2.09 Unit Test Radicals And Complex Numbers
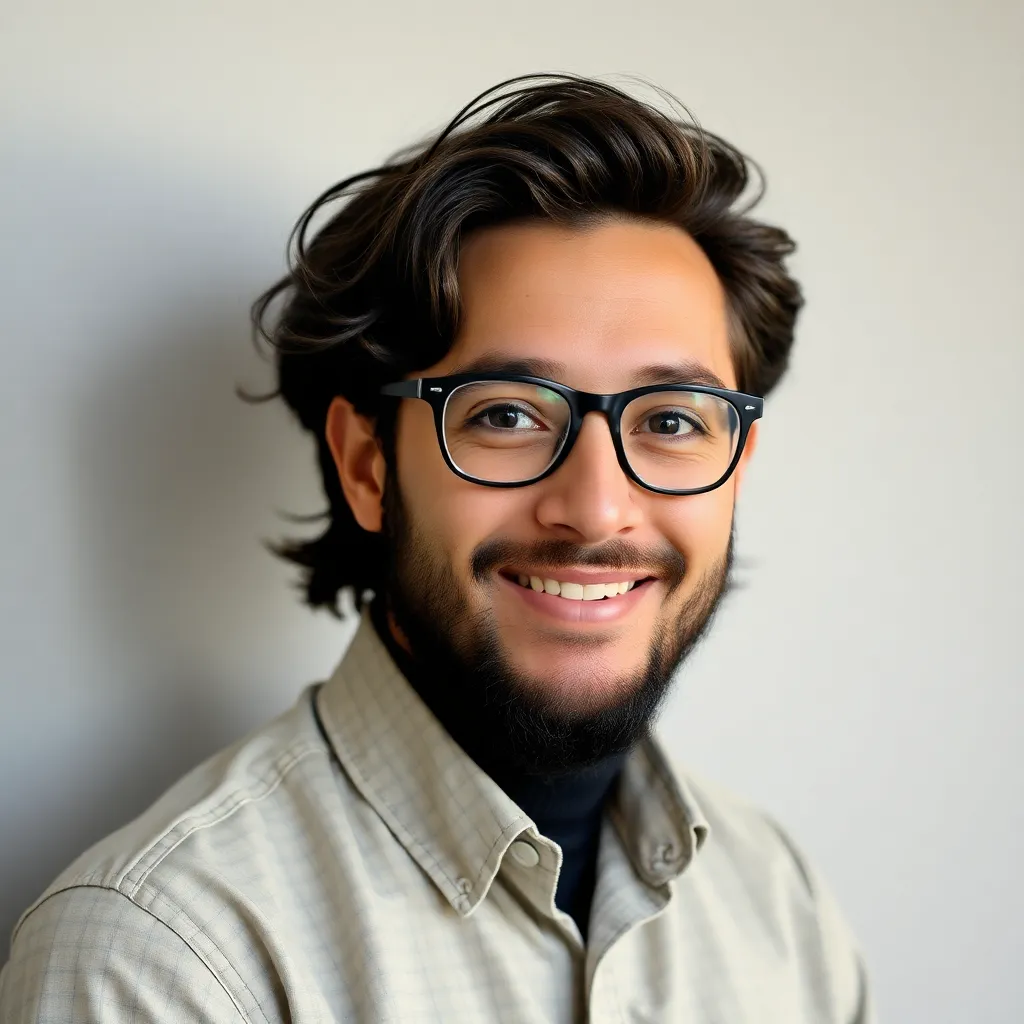
Juapaving
May 25, 2025 · 6 min read
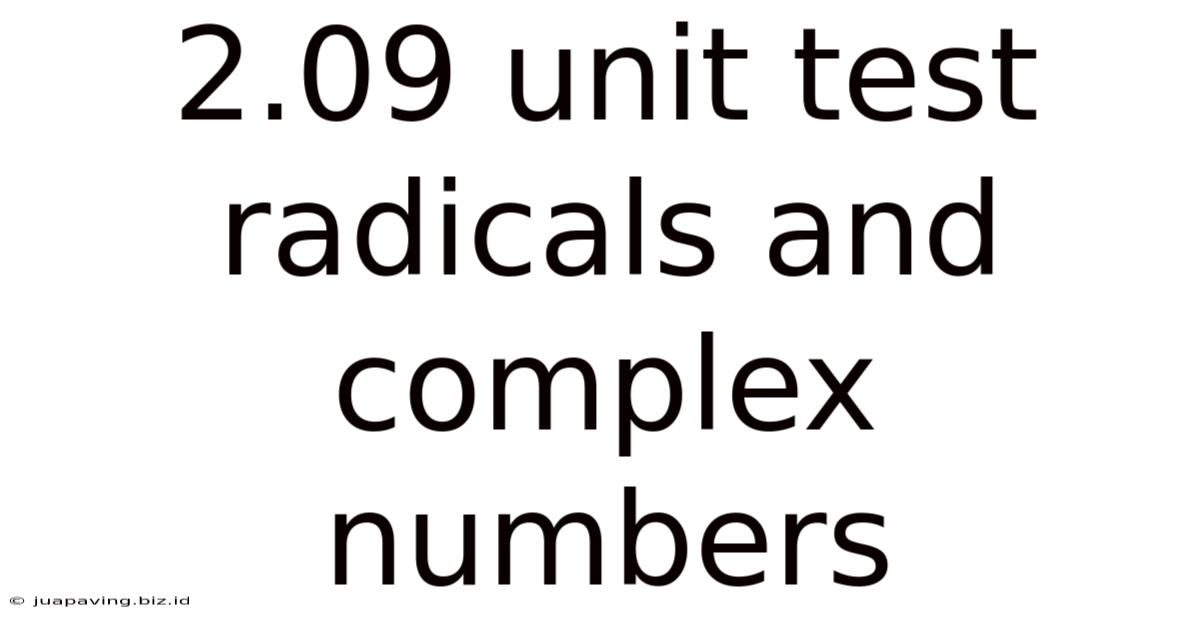
Table of Contents
2.09 Unit Test Radicals and Complex Numbers: A Comprehensive Guide
Unit testing is a crucial part of software development, ensuring individual components function correctly before integration. When dealing with mathematical functions, like those involving radicals and complex numbers, rigorous unit testing becomes even more critical due to the potential for subtle errors and unexpected behavior. This article dives deep into creating robust unit tests for functions handling radicals and complex numbers, focusing on best practices and common pitfalls.
Understanding Radicals and Complex Numbers
Before diving into the testing methodologies, let's briefly review the fundamentals of radicals and complex numbers.
Radicals: A radical expression involves a root (square root, cube root, etc.) of a number. For example, √9 (the square root of 9) is 3. However, dealing with radicals introduces complexities:
- Non-real solutions: Even-numbered roots of negative numbers are not real numbers. For example, √-4 is not a real number. This is where complex numbers come in.
- Domain restrictions: Certain radical expressions have restricted domains. For example, √x is only defined for non-negative values of x.
- Simplification: Radicals often require simplification. For instance, √12 can be simplified to 2√3. Testing should account for both simplified and unsimplified forms.
Complex Numbers: A complex number is a number that can be expressed in the form a + bi, where 'a' and 'b' are real numbers, and 'i' is the imaginary unit (√-1). 'a' is the real part, and 'b' is the imaginary part. Testing complex number functions requires considering:
- Real and imaginary components: Tests should verify the correct calculation of both the real and imaginary parts.
- Complex arithmetic operations: Unit tests should cover addition, subtraction, multiplication, and division of complex numbers.
- Polar representation: Complex numbers can also be represented in polar form (r(cosθ + isinθ)). Tests might need to handle conversions between Cartesian and polar forms.
Designing Effective Unit Tests for Radicals and Complex Numbers
Effective unit testing necessitates a structured approach. Here's a breakdown of key considerations:
1. Test-Driven Development (TDD): Ideally, unit tests should be written before the implementation of the functions being tested. This "test-first" approach helps ensure that the code is designed with testability in mind from the outset.
2. Choosing a Testing Framework: Several excellent unit testing frameworks are available for various programming languages. Popular choices include JUnit (Java), pytest (Python), and NUnit (.NET). These frameworks provide tools for organizing tests, running tests, and reporting results.
3. Defining Test Cases: The heart of effective unit testing lies in creating a comprehensive suite of test cases. This involves:
- Boundary conditions: Test the function with values at the edge of its valid input range. For example, for √x, test with x = 0, and values extremely close to zero. For complex numbers, test with real and imaginary parts approaching zero or infinity.
- Typical values: Test with a wide range of typical input values to ensure the function behaves correctly across the spectrum.
- Edge cases: Test with values that might cause unexpected behavior or errors. For radicals, this includes negative numbers under even-numbered roots. For complex numbers, this could include values with very large magnitudes or those approaching zero.
- Error handling: Test how the function handles invalid or unexpected inputs, such as non-numeric values or inputs outside the defined domain. Expect exceptions or error codes when appropriate.
4. Assertions: Unit testing frameworks provide assertion functions that allow you to check the results of your function against expected values. Common assertion types include:
- assertEqual(actual, expected): Checks if the actual result is equal to the expected result.
- assertNotEqual(actual, expected): Checks if the actual result is not equal to the expected result.
- assertTrue(condition): Checks if a boolean condition is true.
- assertFalse(condition): Checks if a boolean condition is false.
- assertIsNone(value): Checks if a value is None.
- **assertRaises(ExceptionType, function, *args, kwargs): Checks if a specific exception is raised when calling a function.
5. Example Test Cases (Python with pytest):
Let's illustrate with Python examples using the pytest
framework. Suppose we have functions for calculating the square root of a number and adding two complex numbers:
import cmath
import pytest
def sqrt_function(x):
"""Calculates the square root of a number."""
if x < 0:
raise ValueError("Cannot calculate square root of a negative number")
return x**0.5
def add_complex(z1, z2):
"""Adds two complex numbers."""
return z1 + z2
Now, let's write pytest functions to test these functions:
def test_sqrt_positive():
assert sqrt_function(9) == 3.0
assert sqrt_function(0.25) == 0.5
assert sqrt_function(16) == 4.0
def test_sqrt_negative():
with pytest.raises(ValueError):
sqrt_function(-4)
def test_add_complex_numbers():
z1 = complex(2, 3)
z2 = complex(4, -1)
assert add_complex(z1, z2) == complex(6, 2)
assert add_complex(complex(0,0), complex(1,1)) == complex(1,1)
def test_add_complex_zero():
assert add_complex(complex(0,0), complex(0,0)) == complex(0,0)
def test_sqrt_boundary():
assert sqrt_function(0) == 0.0
assert abs(sqrt_function(0.00001) - 0.01) < 0.0001 # accounts for floating point precision
These tests cover positive numbers, negative numbers (checking for the expected ValueError
), complex number addition, addition with zero and boundary conditions near zero for the square root.
6. Comprehensive Test Coverage: Aim for high test coverage. This means striving to write tests that cover all possible execution paths and scenarios within your functions. Tools exist to measure test coverage, providing insights into which parts of your code are thoroughly tested and which areas need more attention.
7. Continuous Integration: Integrate unit tests into your development workflow using continuous integration (CI) systems. CI systems automatically run tests whenever changes are made to the codebase, providing immediate feedback and helping to prevent regressions.
Advanced Testing Techniques for Radicals and Complex Numbers
For more complex scenarios involving radicals and complex numbers, consider these advanced techniques:
1. Property-Based Testing: Property-based testing generates a large number of random inputs to test the function against specific properties or invariants. If the function violates a property for any of the inputs, the test fails, providing valuable insights into potential bugs. Libraries like Hypothesis (Python) provide support for property-based testing.
2. Fuzz Testing: Fuzz testing involves feeding the function with malformed or unexpected inputs to uncover vulnerabilities or crashes. This helps identify edge cases that might not be apparent during traditional unit testing.
3. Numerical Precision and Tolerance: When dealing with floating-point numbers, remember that calculations might not be perfectly accurate due to rounding errors. When comparing floating-point results, use a tolerance value to account for small discrepancies. Instead of assertEqual
, you might use something like:
assert abs(actual - expected) < tolerance
where tolerance
is a small value representing the acceptable error margin.
4. Testing Trigonometric Functions with Complex Numbers: Functions involving trigonometric functions (sine, cosine, tangent, etc.) with complex arguments require careful testing. Consider using known identities and relationships to verify the correctness of the results.
Conclusion
Rigorous unit testing is essential for ensuring the correctness and reliability of software that handles radicals and complex numbers. By adopting a systematic approach, encompassing test-driven development, comprehensive test cases, effective assertions, and advanced testing techniques, developers can build robust and dependable mathematical functions. Remember that continuous integration and monitoring test coverage contribute significantly to maintaining high software quality over time. These strategies not only help prevent bugs but also improve the overall maintainability and scalability of your codebase. The investment in thorough unit testing pays off handsomely in the long run, leading to more stable and reliable applications.
Latest Posts
Latest Posts
-
Test Bank Lewis Medical Surgical 12th Edition
May 25, 2025
-
King Lear Act 1 Scene 2
May 25, 2025
-
The Way Of The World By William Congreve
May 25, 2025
-
About What Are Proctor And Putnam Fighting
May 25, 2025
-
How Does Shakespeare Use The Motif Of Light
May 25, 2025
Related Post
Thank you for visiting our website which covers about 2.09 Unit Test Radicals And Complex Numbers . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.