Complete The Function Definition To Return The Hours Given Minutes.
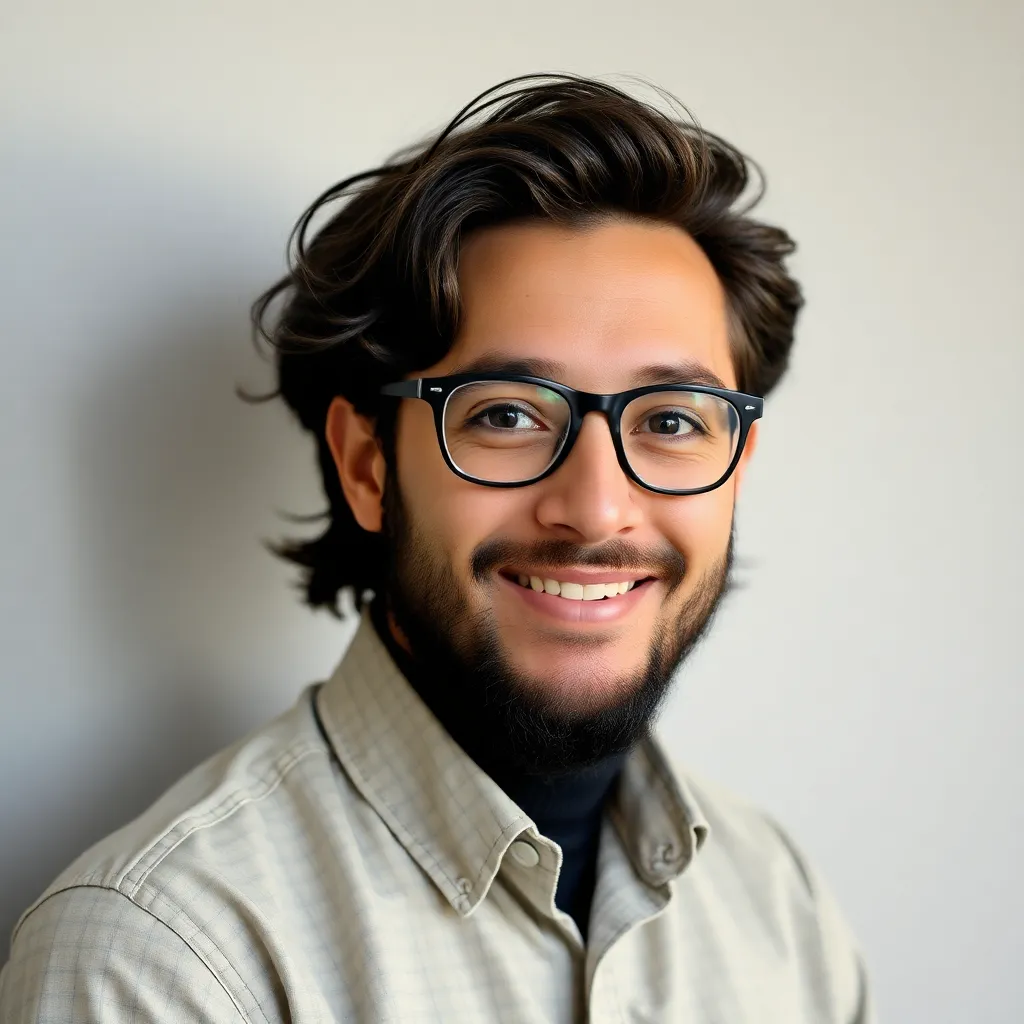
Juapaving
May 24, 2025 · 5 min read
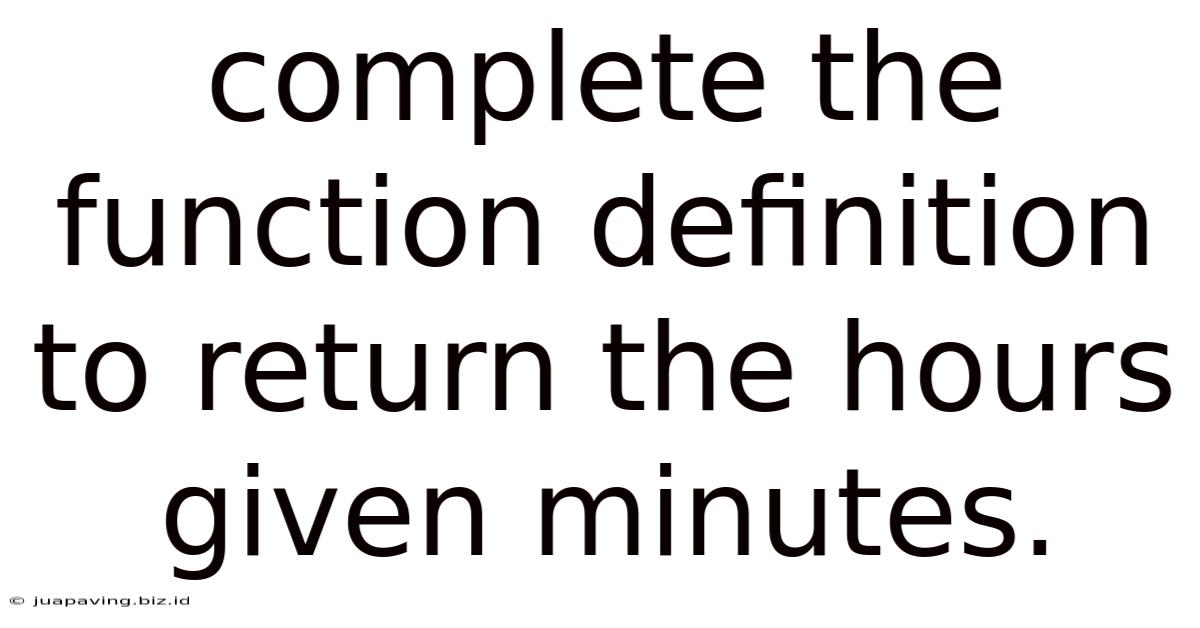
Table of Contents
Complete the Function Definition to Return Hours Given Minutes: A Deep Dive into Time Conversion
Converting minutes to hours is a fundamental task in many programming scenarios, from simple time calculations to complex scheduling systems. This article provides a comprehensive guide on how to complete a function definition to accurately perform this conversion, exploring various approaches, handling edge cases, and optimizing for efficiency and readability. We'll delve into different programming languages and discuss best practices for writing robust and maintainable code.
Understanding the Core Conversion Logic
The basic principle behind converting minutes to hours is straightforward: there are 60 minutes in one hour. Therefore, to find the number of hours, we simply divide the total number of minutes by 60. However, the real-world application often involves handling fractional parts and ensuring the output is appropriately formatted.
Function Definition in Python
Python, known for its readability and versatility, offers an elegant solution for this conversion. Let's consider a function that takes the number of minutes as input and returns the equivalent number of hours:
def minutes_to_hours(minutes):
"""Converts minutes to hours.
Args:
minutes: The number of minutes to convert.
Returns:
The equivalent number of hours as a float. Returns -1 if input is invalid.
"""
if minutes < 0:
return -1 #Handle negative input
return minutes / 60
#Example Usage
print(minutes_to_hours(120)) # Output: 2.0
print(minutes_to_hours(75)) # Output: 1.25
print(minutes_to_hours(-30)) # Output: -1
This Python function efficiently handles the conversion. Note the inclusion of error handling for negative input, a crucial aspect of robust function design. The use of a docstring enhances readability and maintainability.
Handling Fractional Hours and Different Data Types
The above function returns a floating-point number representing the hours, accurately reflecting fractional parts. However, depending on the application, you might need to handle the output differently. For example:
- Rounding: You might want to round the result to the nearest whole number using the
round()
function. This is useful when dealing with scenarios where fractional hours are not relevant (e.g., scheduling tasks in whole hours).
def minutes_to_hours_rounded(minutes):
"""Converts minutes to hours, rounding to the nearest whole number."""
if minutes < 0:
return -1
return round(minutes / 60)
print(minutes_to_hours_rounded(75)) # Output: 1
- Integer Division: If you only need the whole number of hours, discarding the fractional part, you can use integer division (
//
in Python).
def minutes_to_hours_integer(minutes):
"""Converts minutes to hours using integer division."""
if minutes < 0:
return -1
return minutes // 60
print(minutes_to_hours_integer(75)) # Output: 1
- Different Input Types: The function can be made more robust by handling different input data types. For instance, it might receive the input as a string. In such cases, you need to include error handling for invalid input types and convert the input to a numerical type before performing the calculation.
def minutes_to_hours_robust(minutes):
"""Converts minutes to hours, handling various input types."""
try:
minutes = float(minutes) #Attempt to convert to float
if minutes < 0:
return -1
return minutes / 60
except ValueError:
return -1 # Handle invalid input
print(minutes_to_hours_robust("120")) # Output: 2.0
print(minutes_to_hours_robust("abc")) #Output: -1
Function Definition in JavaScript
JavaScript, a prevalent language for web development, provides a similar approach for this conversion. Here's a JavaScript function:
function minutesToHours(minutes) {
//Error Handling for negative values
if (minutes < 0) {
return -1;
}
return minutes / 60;
}
console.log(minutesToHours(120)); // Output: 2
console.log(minutesToHours(75)); // Output: 1.25
console.log(minutesToHours(-30)); //Output: -1
This JavaScript function mirrors the Python function in its core logic and error handling.
Function Definition in C++
C++, a powerful language for system programming, requires a slightly different approach due to its strong typing:
#include
double minutesToHours(double minutes) {
if (minutes < 0) {
return -1.0; // Handle negative input
}
return minutes / 60.0;
}
int main() {
std::cout << minutesToHours(120.0) << std::endl; // Output: 2
std::cout << minutesToHours(75.0) << std::endl; // Output: 1.25
std::cout << minutesToHours(-30.0) << std::endl; // Output: -1
return 0;
}
This C++ function utilizes double
to handle floating-point numbers accurately. The main
function demonstrates example usage.
Advanced Considerations: Large-Scale Applications and Libraries
For large-scale applications dealing with extensive time calculations, consider using dedicated date and time libraries. These libraries often provide optimized functions for time conversions and other related operations, handling various time zones and formats efficiently. Examples include the datetime
module in Python and similar libraries in other languages. These libraries handle potential complexities such as leap years and daylight saving time adjustments, ensuring accuracy in diverse scenarios.
Testing and Debugging
Thorough testing is paramount for any function, especially those dealing with numerical calculations. Create a comprehensive suite of test cases, including:
- Positive test cases: Cover a wide range of valid minute values, including whole numbers and fractional numbers.
- Negative test cases: Test the function's behavior with negative input values. Ensure it handles these cases gracefully (e.g., returning an error code or raising an exception).
- Edge cases: Test boundary conditions, such as zero minutes or very large minute values.
- Invalid input types: Test the function's robustness against invalid input types (e.g., strings, objects).
Employ debugging techniques to identify and fix any issues that arise during testing. Using a debugger allows you to step through the code line by line, examining variables and identifying potential errors.
Code Optimization
For performance-critical applications, consider optimization strategies. While the basic division operation is already very efficient, in extremely high-performance settings, pre-calculated lookup tables or other techniques might offer marginal improvements, though the overhead of creating and maintaining such structures needs to be weighed against the potential gains.
Documenting Your Function
Clear and comprehensive documentation is crucial for maintainability and collaboration. Use docstrings (Python) or JSDoc (JavaScript) to explain the function's purpose, parameters, return values, and any error handling mechanisms. This makes it easier for others (and your future self) to understand and use the function correctly.
Conclusion
Completing the function definition to convert minutes to hours is a seemingly simple task, yet it underscores important programming principles: error handling, data type considerations, efficiency, testing, and documentation. By carefully addressing these aspects, you can create robust, reliable, and maintainable code that will serve as a valuable component in larger applications. Remember to always choose the implementation best suited to your specific needs and context, considering factors like performance requirements, code readability, and the complexity of your overall project. Using dedicated date and time libraries for larger projects is highly recommended to handle complexities efficiently and accurately.
Latest Posts
Latest Posts
-
Act 1 Scene 3 Of Julius Caesar
May 24, 2025
-
Cliff Notes On Who Moved My Cheese
May 24, 2025
-
Do Defense Attorneys Know The Truth
May 24, 2025
-
Art Labeling Activity Blood Vessels Of The Thoracic Cavity
May 24, 2025
-
Spark Notes Tale Of Two Cities
May 24, 2025
Related Post
Thank you for visiting our website which covers about Complete The Function Definition To Return The Hours Given Minutes. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.