1.25 Lab Warm Up Variables Input And Type Conversion
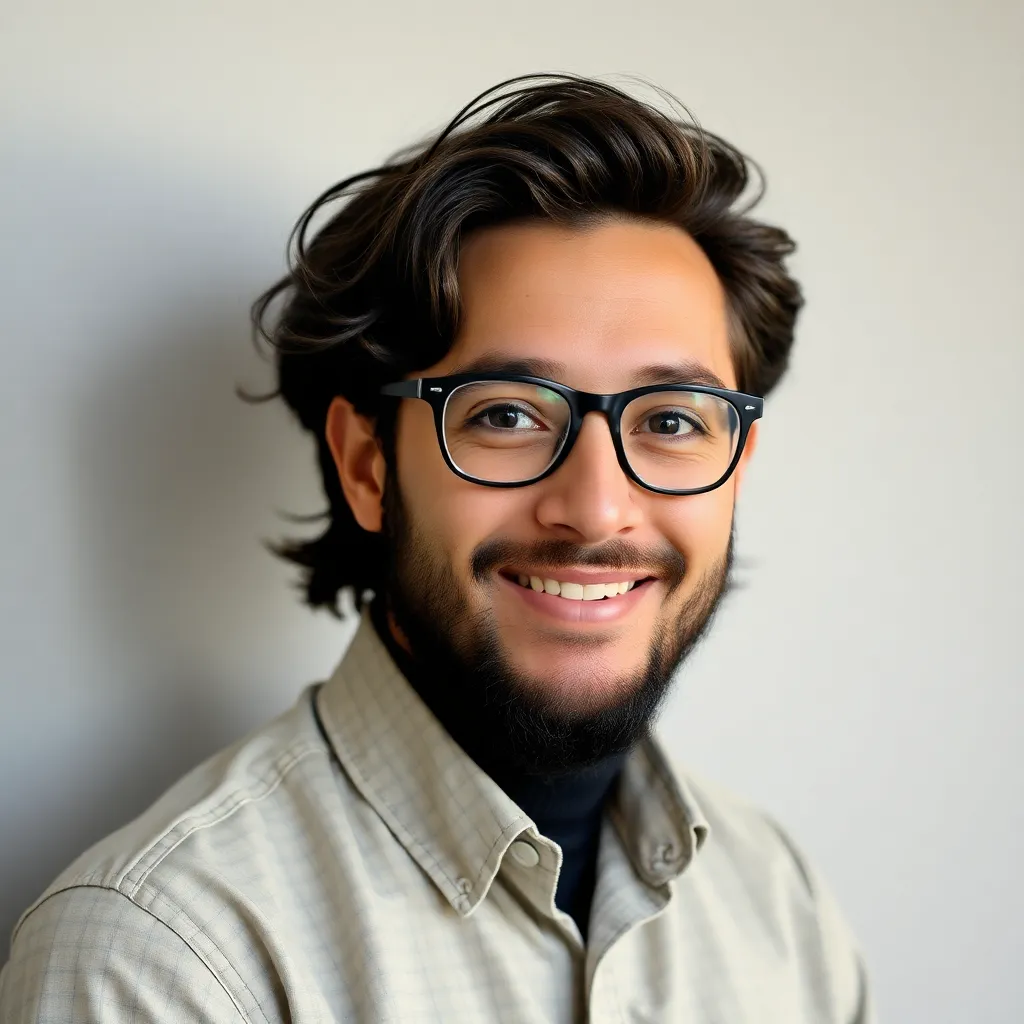
Juapaving
May 25, 2025 · 6 min read
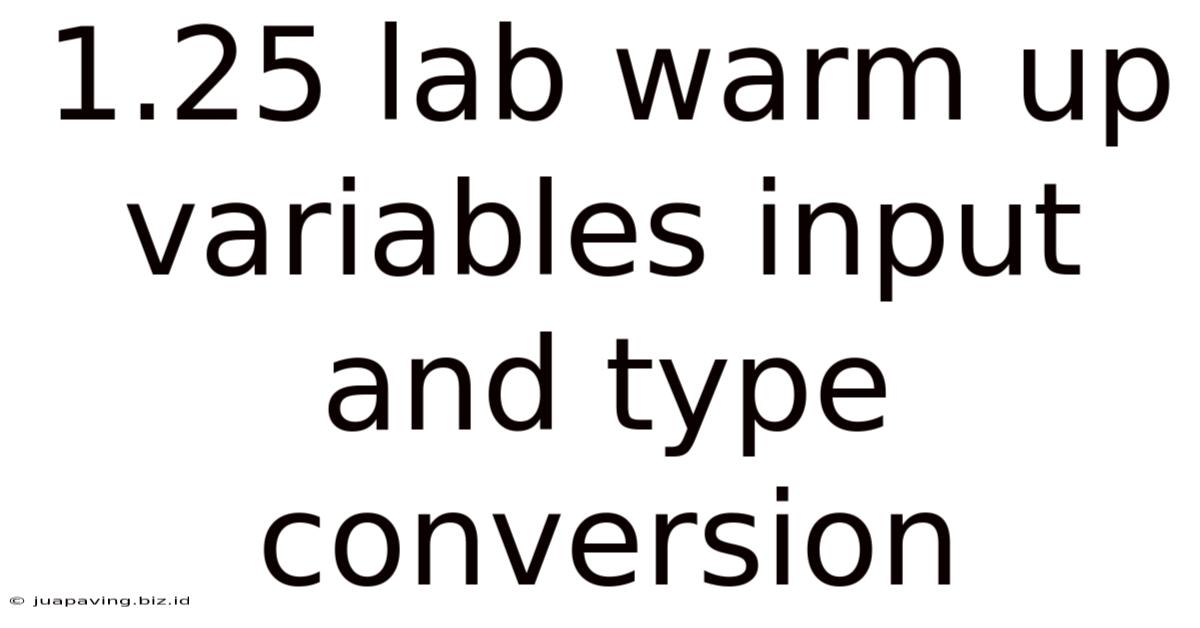
Table of Contents
1.25 Lab Warm-up: Variables, Input, and Type Conversion in Programming
This comprehensive guide delves into the fundamentals of variables, input, and type conversion, crucial elements for any aspiring programmer. We'll explore these concepts with practical examples, focusing on clarity and understanding. This lab-style approach is designed to build a strong foundation for more complex programming tasks. We'll be using Python for our examples, but the underlying principles are applicable to most programming languages.
Understanding Variables
In programming, a variable acts as a named container for storing data. Think of it like a labeled box where you can place information. This information can be anything from numbers and text to more complex data structures. The key features of variables include:
-
Name: A variable is identified by a unique name, following specific naming conventions (e.g., starting with a letter or underscore, using only alphanumeric characters and underscores). Good variable names are descriptive and reflect the data they hold. For instance,
user_age
is better thanx
ora
. -
Data Type: Every variable holds a specific data type, indicating the kind of information it can store. Common data types include:
- Integer (int): Whole numbers (e.g., 10, -5, 0).
- Floating-point number (float): Numbers with decimal points (e.g., 3.14, -2.5, 0.0).
- String (str): Text enclosed in quotes (e.g., "Hello", 'Python').
- Boolean (bool): Represents truth values (True or False).
-
Value: The actual data stored within the variable. This can change during the program's execution.
Declaring and Assigning Variables in Python
In Python, variable declaration is implicit. You don't need to specify the data type explicitly; Python infers it based on the assigned value. Assignment is done using the =
operator.
# Assigning integer value
age = 30
# Assigning floating-point value
price = 99.99
# Assigning string value
name = "Alice"
# Assigning boolean value
is_active = True
Best Practices for Variable Names
Choosing meaningful variable names significantly improves code readability and maintainability. Here are some best practices:
-
Descriptive Names: Use names that clearly indicate the variable's purpose (e.g.,
total_sales
,customer_id
). -
Camel Case or Snake Case: Adopt a consistent naming convention. Camel case (
userName
) capitalizes the first letter of each word except the first, while snake case (user_name
) uses underscores to separate words. -
Avoid Reserved Words: Don't use Python keywords (e.g.,
if
,else
,for
,while
) as variable names.
User Input and the input()
Function
Often, programs need to interact with the user, obtaining data through input. In Python, the input()
function facilitates this. It reads a line of text from the user's console and returns it as a string.
user_name = input("Please enter your name: ")
print("Hello,", user_name + "!")
This code snippet prompts the user to enter their name, stores it in the user_name
variable, and then displays a personalized greeting. Note that even if the user enters a number, input()
treats it as a string.
Type Conversion (Casting)
Since input()
always returns a string, you often need to convert (cast) the input to the appropriate data type for your calculations or operations. Python provides built-in functions for type conversion:
-
int()
: Converts a string or a number to an integer. It will raise aValueError
if the string cannot be converted to an integer. -
float()
: Converts a string or a number to a floating-point number. Similar toint()
, it raises aValueError
if the conversion fails. -
str()
: Converts a number or other data type to a string. -
bool()
: Converts a value to a Boolean. Empty strings, 0, and None are considered False; otherwise, it's True.
Handling Potential Errors with try-except
Blocks
Type conversion can fail if the user enters invalid input (e.g., typing letters when an integer is expected). To gracefully handle these errors, use try-except
blocks:
try:
age = int(input("Enter your age: "))
print("You are", age, "years old.")
except ValueError:
print("Invalid input. Please enter a valid integer.")
This code attempts to convert the user's input to an integer. If a ValueError
occurs (due to invalid input), the except
block executes, displaying an error message. This prevents the program from crashing.
Advanced Type Conversion Scenarios
Let's explore more complex scenarios involving type conversion:
Converting Strings to Numbers with Different Bases
You might encounter strings representing numbers in bases other than 10 (decimal). Python offers functions to handle these:
int(string, base)
: Converts a string representing a number in the specifiedbase
(e.g., 2 for binary, 16 for hexadecimal) to an integer.
binary_number = "101101"
decimal_equivalent = int(binary_number, 2)
print(f"The decimal equivalent of {binary_number} is: {decimal_equivalent}")
hex_number = "1A"
decimal_hex = int(hex_number, 16)
print(f"The decimal equivalent of {hex_number} is: {decimal_hex}")
Working with Floating-Point Numbers and Precision
Floating-point numbers can sometimes lead to unexpected results due to their representation in computer memory. Be mindful of precision issues when performing calculations:
price = 99.99
quantity = 10
total = price * quantity
print(f"Total cost: {total}") # Might display a slightly imprecise result due to floating-point representation
For situations requiring high precision, consider using the decimal
module:
from decimal import Decimal
price = Decimal("99.99")
quantity = Decimal("10")
total = price * quantity
print(f"Total cost (using Decimal): {total}") #More precise result
String Formatting and Type Conversion
When combining different data types in output strings, use f-strings (formatted string literals) or other formatting techniques for clean and readable results:
name = "Bob"
age = 25
print(f"My name is {name} and I am {age} years old.")
#Alternative method using str.format()
print("My name is {} and I am {} years old.".format(name, age))
Practical Applications and Examples
Let's solidify our understanding with some practical examples that integrate variables, input, and type conversion:
1. Simple Calculator:
try:
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
operator = input("Enter the operator (+, -, *, /): ")
if operator == "+":
result = num1 + num2
elif operator == "-":
result = num1 - num2
elif operator == "*":
result = num1 * num2
elif operator == "/":
if num2 == 0:
print("Error: Division by zero")
else:
result = num1 / num2
else:
print("Invalid operator")
print(f"Result: {result}")
except ValueError:
print("Invalid input. Please enter numbers only.")
This program takes two numbers and an operator as input, performs the calculation, and displays the result. It includes error handling for invalid input and division by zero.
2. Unit Converter:
try:
celsius = float(input("Enter temperature in Celsius: "))
fahrenheit = (celsius * 9/5) + 32
print(f"{celsius} degrees Celsius is equal to {fahrenheit} degrees Fahrenheit.")
except ValueError:
print("Invalid input. Please enter a number.")
This program converts Celsius to Fahrenheit, demonstrating the use of type conversion and a simple formula.
3. Area Calculator:
shape = input("Enter shape (rectangle, circle): ").lower()
if shape == "rectangle":
try:
length = float(input("Enter length: "))
width = float(input("Enter width: "))
area = length * width
print(f"Area of rectangle: {area}")
except ValueError:
print("Invalid input for rectangle dimensions.")
elif shape == "circle":
try:
radius = float(input("Enter radius: "))
area = 3.14159 * radius**2
print(f"Area of circle: {area}")
except ValueError:
print("Invalid input for circle radius.")
else:
print("Invalid shape selected.")
This program calculates the area of either a rectangle or a circle based on user input, showcasing conditional logic and error handling.
Conclusion
Mastering variables, input, and type conversion forms the foundation for effective programming. Understanding how to store data, handle user input, and convert between data types is essential for building robust and interactive applications. Remember to always prioritize clear variable names, handle potential errors with try-except
blocks, and choose appropriate type conversion methods to ensure your programs function correctly and efficiently. Practice these concepts with diverse exercises to solidify your understanding and build your programming skills.
Latest Posts
Latest Posts
-
Amigos De Todas Partes Vhl Answers
May 25, 2025
-
A Christmas Carol Stave 5 Summary
May 25, 2025
-
Closed Innovation Is Associated With Which Organizational Inertia Problem
May 25, 2025
-
Which Of The Following Is A Valid Offer
May 25, 2025
-
What Point Of View Is A Rose For Emily
May 25, 2025
Related Post
Thank you for visiting our website which covers about 1.25 Lab Warm Up Variables Input And Type Conversion . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.