6.10.6: Handling Multiple Exceptions: Vending Machine Example.
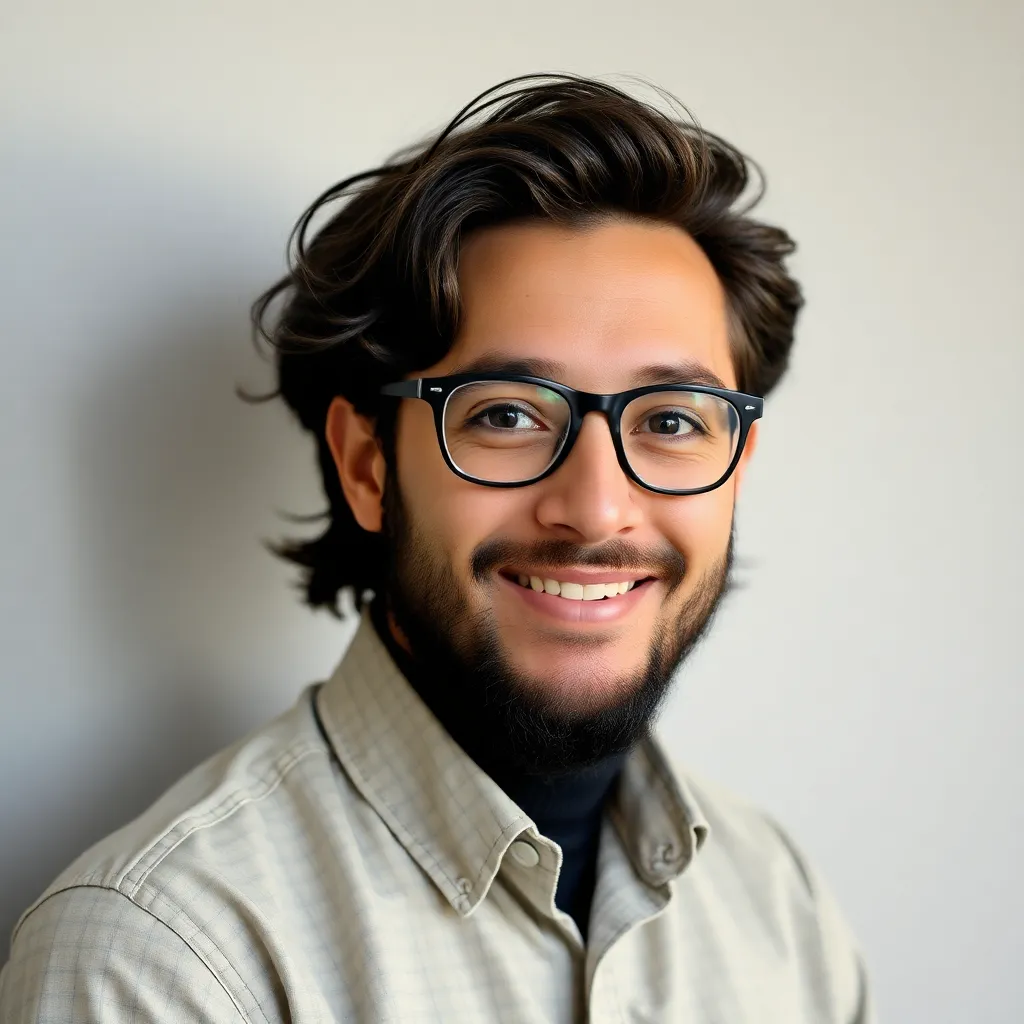
Juapaving
May 24, 2025 · 5 min read
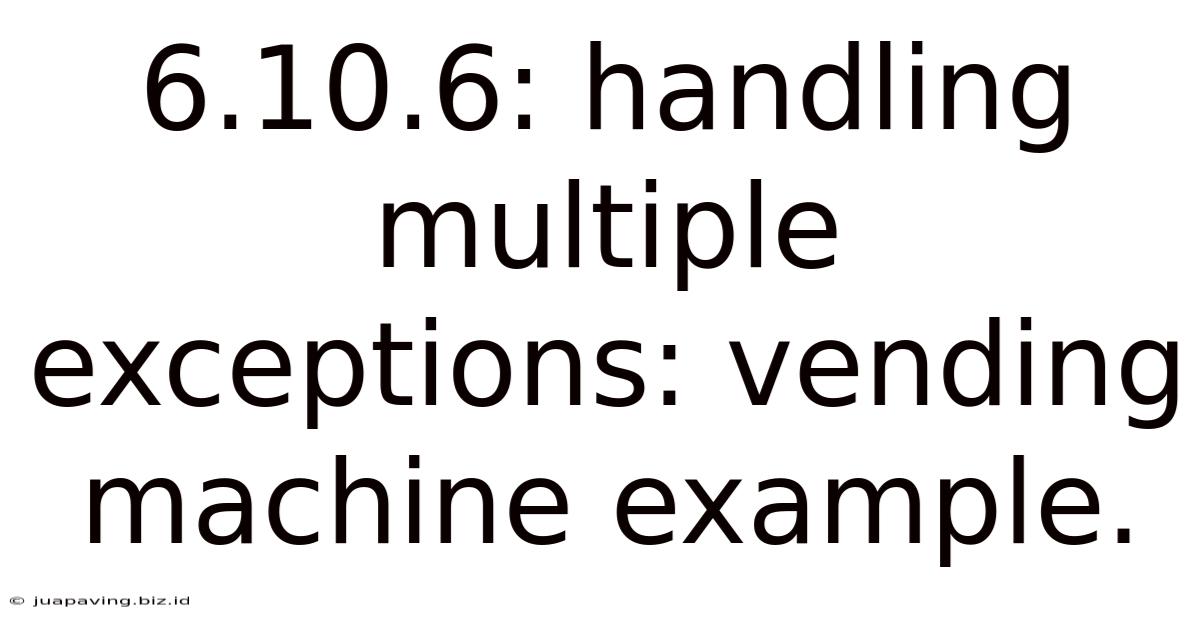
Table of Contents
6.10.6: Handling Multiple Exceptions: A Vending Machine Example
Exception handling is a crucial aspect of robust software development. It allows your programs to gracefully handle unexpected errors, preventing crashes and providing informative feedback to the user. While handling a single exception type is straightforward, real-world applications often require managing multiple potential exceptions. This article delves into the intricacies of handling multiple exceptions using a practical example: a vending machine simulator. We'll explore various techniques and best practices, illustrating how to build resilient and user-friendly applications.
Understanding Exception Handling Fundamentals
Before diving into the vending machine example, let's recap the basics of exception handling in Python. Exceptions are events that disrupt the normal flow of a program. They arise from various sources, including:
ZeroDivisionError
: Attempting to divide by zero.TypeError
: Performing an operation on incompatible data types.IndexError
: Accessing an index that is out of bounds in a sequence (e.g., list, tuple).FileNotFoundError
: Attempting to open a file that doesn't exist.ValueError
: Passing an invalid value to a function or operation.KeyError
: Trying to access a key that is not present in a dictionary.
Python's try-except
block is the primary mechanism for handling exceptions. The try
block contains the code that might raise an exception, while the except
block specifies how to handle the exception if it occurs.
try:
result = 10 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError:
print("Error: Cannot divide by zero.")
The Vending Machine Scenario
Let's imagine we're building a software simulator for a vending machine. This machine dispenses items based on user input (item selection and payment). Several exceptions could occur during this process:
- Insufficient Funds: The user doesn't have enough money to purchase the selected item.
- Item Out of Stock: The selected item is not available in the machine.
- Invalid Selection: The user enters an invalid item code.
- Payment System Error: A problem occurs during the payment processing (simulated for our example).
Implementing Multiple Exception Handling
We'll now develop a Python program that simulates a vending machine and handles these multiple exceptions:
class VendingMachine:
def __init__(self, inventory):
self.inventory = inventory # Dictionary: {item_code: (price, quantity)}
self.balance = 0
def display_inventory(self):
print("Available Items:")
for item_code, (price, quantity) in self.inventory.items():
print(f"{item_code}: ${price:.2f} ({quantity} available)")
def select_item(self, item_code):
if item_code not in self.inventory:
raise ValueError("Invalid item code.")
price, quantity = self.inventory[item_code]
if quantity == 0:
raise ValueError("Item is out of stock.")
return price
def make_payment(self, amount, price):
if amount < price:
raise ValueError("Insufficient funds.")
self.balance += amount - price # Update balance with change
return True
def dispense_item(self, item_code):
price, quantity = self.inventory[item_code]
self.inventory[item_code] = (price, quantity - 1)
print(f"Dispensing {item_code}. Your change is ${self.balance:.2f}")
self.balance = 0 # Reset balance after dispensing
def main():
inventory = {
"A1": (1.50, 3),
"B2": (2.00, 5),
"C3": (1.00, 2),
}
vending_machine = VendingMachine(inventory)
while True:
vending_machine.display_inventory()
try:
item_code = input("Enter item code (or 'q' to quit): ").upper()
if item_code == 'Q':
break
price = vending_machine.select_item(item_code)
amount = float(input(f"Enter amount to pay (${price:.2f}): "))
vending_machine.make_payment(amount, price)
vending_machine.dispense_item(item_code)
except ValueError as e:
print(f"Error: {e}")
except Exception as e: # Catch any other unexpected exceptions
print(f"An unexpected error occurred: {e}")
if __name__ == "__main__":
main()
This improved code uses multiple except
blocks to handle specific exceptions (ValueError
for insufficient funds, invalid selection, etc.). A general except Exception
block catches any other unforeseen errors, providing a safety net. This structured approach enhances the program's robustness.
Advanced Techniques: Exception Chaining and Custom Exceptions
For more complex scenarios, you can leverage exception chaining and create custom exceptions.
Exception Chaining: Allows you to raise a new exception while preserving the original exception's context. This is helpful for providing more detailed error information.
try:
# ... some code that might raise an exception ...
except Exception as e:
raise RuntimeError("A critical error occurred.") from e # Chain the original exception
Custom Exceptions: Defining your own exception classes allows you to create highly specific exception types tailored to your application's needs.
class InsufficientFundsError(Exception):
pass
class ItemOutOfStockError(Exception):
pass
# ... within the VendingMachine class ...
def make_payment(self, amount, price):
if amount < price:
raise InsufficientFundsError("Insufficient funds.")
By using custom exceptions, your code becomes more readable and maintainable, as the exception types directly reflect the specific errors within your vending machine's logic.
Logging and Debugging
In a production environment, you would integrate logging to record exceptions and other relevant information for debugging and analysis. The logging
module in Python provides powerful tools for this purpose.
import logging
logging.basicConfig(filename='vending_machine.log', level=logging.ERROR,
format='%(asctime)s - %(levelname)s - %(message)s')
# ... within the main function ...
except Exception as e:
logging.exception("An unexpected error occurred.") # Log the exception with traceback
print(f"An unexpected error occurred. Please check the log file.")
This snippet configures the logger to write error messages to a file named vending_machine.log
. The logging.exception()
method automatically includes the full traceback, invaluable for identifying the root cause of errors.
Testing and Validation
Thorough testing is essential for ensuring that your exception handling mechanisms work correctly. Write unit tests that cover various scenarios, including edge cases and error conditions. Tools like unittest
or pytest
can be used to automate testing and improve the reliability of your code.
Conclusion
Handling multiple exceptions effectively is vital for building robust and user-friendly applications. The vending machine example demonstrates how to use multiple except
blocks, custom exceptions, exception chaining, and logging to create a resilient system. By employing these techniques and focusing on thorough testing, you can significantly improve the quality and reliability of your software. Remember that clear, informative error messages are crucial for a positive user experience. A well-designed exception handling strategy not only prevents crashes but also facilitates debugging and improves the overall maintainability of your code. Adopting these best practices contributes to a more professional and dependable software product.
Latest Posts
Latest Posts
-
1 1 Discussion Population Samples And Bias
May 24, 2025
-
How Was The Pillage And Gift System Useful To Nobles
May 24, 2025
-
The Things They Carried How To Tell A War Story
May 24, 2025
-
All The Characters In To Kill A Mockingbird
May 24, 2025
-
Which Of The Following Is A Valid Conversion Factor
May 24, 2025
Related Post
Thank you for visiting our website which covers about 6.10.6: Handling Multiple Exceptions: Vending Machine Example. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.