4.17 Lab: Mad Lib - Loops
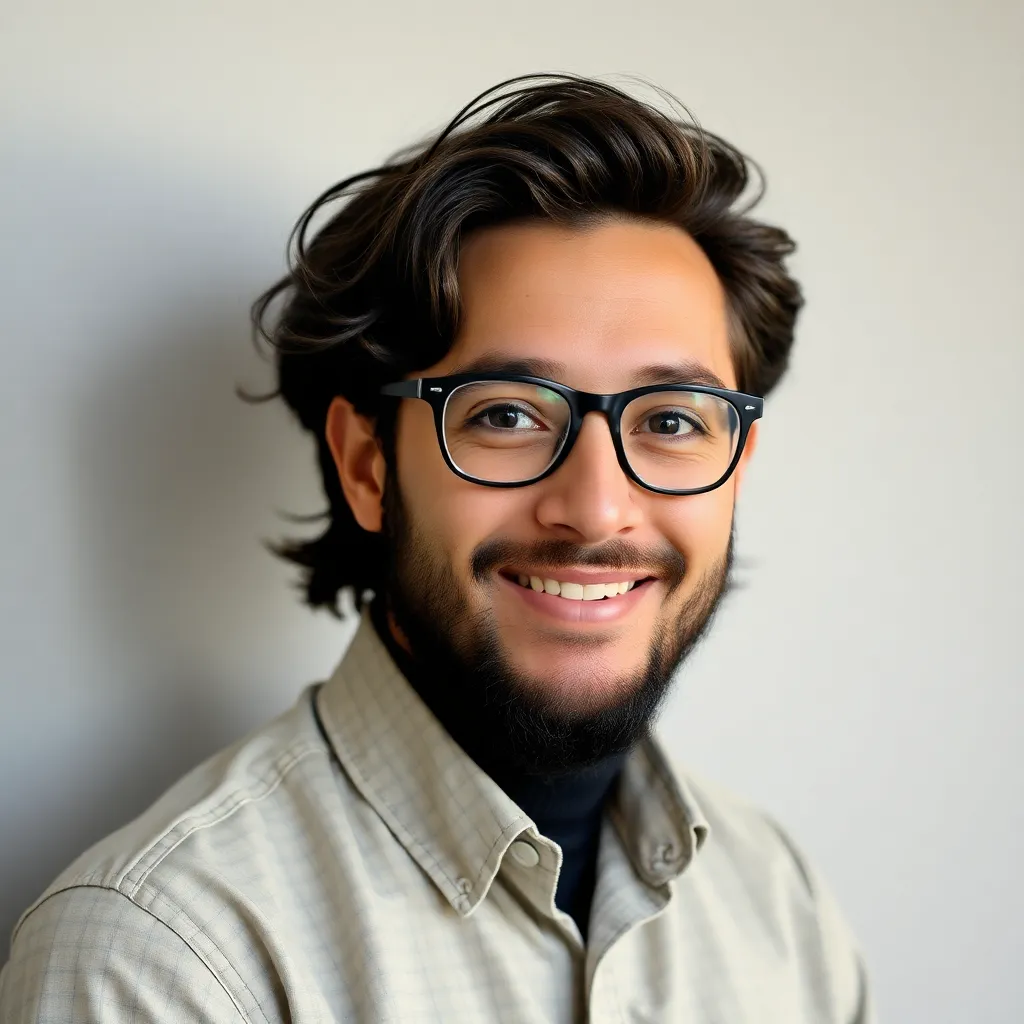
Juapaving
May 24, 2025 · 7 min read
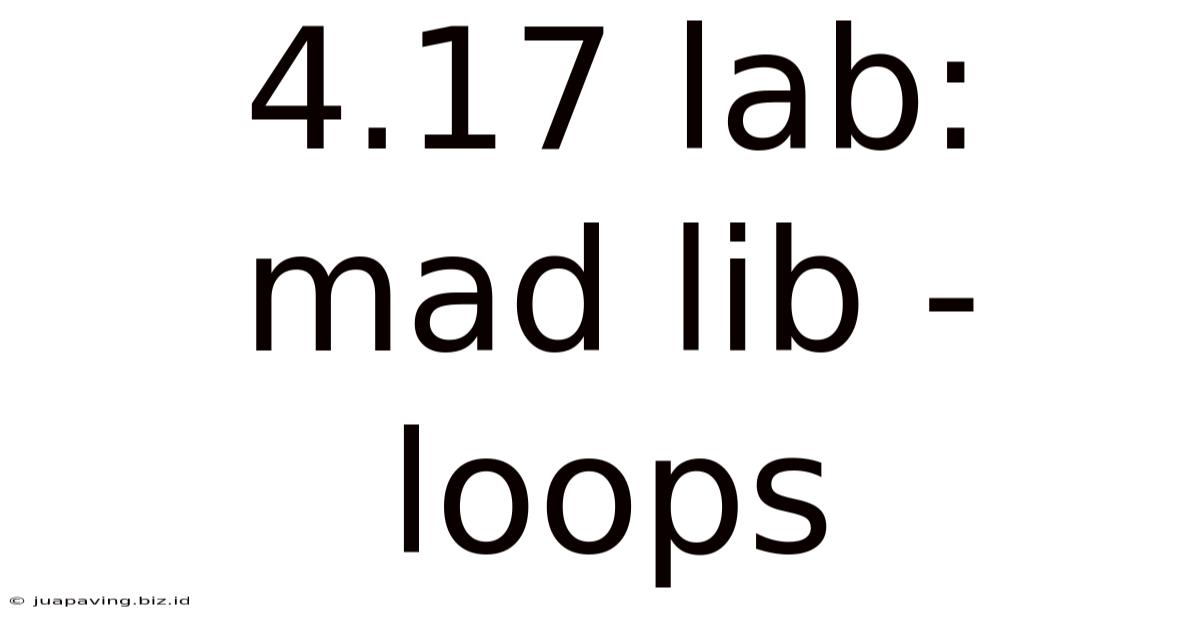
Table of Contents
4.17 Lab: Mad Libs – Loops: A Deep Dive into Python Programming
This comprehensive guide delves into the "4.17 Lab: Mad Libs – Loops" exercise, a common programming assignment designed to teach fundamental programming concepts, specifically focusing on the practical application of loops in Python. We'll explore various approaches, best practices, and advanced techniques to enhance your understanding and build a robust, user-friendly Mad Libs generator.
Understanding the Mad Libs Game
Before we dive into the coding aspect, let's understand the core mechanics of a Mad Libs game. The game involves a story template with several blanks representing different parts of speech (nouns, verbs, adjectives, adverbs, etc.). Players provide words corresponding to these blanks without knowing the context, creating a humorous and often nonsensical story. Our Python program will automate this process, taking user input and dynamically generating the final story.
The Basic Python Implementation: Using while
loops
A simple approach uses while
loops and user input to populate the blanks. This method offers a good starting point for understanding the fundamental concepts.
# Basic Mad Libs using while loops
story = """
Once upon a time, there was a {adjective} {noun} who lived in a {adjective} {place}.
One day, the {noun} decided to {verb} to the {adjective} {place}.
On their journey, they met a {adjective} {animal} who helped them {verb} a {adjective} {object}.
In the end, the {noun} returned home feeling very {adjective}."""
print("Welcome to Mad Libs!")
blanks = ["adjective", "noun", "adjective", "place", "noun", "verb", "adjective", "place", "adjective", "animal", "verb", "adjective", "object", "noun", "adjective"]
words = []
i = 0
while i < len(blanks):
word = input(f"Please enter a {blanks[i]}: ")
words.append(word)
i += 1
# Replace placeholders in the story
result = story.format(adjective=words[0], noun=words[1], adjective=words[2], place=words[3], noun=words[4], verb=words[5], adjective=words[6], place=words[7], adjective=words[8], animal=words[9], verb=words[10], adjective=words[11], object=words[12], noun=words[13], adjective=words[14])
print("\nYour Mad Libs story:\n")
print(result)
This code iteratively prompts the user for words until all blanks are filled. The format()
method elegantly substitutes the user-provided words into the story template.
Advantages of the while
loop approach:
- Simplicity: Easy to understand and implement, especially for beginners.
- Flexibility: Allows for easy modification of the story template and the number of blanks.
Disadvantages of the while
loop approach:
- Repetitive code: The loop structure can become verbose for a large number of blanks.
- Error handling: Lacks robust error handling (e.g., handling non-string inputs).
Enhancing the Program with for
loops and Lists
Utilizing for
loops and lists improves code readability and efficiency. We can store the blanks and corresponding user inputs in lists, simplifying the iteration process.
# Mad Libs using for loops and lists
story = """
Once upon a time, there was a {adjective} {noun} who lived in a {adjective} {place}.
One day, the {noun} decided to {verb} to the {adjective} {place}.
On their journey, they met a {adjective} {animal} who helped them {verb} a {adjective} {object}.
In the end, the {noun} returned home feeling very {adjective}."""
blanks = ["adjective", "noun", "adjective", "place", "noun", "verb", "adjective", "place", "adjective", "animal", "verb", "adjective", "object", "noun", "adjective"]
words = []
print("Welcome to Mad Libs!")
for blank in blanks:
word = input(f"Please enter a {blank}: ")
words.append(word)
# Using zip to efficiently format the string
result = story.format(*dict(zip(blanks, words)))
print("\nYour Mad Libs story:\n")
print(result)
This version leverages zip
to efficiently pair blanks with user inputs, making the format
method call more concise. The for
loop structure is cleaner and more readable than the equivalent while
loop.
Advantages of the for
loop and list approach:
- Readability: More concise and easier to understand.
- Efficiency: Improved iteration efficiency for a large number of blanks.
- Maintainability: Easier to modify and extend the program.
Adding Error Handling and Input Validation
Robust error handling is crucial for a user-friendly application. We can enhance the program by validating user inputs and handling potential errors.
# Mad Libs with error handling
story = """
Once upon a time, there was a {adjective} {noun} who lived in a {adjective} {place}.
One day, the {noun} decided to {verb} to the {adjective} {place}.
On their journey, they met a {adjective} {animal} who helped them {verb} a {adjective} {object}.
In the end, the {noun} returned home feeling very {adjective}."""
blanks = ["adjective", "noun", "adjective", "place", "noun", "verb", "adjective", "place", "adjective", "animal", "verb", "adjective", "object", "noun", "adjective"]
words = []
print("Welcome to Mad Libs!")
for blank in blanks:
while True:
word = input(f"Please enter a {blank}: ")
if word: # Check if input is not empty
words.append(word)
break
else:
print("Please enter a valid word.")
# Using zip to efficiently format the string
result = story.format(*dict(zip(blanks, words)))
print("\nYour Mad Libs story:\n")
print(result)
This improved version includes a while
loop inside the for
loop to ensure that the user provides a non-empty input for each blank.
Advanced Features: Function Modularization and File Input
To further improve the program's structure and reusability, we can modularize it using functions. We can also read the story template from a file, making it easily customizable without modifying the code directly.
# Mad Libs with functions and file input
def read_story_from_file(filename="madlibs_template.txt"):
"""Reads the story template from a file."""
try:
with open(filename, "r") as file:
return file.read()
except FileNotFoundError:
print(f"Error: File '{filename}' not found.")
return None
def get_user_input(blanks):
"""Gets user input for the blanks with error handling."""
words = []
for blank in blanks:
while True:
word = input(f"Please enter a {blank}: ")
if word:
words.append(word)
break
else:
print("Please enter a valid word.")
return words
def generate_madlibs(story, blanks):
"""Generates the Mad Libs story."""
words = get_user_input(blanks)
try:
return story.format(*dict(zip(blanks, words)))
except KeyError:
print("Error: Mismatch between blanks and story template.")
return None
# Main program
filename = "madlibs_template.txt" # Replace with your template file
story_template = read_story_from_file(filename)
if story_template:
blanks = ["adjective", "noun", "adjective", "place", "noun", "verb", "adjective", "place", "adjective", "animal", "verb", "adjective", "object", "noun", "adjective"] # Update blanks as needed based on template.
madlibs_story = generate_madlibs(story_template, blanks)
if madlibs_story:
print("\nYour Mad Libs story:\n")
print(madlibs_story)
This version introduces three functions: read_story_from_file
, get_user_input
, and generate_madlibs
. This improves code organization and allows for easier maintenance and extension. The story template is now loaded from a file, offering flexibility and customization. Error handling is also incorporated to gracefully manage file not found and format errors.
Conclusion: Iterative Improvement and Further Enhancements
The "4.17 Lab: Mad Libs – Loops" exercise serves as an excellent introduction to programming with loops in Python. By iteratively improving the program, adding features such as error handling and file input, we have built a robust and user-friendly Mad Libs generator. Future enhancements could include:
- More sophisticated input validation: Check for specific parts of speech using regular expressions or Natural Language Processing (NLP) techniques.
- Different story templates: Allow users to select from a variety of story templates.
- GUI implementation: Develop a graphical user interface (GUI) for a more interactive user experience using libraries like Tkinter or PyQt.
- Saving generated stories: Allow users to save their generated stories to a file.
- Multiplayer functionality: Extend the program to support multiple players.
This detailed exploration of the Mad Libs program highlights the importance of iterative development, modular design, and robust error handling in creating effective and user-friendly Python applications. Remember that consistent practice and exploration of different approaches are key to mastering programming concepts and building increasingly complex applications.
Latest Posts
Latest Posts
-
4 08 Unit Test Creating An American Mythology
May 24, 2025
-
Which Scenario Is An Example Of Irony
May 24, 2025
-
The Adventures Of Tom Sawyer Characters
May 24, 2025
-
Bioman Protein Synthesis Race Answer Key
May 24, 2025
-
A Dog Training Business Began On December 1
May 24, 2025
Related Post
Thank you for visiting our website which covers about 4.17 Lab: Mad Lib - Loops . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.