.
Why Use (or Not Use) Unchecked Exceptions?
Unchecked exceptions offer flexibility. You can choose to handle them or let them propagate up the call stack, potentially resulting in a program crash. While this might seem risky, it often reflects the nature of these exceptions: they frequently represent programming flaws that should be fixed rather than handled with error recovery mechanisms.
Example:
public class UncheckedExceptionExample {
public static int divide(int a, int b) {
return a / b; // Potential ArithmeticException
}
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.err.println("Cannot divide by zero: " + e.getMessage());
}
}
}
In this case, an ArithmeticException
is an unchecked exception. While we're handling it here, it's not strictly required by the compiler.
Key Differences Summarized
Feature | Checked Exception | Unchecked Exception |
---|---|---|
Handling | Compiler forces explicit handling | No compiler-enforced handling |
Type | Subclasses of Exception (excluding RuntimeException ) |
Subclasses of RuntimeException |
Recoverability | Often recoverable | Often unrecoverable (programming errors) |
Predictability | Usually predictable | Often unpredictable |
Examples | IOException , SQLException |
NullPointerException , ArithmeticException |
Best Practices for Exception Handling
Regardless of whether you're dealing with checked or unchecked exceptions, adhering to best practices is essential for creating robust and maintainable code:
- Be Specific: Catch only the exceptions you expect and can handle appropriately. Avoid broad
catch (Exception e)
blocks unless absolutely necessary. - Handle Exceptions Gracefully: Provide informative error messages to the user (or log them for debugging) instead of simply letting the program crash.
- Don't Ignore Exceptions: Unless you have a specific reason to ignore an exception (e.g., in some logging scenarios), handle it properly to prevent unexpected behavior.
- Avoid Excessive Exception Handling: Don't try to handle every possible exception; focus on those that are likely to occur and that you can realistically recover from.
- Use Custom Exceptions: For situations specific to your application, create custom exception classes to provide more context and improve error handling.
- Log Exceptions Thoroughly: Detailed logging helps in debugging and troubleshooting issues in production environments.
Conclusion
The distinction between checked and unchecked exceptions is a fundamental aspect of Java programming. Understanding their characteristics and applying best practices for exception handling are vital for building reliable, robust, and maintainable applications. By carefully considering the nature of each exception and choosing appropriate handling strategies, you can enhance the overall quality and resilience of your Java code. Remember, the goal is not simply to prevent crashes, but to create software that gracefully handles unexpected situations, provides useful feedback, and continues functioning smoothly, even in the face of adversity. This approach fosters a positive user experience and contributes to a more robust and dependable software ecosystem.
Latest Posts
Related Post
Thank you for visiting our website which covers about Difference Between Checked Exception And Unchecked Exception . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.