1.13 Unit Test Graphs Of Sinusoidal Functions
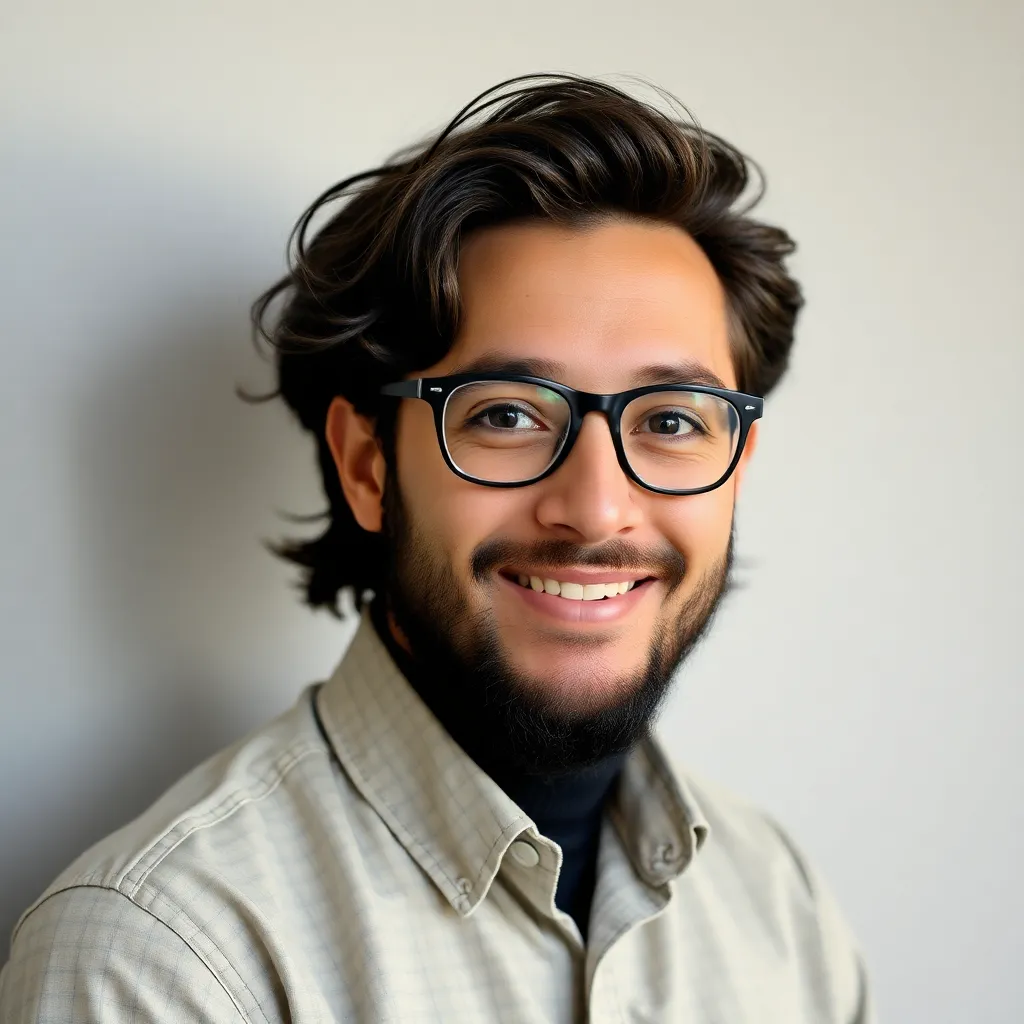
Juapaving
May 24, 2025 · 5 min read
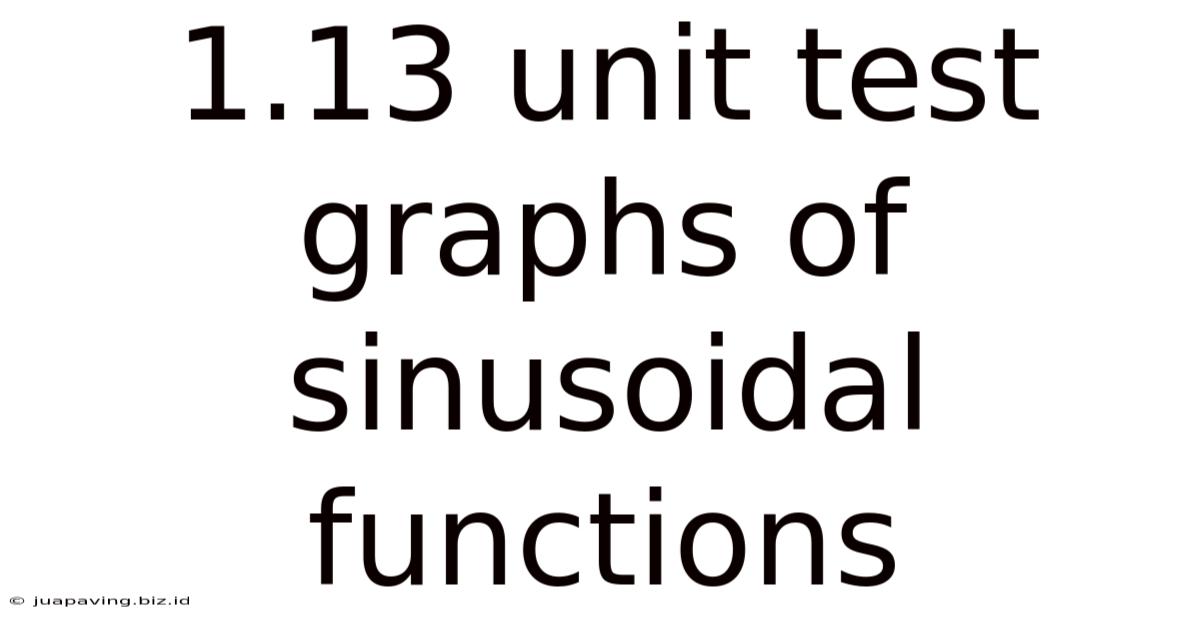
Table of Contents
1.13 Unit Test Graphs of Sinusoidal Functions: A Comprehensive Guide
This article delves into the intricacies of unit testing graphs of sinusoidal functions, a crucial aspect of software development in fields like signal processing, physics simulations, and mathematical modeling. We will explore various testing strategies, emphasizing best practices and providing practical examples to ensure your sinusoidal function implementations are robust and accurate. This guide aims to be comprehensive, covering aspects from fundamental concepts to advanced testing techniques.
Understanding Sinusoidal Functions and their Graphs
Before diving into unit testing, let's solidify our understanding of sinusoidal functions. These functions, including sine and cosine, are characterized by their periodic oscillations. Their graphs are smooth, continuous waves that repeat over a fixed interval known as the period. Key parameters defining a sinusoidal function include:
- Amplitude: The height of the wave from its center line to its peak or trough.
- Period: The horizontal distance after which the wave repeats itself.
- Phase Shift: A horizontal shift of the wave to the left or right.
- Vertical Shift: A vertical shift of the wave upwards or downwards.
A general sinusoidal function can be represented as: y = A * sin(B(x - C)) + D
or y = A * cos(B(x - C)) + D
, where:
A
is the amplitude.B
determines the period (period = 2π/|B|).C
is the phase shift.D
is the vertical shift.
Understanding these parameters is fundamental to effectively testing sinusoidal functions.
The Importance of Unit Testing Sinusoidal Functions
Unit testing plays a vital role in ensuring the correctness and reliability of software involving sinusoidal functions. Inaccurate implementations can lead to significant errors in applications ranging from audio processing to control systems. Rigorous testing helps:
- Identify Bugs Early: Catching errors during the development phase is significantly cheaper and easier than fixing them later.
- Improve Code Quality: Writing testable code inherently leads to better design and modularity.
- Ensure Accuracy: Accurate representation of sinusoidal functions is critical for many applications. Unit tests provide a mechanism to verify this accuracy.
- Facilitate Regression Testing: As the code evolves, unit tests ensure that new features or bug fixes don't inadvertently break existing functionality.
Strategies for Unit Testing Sinusoidal Function Graphs
Effectively testing graphs requires a multifaceted approach. We will focus on three primary strategies:
1. Point-by-Point Verification
This method involves evaluating the function at various points within its period and comparing the calculated values to expected values. This is best suited for smaller, well-defined intervals. For instance, you might test at:
- Key Points: Points corresponding to the peaks, troughs, zero-crossings, and other critical points of the wave.
- Random Points: Selecting random points within the period to ensure consistent accuracy across the entire function.
- Boundary Points: Testing points at the beginning and end of the period to handle potential edge cases.
Example (Python):
import math
import unittest
def sine_wave(x, amplitude, period, phase_shift, vertical_shift):
"""Calculates the y-value of a sine wave at a given x."""
return amplitude * math.sin(2 * math.pi / period * (x - phase_shift)) + vertical_shift
class TestSineWave(unittest.TestCase):
def test_key_points(self):
amplitude = 2
period = 1
phase_shift = 0
vertical_shift = 1
self.assertAlmostEqual(sine_wave(0, amplitude, period, phase_shift, vertical_shift), 1) #Start point
self.assertAlmostEqual(sine_wave(0.25, amplitude, period, phase_shift, vertical_shift), 3) #Peak
self.assertAlmostEqual(sine_wave(0.5, amplitude, period, phase_shift, vertical_shift), 1) #Mid point
self.assertAlmostEqual(sine_wave(0.75, amplitude, period, phase_shift, vertical_shift), -1) #Trough
self.assertAlmostEqual(sine_wave(1, amplitude, period, phase_shift, vertical_shift), 1) #End point
def test_random_points(self):
# Add more assertions with randomly generated points
pass
if __name__ == '__main__':
unittest.main()
2. Comparative Testing with a Reference Implementation
This strategy involves comparing the output of your function to the output of a known, reliable implementation (e.g., a well-tested library function). This approach is particularly useful for verifying overall accuracy across a wider range of inputs. Discrepancies reveal potential errors in your implementation.
3. Visual Inspection and Graphical Comparison
While not a rigorous method on its own, visual inspection offers valuable insights. Plotting your function's graph alongside the expected graph allows for quick identification of gross inaccuracies. Tools like matplotlib (Python) or similar libraries can be used to generate these plots. Discrepancies might be subtle and missed by point-by-point testing.
Example (Python with Matplotlib):
import matplotlib.pyplot as plt
import numpy as np
# ... your sine_wave function ...
x = np.linspace(0, 10, 500) # Generate x-values
y = sine_wave(x, 2, 1, 0, 1) #Calculate y-values with your function
#Plot the generated sine wave
plt.plot(x, y)
plt.xlabel("x")
plt.ylabel("y")
plt.title("Sine Wave Graph")
plt.grid(True)
plt.show()
Advanced Testing Techniques
- Parameterization: Use parameterized tests to efficiently test the function with various combinations of amplitude, period, phase shift, and vertical shift. This minimizes code duplication.
- Edge Case Testing: Thoroughly test boundary conditions, such as extremely large or small values of the input parameters, zero values, and values near singularities.
- Performance Testing: For computationally intensive functions, measure the execution time to ensure that your implementation is efficient.
- Integration Testing: Integrate your sinusoidal function into a larger system and test its interaction with other components.
Best Practices for Unit Testing Sinusoidal Functions
- Use a Testing Framework: Employ a dedicated testing framework like unittest (Python), JUnit (Java), or pytest (Python) for organized and effective testing.
- Write Clear and Concise Tests: Use descriptive test names that clearly communicate the purpose of each test.
- Aim for High Test Coverage: Strive for a high percentage of code coverage to ensure that all parts of the function are tested.
- Regularly Update Tests: Keep your tests up to date as your code evolves to prevent regressions.
- Use Assertions Effectively: Employ appropriate assertion methods to check for expected values, ranges, or types.
Conclusion
Thorough unit testing of sinusoidal function graphs is paramount for creating reliable and accurate software. The combination of point-by-point verification, comparative testing, visual inspection, and advanced techniques outlined above provides a robust framework for ensuring the quality of your implementations. Remember to prioritize code clarity, comprehensive test coverage, and regular testing updates to maintain high software quality and prevent errors. By adhering to these guidelines, you can build robust and accurate applications that rely on the precise representation of sinusoidal functions.
Latest Posts
Latest Posts
-
The Following Musical Excerpt Is From A Recitative
May 25, 2025
-
A Lesson Before Dying Chapter Summary
May 25, 2025
-
Which Term Best Describes Observable Movement Of The Limbs
May 25, 2025
-
Walmarts Implementation Of Rfid A Type Of Technology
May 25, 2025
-
Equilibrium And Le Chateliers Principle Lab
May 25, 2025
Related Post
Thank you for visiting our website which covers about 1.13 Unit Test Graphs Of Sinusoidal Functions . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.