Difference Between A Variable And A Constant
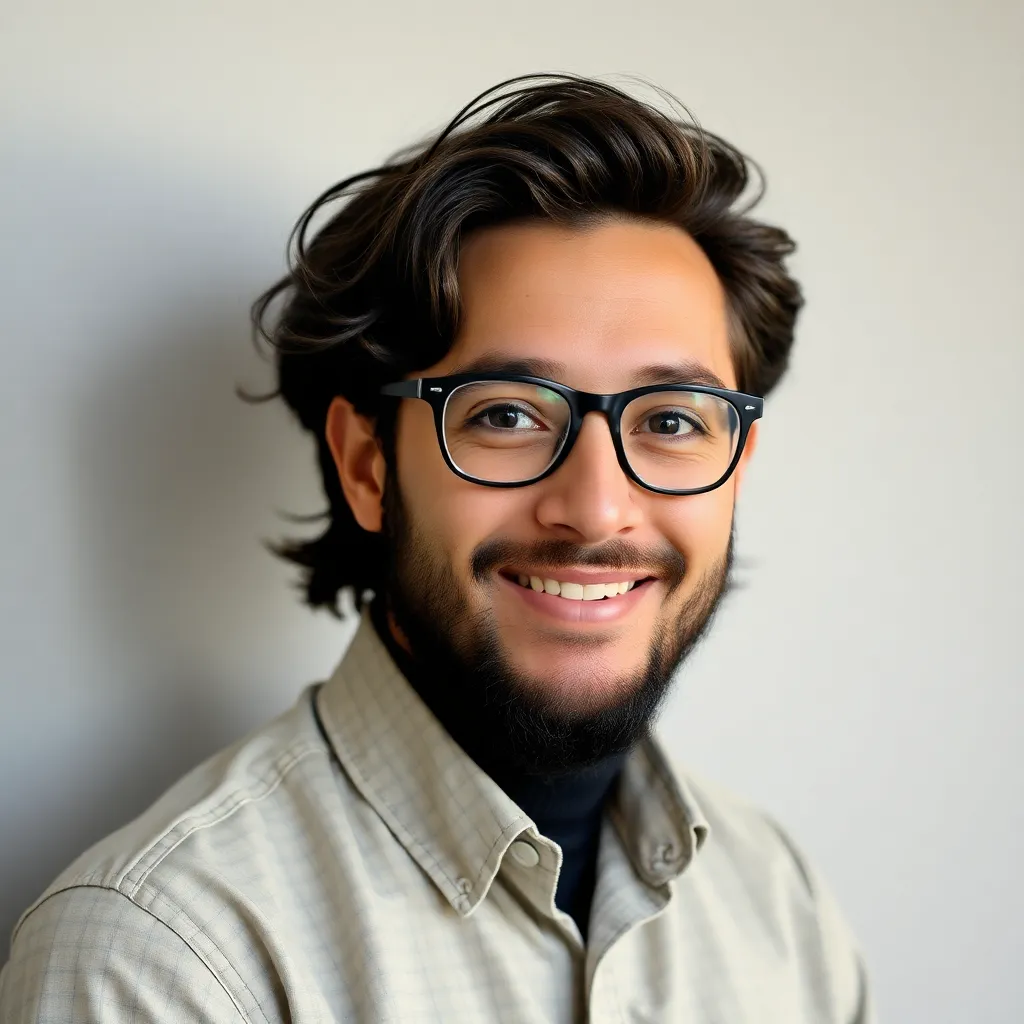
Juapaving
Apr 07, 2025 · 6 min read

Table of Contents
The Fundamental Difference Between Variables and Constants in Programming
Understanding the distinction between variables and constants is foundational to programming. While seemingly simple, grasping their nuances is crucial for writing clean, efficient, and maintainable code. This comprehensive guide will delve deep into the differences, exploring their functionalities, use cases, and the implications of using each correctly.
What is a Variable?
A variable is a named storage location in a computer's memory that holds a value. Think of it as a container that can store different types of data, such as numbers, text (strings), or more complex data structures. The key characteristic of a variable is its mutability – its value can be changed during the execution of a program.
Key Features of Variables:
- Mutability: This is the defining characteristic. The value stored in a variable can be altered throughout the program's runtime.
- Data Types: Variables are typically associated with a data type (e.g., integer, float, string, boolean) which dictates the kind of data they can hold and the operations that can be performed on them. The specific data types supported depend on the programming language.
- Naming Conventions: Programming languages usually have rules for naming variables (e.g., starting with a letter, using only alphanumeric characters and underscores). Good naming practices enhance code readability. Descriptive names are crucial for understanding the variable's purpose.
- Memory Allocation: When a variable is declared, the computer allocates a portion of memory to store its value. The size of this memory allocation depends on the variable's data type.
- Scope: A variable's scope defines the region of the code where it is accessible. Variables can have global scope (accessible from anywhere in the program), local scope (accessible only within a specific function or block of code), or other more specialized scopes depending on the programming language.
Example (Python):
name = "Alice" # Assigning a string value to the variable 'name'
age = 30 # Assigning an integer value to the variable 'age'
print(name, age) # Output: Alice 30
age = 31 # Changing the value of the variable 'age'
print(age) # Output: 31
In this example, name
and age
are variables. Their values are assigned initially and then the value of age
is modified later.
What is a Constant?
A constant, in contrast to a variable, is a named storage location that holds a value that cannot be changed after its initial assignment. It represents a fixed value that remains the same throughout the program's execution.
Key Features of Constants:
- Immutability: This is the defining characteristic. The value of a constant cannot be altered once it has been assigned.
- Named Constants: Constants are often given descriptive names to improve code readability and maintainability. This makes the code's intent clearer.
- Readability and Maintainability: Using constants makes code more readable and easier to maintain. If a value needs to be changed, it only needs to be modified in one place (the constant's declaration), rather than throughout the entire code. This reduces the risk of errors.
- Symbolic Constants: Constants are often used to represent symbolic values, like mathematical constants (π, e), physical constants (speed of light), or configuration settings. This improves the code's clarity and makes it easier to understand.
- Language Support: Not all programming languages explicitly support constants in the same way. Some languages have built-in mechanisms (e.g.,
const
keyword in C++,final
keyword in Java), while others rely on programming conventions (e.g., using all uppercase names for constants in Python).
Example (C++):
const double PI = 3.14159; // Declaring a constant PI
int radius = 5;
double area = PI * radius * radius;
// The following line would result in a compile-time error:
// PI = 3.1416; // Attempting to change the value of a constant
In C++, the const
keyword signifies that PI
is a constant. Any attempt to modify its value after initialization will result in a compile-time error.
Key Differences Summarized:
Feature | Variable | Constant |
---|---|---|
Mutability | Mutable (changeable) | Immutable (unchangeable) |
Value | Can change during program execution | Remains the same throughout execution |
Purpose | Stores data that may change | Stores fixed values that should not change |
Readability | Can sometimes be less readable if not named well | Generally improves readability and maintainability |
Error Prevention | More prone to accidental modification errors | Reduces the risk of accidental modification |
Naming Convention | Usually lowercase with camelCase or snake_case | Often uppercase or with a specific prefix (e.g., k for constant) |
When to Use Variables and Constants
The choice between using a variable or a constant depends entirely on whether the value needs to change during program execution.
Use Variables When:
- The value needs to be updated during program execution. For example, a counter that increments in a loop, or a variable that stores user input.
- The value is inherently dynamic and depends on program conditions. Consider a variable storing the current temperature, which fluctuates over time.
- You need temporary storage for intermediate calculations.
Use Constants When:
- The value represents a fundamental constant like π or the speed of light. These values never change.
- The value represents a configuration setting that should not be modified during runtime (e.g., maximum number of users, database connection string).
- The value represents a symbolic name for a fixed value. Using named constants makes the code self-documenting and easier to understand. For example, using
MAX_ATTEMPTS = 3
instead of hardcoding the number3
in multiple locations improves clarity and maintainability. - You want to enforce immutability to prevent accidental modification of critical values.
Best Practices for Variables and Constants
- Descriptive Naming: Choose names that clearly indicate the purpose of the variable or constant.
- Consistent Naming Conventions: Follow the naming conventions of your programming language to enhance readability.
- Data Type Considerations: Select appropriate data types for variables to optimize memory usage and prevent type-related errors.
- Comments: Add comments to explain the purpose of variables and constants, especially if their names aren't entirely self-explanatory.
- Avoid Magic Numbers: Don't use "magic numbers" (hardcoded numerical values without clear meaning) in your code. Instead, replace them with named constants.
Conclusion
Variables and constants are essential building blocks of any program. Understanding their differences—particularly mutability versus immutability—is critical for writing effective and maintainable code. By employing appropriate naming conventions and choosing between variables and constants judiciously, you can significantly improve the clarity, reliability, and overall quality of your software. Remember that while variables provide flexibility, constants enhance readability, prevent errors, and contribute to a more robust and understandable program structure. Mastering the use of both is a key skill for any programmer.
Latest Posts
Latest Posts
-
Is Formic Acid A Weak Acid
Apr 09, 2025
-
Find Eigenvalues And Eigenvectors Of A 3x3 Matrix
Apr 09, 2025
-
What Is 13 16 As A Decimal
Apr 09, 2025
-
How Many Electrons Are In The Valence Shell Of Carbon
Apr 09, 2025
-
Difference Between Biotic And Abiotic Factors
Apr 09, 2025
Related Post
Thank you for visiting our website which covers about Difference Between A Variable And A Constant . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.